How to Extract URL Parameters with JavaScript
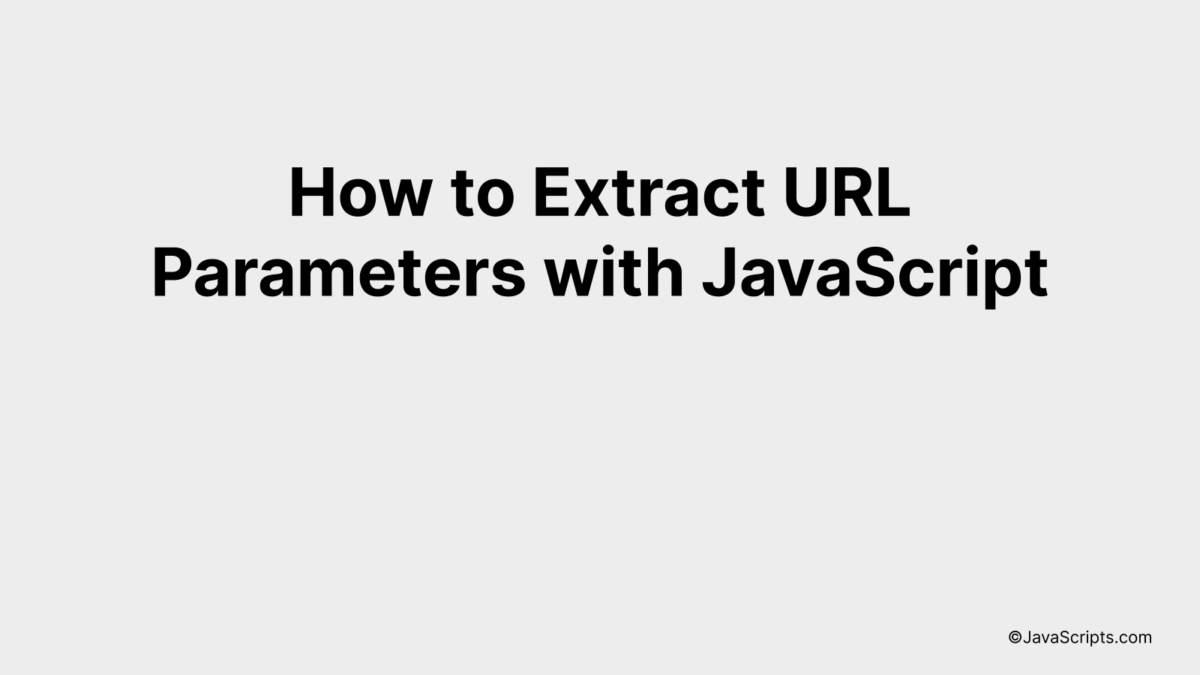
Navigating the world of web development can feel like deciphering a complex puzzle. Have you ever wondered how to retrieve specific bits of information from a URL? JavaScript, with its wide array of capabilities, holds the answer to this question.
We’re here to unravel the mystery behind extracting URL parameters using JavaScript. Picture yourself dealing with an intricate web of data. Now imagine a tool that can swiftly sift through this data to find what you need. That’s the power we’re about to harness together.
In this post, we will be looking at the following 3 ways to extract URL parameters with JavaScript:
- By using the URLSearchParams() interface
- By using the split() and slice() methods
- By using the RegExp and match() method
Let’s explore each…
#1 – By using the URLSearchParams() interface
The URLSearchParams() interface provides utility methods to work with the query string of a URL. It helps us to easily parse, read, append, delete, or modify query string parameters. Here’s an example of how you can extract URL parameters using this interface in JavaScript.
var url = new URL('https://example.com?product=shirt&color=blue&size=m');
var params = new URLSearchParams(url.search);
var product = params.get('product');
var color = params.get('color');
var size = params.get('size');
console.log(product); // Output: "shirt"
console.log(color); // Output: "blue"
console.log(size); // Output: "m"
How it works
The URLSearchParams() interface in JavaScript is used to work with the query string of a URL. The example provided demonstrates how to extract URL parameters using this interface.
- The first line of the code creates a new URL object using the URL API. The URL used for this example is ‘https://example.com?product=shirt&color=blue&size=m’.
- Next, we create a new URLSearchParams object, passing in the search property of our URL object. This provides us with an object that makes it easy to read or manipulate the parameters of the URL.
- The ‘get’ method of the URLSearchParams object is then used to retrieve the values of specific parameters. In this case, we’re retrieving the values for ‘product’, ‘color’, and ‘size’.
- Finally, we log these values to the console to display the results. The ‘get’ method will return the value of the specified URL parameter, if it exists.
In this way, URL parameters can be easily extracted using the URLSearchParams interface in JavaScript.
#2 – By using the split() and slice() methods
This JavaScript approach will work by first extracting the entire URL after the “?” symbol using the slice() method and then splitting the parameters by the “&” symbol with the split() method. To understand better, let’s consider an example URL like “www.example.com?param1=value1¶m2=value2”.
var url_params = window.location.search.slice(1).split('&');
How it works
This method extracts URL parameters by taking the part of the URL after the “?” symbol and splitting it into an array, where each element is a distinct parameter.
- First, the
window.location.search
property is used to get the part of the URL that follows the “?” symbol, which includes the “?” symbol. This gives us “?param1=value1¶m2=value2”. - The
slice(1)
method is then used to remove the “?” symbol from the start, resulting in “param1=value1¶m2=value2”. - Finally, the
split('&')
method is used to divide the string into an array of substrings at each “&” symbol. The result is an array: [“param1=value1”, “param2=value2”].
#3 – By using the RegExp and match() method
We will extract URL parameters using JavaScript by creating a regular expression (RegExp) that matches URL parameters, and then applying this RegExp to the URL using the match() method. This will return an array of matches that we can then process further. For example, if our URL is “http://example.com/?param1=test¶m2=test2”, we can extract the parameters “param1” and “param2” along with their values.
let url = "http://example.com/?param1=test¶m2=test2";
let params = url.match(/(?|&)([^=]+)=([^&]+)/g);
How it works
The RegExp in the match() method targets URL parameters and their values. It finds each parameter by looking for a “?” or “&”, followed by any number of characters that aren’t “=”, followed by an “=”, followed by any number of characters that aren’t “&”. This returns an array of matches (URL parameters and their values) that we can further process.
- The first character in the RegExp, “(?|&)”, is a group that matches either a “?” or “&”. This is because URL parameters are always preceded by one of these two characters.
- The next part, “([^=]+)”, is a group that matches one or more of any character that is not “=”. This represents the parameter name.
- The “=” character in the RegExp is a literal match. It separates the parameter name from its value in the URL.
- The final group, “([^&]+)”, matches one or more of any character that is not “&”. This represents the parameter value. The value of a parameter ends when it encounters an “&” (which starts the next parameter), or when it reaches the end of the string.
Related:
- How to Compare Values with Less Than or Equal to in JavaScript
- How to Check if a String is Empty in JavaScript
- How to Check if a String is a Number in JavaScript
In conclusion, extracting URL parameters with JavaScript is a simple but valuable skill for web developers. It can help in dynamically modifying web content, tracking user behavior, and troubleshooting issues. By understanding and implementing this feature, you’ll be able to create a more flexible and interactive website.
Remember, practice is key when mastering new technical abilities. Don’t hesitate to experiment with URL parameters and JavaScript. The more you work with it, the more you’ll uncover its potential. Happy coding!