How to Replace DIV Content with JavaScript
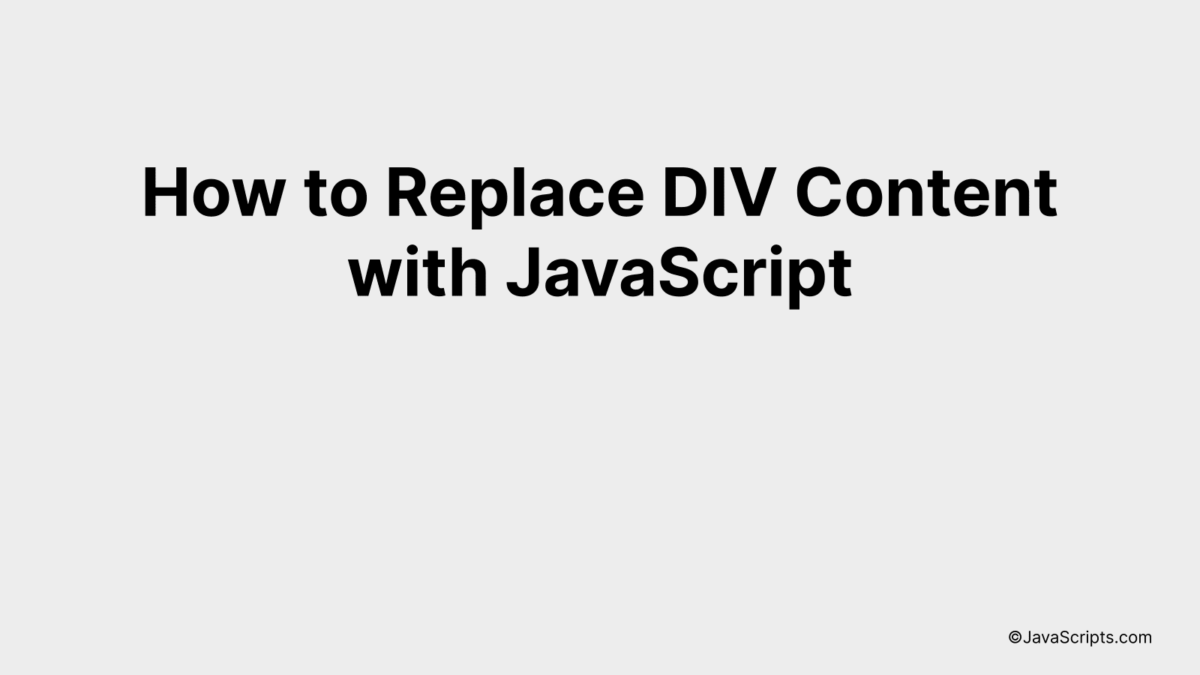
Mastering JavaScript can truly open a world of dynamic possibilities for your web applications. It’s a versatile tool, capable of animating site features, validating input data, and even swapping out content on the fly! One such application involves replacing DIV content, which can greatly enhance the interactivity and user experience of your site.
Now, you might be wondering, “How do I replace DIV content with JavaScript?” Don’t fret, we’re here to guide you through this process. Whether you’re a seasoned developer or a beginner just dipping your toes into the JavaScript pool, we’re confident you’ll find our guide easy to understand and apply.
In this post, we will be looking at the following 3 ways to replace DIV content with JavaScript:
- By using the innerHTML property
- By using the textContent property
- By using the createElement and appendChild methods
Let’s explore each…
#1 – By using the innerHTML property
The JavaScript innerHTML property allows you to get or replace the content of HTML elements. This function can be used to dynamically change the content inside a ‘div’ using a simple line of code. Let’s see this in action with an example.
document.getElementById('myDiv').innerHTML = "New DIV content";
How it works
The JavaScript innerHTML property is used to change the content of the HTML element with id ‘myDiv’. It fetches the element and replaces its content with the new text string ‘New DIV content’.
- First, ‘document.getElementById(‘myDiv’)’ is used to select the HTML element with the id ‘myDiv’.
- Then, the ‘.innerHTML’ property is accessed on this selected element. This property represents the markup of the element’s content.
- The equals sign (‘=’) is used to assign new content to this property. In this case, the new content is the string “New DIV content”.
- As a result, the content inside the ‘div’ with id ‘myDiv’ is replaced with “New DIV content”.
#2 – By using the textContent property
The textContent property in JavaScript allows us to replace the text contained within a certain HTML DIV element. We will identify our target DIV using its ID and then replace the text using this property. Below is a code sample to help you understand better.
document.getElementById("myDiv").textContent = "New text!";
How it works
The provided JavaScript code targets an HTML DIV element with an ID of “myDiv” and changes its text content to “New text!”. This is done using the textContent property, which represents the text contained within a node and all its descendants.
- Step 1: The JavaScript function
document.getElementById("myDiv")
is used to target the DIV element with the ID of “myDiv”. - Step 2: The
.textContent
property is then used to get the text contained in this DIV element as a string. - Step 3: This property is not just read-only; we can also set its value. By setting the value of
.textContent
to “New text!”, we are replacing the existing text in the DIV element with this new string. - Step 4: As a result, the new text is displayed on the webpage in place of the original content of the targeted DIV element.
#3 – By using the createElement and appendChild methods
This procedure involves creating a new HTML element using the createElement
method, populating it with content, and then replacing an existing Div element with this new element using the appendChild
method. Let’s better understand this by using an example.
var oldDiv = document.getElementById("oldDiv"); // Div to be replaced
var newDiv = document.createElement("div"); // Create new Div
newDiv.innerHTML = "New content for the div"; // Add content to the new Div
oldDiv.parentNode.replaceChild(newDiv, oldDiv); // Replace old Div with new Div
How it works
The script first identifies the old div that needs to be replaced. It then creates a new div and inserts content into it. Finally, it replaces the old div with the newly created div in the DOM.
- Step 1: Using the
getElementById
method, we select the div that we want to replace and store it in theoldDiv
variable. - Step 2: Using the
createElement
method, we create a new div and store it in thenewDiv
variable. - Step 3: We populate the newly created div with content by setting the
innerHTML
property of thenewDiv
variable. - Step 4: Using the
replaceChild
method, we replace the old div with the new div. In this method, the new node is passed as the first argument and the node to be replaced as the second argument. This method is called on the parent node, which we access with theparentNode
property of theoldDiv
element.
Related:
- How to Remove Substrings from Strings in JavaScript
- How to Perform Case-Insensitive Includes in JavaScript
- How to Get Unique Values from an Array in JavaScript
In conclusion, replacing DIV content with JavaScript is a simple yet powerful tool to enhance website interactivity. A clear understanding of the Document Object Model (DOM) is the key to effective manipulation of webpage elements.
You can change any HTML element’s content, including DIVs, by using JavaScript’s innerHTML property. Remember that practice is the cornerstone for mastering this skill, so keep experimenting with JavaScript on your webpages.