How to Perform Case-Insensitive Includes in JavaScript
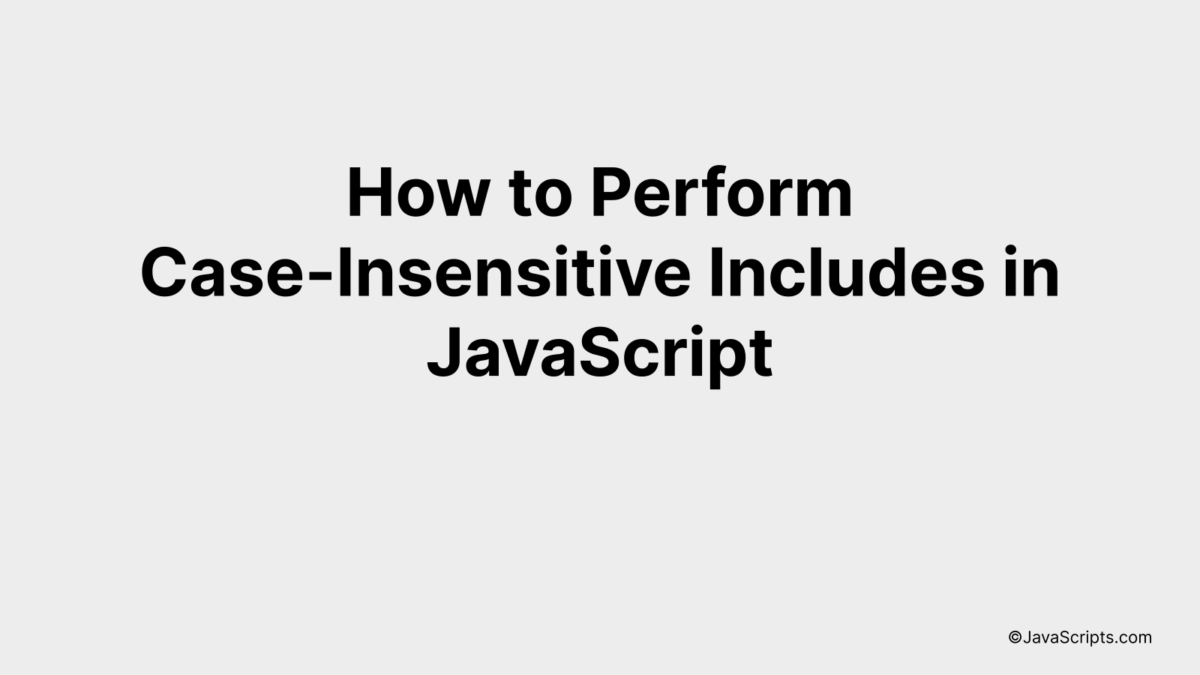
As JavaScript developers, we often encounter situations where we need to check if a string contains another string, without concerning ourselves with case sensitivity. Can you recall how many times you’ve found yourself troubleshooting an error, only to discover it was a simple case mismatch?
Don’t worry, there’s a solution to this common problem! Let’s explore a straightforward method for performing case-insensitive includes in JavaScript. This technique allows you to compare or search strings without worrying about whether they’re in upper case, lower case, or a mixture of both.
In this post, we will be looking at the following 3 ways to perform case-insensitive includes in JavaScript:
- By using the toLowerCase() method
- By using the toUpperCase() method
- By using the RegExp constructor with the ‘i’ flag
Let’s explore each…
#1 – By using the toLowerCase() method
This process involves converting both the main string and the target string to the same case (lowercase) by using the toLowerCase() JavaScript method, then checking if the main string contains the target string with the includes() method. See the example below for better understanding.
let mainStr = "Hello World";
let targetStr = "hello";
let isIncludes = mainStr.toLowerCase().includes(targetStr.toLowerCase());
console.log(isIncludes);
How it works
This code works by converting all the letters in both the main string and the target string to lowercase, thereby removing any differences due to case. The includes() method then checks if the target string exists within the main string.
- First, the toLowerCase() method is called on mainStr which converts all the characters in mainStr to lowercase.
- Next, the toLowerCase() method is called on targetStr which converts all the characters in targetStr to lowercase.
- The includes() method is then called on the lowercase version of mainStr and it checks if this string includes the lowercase version of targetStr.
- If the lowercase version of targetStr does exist within the lowercase version of mainStr, the includes() method returns true – indicating that mainStr does include targetStr, ignoring case. If it doesn’t, it returns false.
- The result is stored in the variable isIncludes and then logged to the console.
This way, we can perform a case-insensitive includes check in JavaScript.
#2 – By using the toUpperCase() method
The process involves transforming both the input string and the target string to the same case (upper or lower) using the toUpperCase() method, then checking if the target string exists within the input string using the includes() method. This ensures a case-insensitive check.
let inputString = "Hello, World!";
let targetString = "WORLD";
if(inputString.toUpperCase().includes(targetString.toUpperCase())){
console.log("Target string exists in the input string.");
} else {
console.log("Target string does not exist in the input string.");
}
How it works
The above JavaScript code converts both the input string and target string to uppercase and then checks if the target string exists in the input string. This is a case-insensitive technique, as it does not consider the case (upper or lower) of the original strings.
- Step 1: Define the input string and the target string. The input string is “Hello, World!”, and the target string is “WORLD”.
- Step 2: Use the toUpperCase() method to convert both the input string and the target string to uppercase.
- Step 3: Use the includes() method to check if the converted target string exists in the converted input string.
- Step 4: If the target string exists in the input string, it will print “Target string exists in the input string.” If not, it will print “Target string does not exist in the input string.”
#3 – By using the RegExp constructor with the ‘i’ flag
In JavaScript, to perform a case-insensitive includes operation, we can leverage the RegExp constructor with the ‘i’ flag. Here, the ‘i’ flag indicates the operation is case-insensitive. For instance, let’s say we want to find the word ‘test’ in a string regardless of its case.
var str = "I am a TeSt string";
var keyword = "test";
var pattern = new RegExp(keyword, 'i');
var result = pattern.test(str);
How it works
In the given JavaScript code, we are creating a new instance of RegExp using the keyword we want to match, and specifying the ‘i’ flag for case-insensitive matching. The test() method of the RegExp object then checks if our input string contains the keyword and returns a boolean value.
- First, we declare a variable ‘str’ that holds the string in which we want to find the keyword.
- Then, we declare a variable ‘keyword’ that contains the word we want to match in the string.
- Next, we create an instance of RegExp with the keyword and the ‘i’ flag for case-insensitivity. This gives us a pattern to match against.
- Finally, using the test() method of the RegExp object, we check if our string ‘str’ contains the keyword. This method will return true if it finds a match; otherwise, it will return false.
Related:
- How to Get Unique Values from an Array in JavaScript
- How to Determine the Size of a Map in JavaScript
- How to Determine the Length of a Dictionary in JavaScript
To sum up, performing case-insensitive includes in JavaScript is simple yet important. Proper use of the ‘toLowerCase’ function can help you achieve the goal without any complications.
Remember, every small code optimization can lead to a more efficient and user-friendly application. So, always keep an eye out for these small but significant improvements.