How to Remove Substrings from Strings in JavaScript
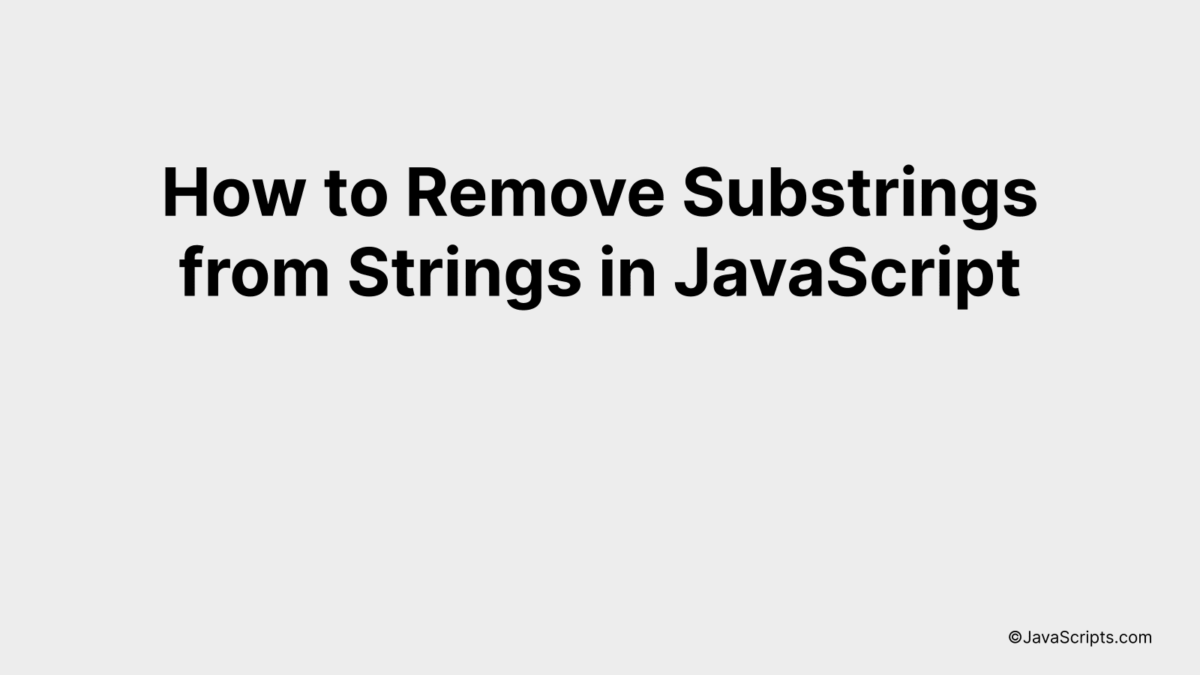
Unraveling the intricacies of JavaScript, we often stumble upon certain tasks which might seem complex at first, but are straightforward once understood. One such task is the removal of substrings from strings, a frequently encountered operation whether you’re manipulating data or creating interactive websites.
Let’s dive into the world of JavaScript together, exploring easy methods to remove substrings from strings efficiently. Throughout this journey, you’ll be equipped with practical examples and clear explanations, making every step of the process simpler and more engaging.
In this post, we will be looking at the following 3 ways to remove substrings from strings in JavaScript:
- By using the replace() method
- By using the slice() method
- By using the substring() method
Let’s explore each…
#1 – By using the replace() method
The JavaScript replace()
method is used to replace a specified value or substring in a string with another value or substring. It creates a new string without altering the original one. To illustrate this, let’s consider an example where we want to remove the substring “World” from the string “Hello World”.
var str = "Hello World";
var newStr = str.replace("World", "");
How it works
The replace()
method scans the original string for the first match of the substring specified to be replaced (in this case “World”). When the method finds the substring, it replaces it with the new value specified (in this case, an empty string “”).
- The variable
str
is initialized with the string “Hello World”. - The
replace()
method is called onstr
with “World” as the value to be replaced and “” (empty string) as the replacement. - Since “World” exists in
str
, it is replaced with an empty string, effectively removing “World” from the string. - The new string is then stored in the variable
newStr
. - As a result,
newStr
will contain “Hello ” (with a trailing space) as it has removed “World”.
#2 – By using the slice() method
The JavaScript slice()
method will be used to remove a substring from a string by identifying its start and end index. This technique will be demonstrated through an example.
var str = "Hello, World!";
var newStr = str.slice(0, 5) + str.slice(7);
How it works
The slice()
method in JavaScript is used to extract a section of a string and returns it as a new string without modifying the original string. In this case, it is used to remove a substring by concatenating two sliced parts of the original string, effectively excluding the undesired substring.
- The
slice()
method takes two parameters: the start index and the end index. The start index is inclusive, whereas the end index is exclusive. - In the provided code, two slices are made: one from index 0 to 5 (extracting “Hello”) and another from index 7 to the end of the string (extracting “World!”).
- Notice that the substring from index 5 to 7, i.e., “, ” is left out. This effectively removes that substring from the original string.
- The ‘+’ operator is then used to concatenate the two resulting strings, forming “HelloWorld!”
- The original string remains unchanged, and we have a new string without the undesired substring.
#3 – By using the substring() method
The substring() method in JavaScript can be used to extract a part of a string, but it doesn’t remove substrings. To remove a substring, we can use the replace() method. The replace() method searches for a specific substring in a string and replaces it with another substring. Here’s how it works.
var originalString = "Hello World!";
var substringToRemove = " World";
var newString = originalString.replace(substringToRemove, "");
console.log(newString); // Outputs: "Hello!"
How it works
The JavaScript replace() method is used to replace a substring in a string with a new substring. It takes two parameters: the substring to find and the substring to replace it with. When the specified substring is found, it is replaced by the new substring and a new string is returned. The original string does not change.
- Step 1: We define the original string: “Hello World!”.
- Step 2: We define the substring we want to remove: ” World”.
- Step 3: We use the replace() method on the original string. We pass the substring to remove as the first parameter and an empty string as the second parameter. This replaces the substring with nothing, effectively removing it.
- Step 4: The replace() method returns a new string with the specified substring removed. We store this new string in a variable.
- Step 5: We print the new string to the console. The output is: “Hello!”.
Related:
- How to Perform Case-Insensitive Includes in JavaScript
- How to Get Unique Values from an Array in JavaScript
- How to Determine the Size of a Map in JavaScript
In conclusion, removing substrings from strings in JavaScript can be easily accomplished using methods like replace() and slice(). These built-in methods offer straightforward and efficient solutions to modify strings according to your needs.
Remember, understanding and mastering these techniques not only helps in data manipulation but also enhances your overall coding skillset. Keep practicing to perfect your JavaScript string handling skills.