How to Check if a String Contains a Substring in JavaScript
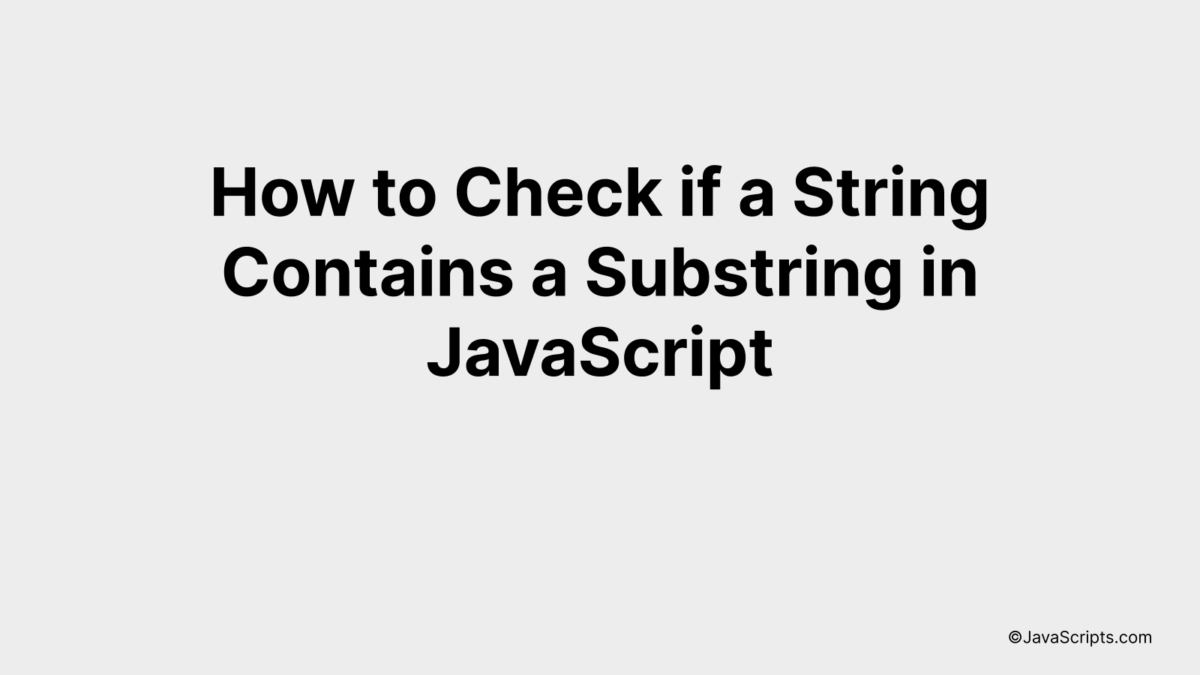
Have you ever found yourself in a situation where you need to check if a specific word or character sequence is present in a longer text string while coding in JavaScript? You’re not alone! This is a pretty common scenario faced by many JavaScript developers.
Don’t worry, though. JavaScript provides straightforward methods to accomplish this task. This process, known as substring checking, can be carried out with ease and proficiency using various JavaScript techniques that we’ll explore together.
In this post, we will be looking at the following 3 ways to check if a string contains a substring in JavaScript:
- By using the indexOf() method
- By using the includes() method
- By using the search() method
Let’s explore each…
#1 – By using the indexOf() method
The JavaScript indexOf() method is used to determine whether a specified string contains a substring. This method returns the index of the first occurrence of the specified value, or -1 if the value is not found. Here’s an example:
var string = "Hello, World!";
var substring = "World";
if(string.indexOf(substring) !== -1) {
console.log(substring + " found!");
} else {
console.log(substring + " not found!");
}
How it works
The indexOf() method compares the specified substring with the strings in the original string. It starts from the beginning of the original string and checks each character until it finds a match or reaches the end of the string.
- Firstly, we define a string variable “string” and a substring “substring”.
- Then, we use the indexOf() method on the “string” variable, passing the “substring” as an argument.
- If the substring is found in the string, indexOf() will return the index (starting from 0) of the first occurrence of the substring.
- If the substring is not found, indexOf() will return -1.
- We then use an if-else statement to check the result of the indexOf() method. If the result is not -1, it means the substring is found in the string. Otherwise, the substring is not found.
- Finally, we print the result to the console.
#2 – By using the includes() method
The includes() method in JavaScript will be used to check if a specific string (substring) is present within another string. This method returns a boolean value (true if the substring is found, false if not). Let’s understand this better with an example.
let str = "Hello, welcome to OpenAI!";
let substring = "OpenAI";
if (str.includes(substring)) {
console.log("Substring found!");
} else {
console.log("Substring not found!");
}
How it works
The includes() method in JavaScript checks if a string (the ‘str’ variable in our example) contains a specific substring (the ‘substring’ variable). It returns ‘true’ if the substring is found and ‘false’ if it’s not. We then use an ‘if’ statement to print a message to the console depending on the result.
- The variable ‘str’ is initialized with the string “Hello, welcome to OpenAI!”.
- The variable ‘substring’ is initialized with the string “OpenAI”.
- The includes() method is called on ‘str’ with ‘substring’ as the argument. This checks whether ‘str’ contains ‘substring’.
- If ‘str’ does include ‘substring’, the method returns ‘true’. We then enter the ‘if’ branch and “Substring found!” is printed to the console.
- If ‘str’ does not include ‘substring’, the method returns ‘false’. We then enter the ‘else’ branch and “Substring not found!” is printed to the console.
#3 – By using the search() method
The JavaScript search()
method can be used to check if a string contains a specific substring. This method returns the index of the first occurrence of the specified substring or -1 if no match is found. Let’s see how this can be applied in practice.
var str = "Hello, welcome to the world of JavaScript!";
var n = str.search("JavaScript");
How it works
The search()
method is executed on the string stored in the variable str
. It checks for the presence of the substring “JavaScript”. If found, it returns the starting position of the substring in str
; if not, it returns -1.
- First, a variable
str
is declared and initialized with the string “Hello, welcome to the world of JavaScript!”. - Next, the
search()
method is invoked on this string with the argument “JavaScript”. This is the substring we are checking for in the main string. - The
search()
method then scans throughstr
, checking for the presence of the substring “JavaScript”. - If the substring is found, the method returns the index of the first occurrence of the substring, which is the position of the first character of the substring in the main string. This value is assigned to the variable
n
. - If the substring is not found, the method returns -1, indicating a lack of match.
Related:
- How to Change Image Source in JavaScript
- How to Access -1 Index in JavaScript Arrays
- How to Use Shorthand If-Else Statements in JavaScript
To sum up, checking if a string contains a substring in JavaScript is a straightforward task. By utilizing methods like indexOf(), includes(), or even match(), we can effectively achieve this.
Always remember, JavaScript is a versatile language. Practicing these methods will undoubtedly help you become more adept at handling different scenarios involving strings.