How to Parse Strings in JavaScript
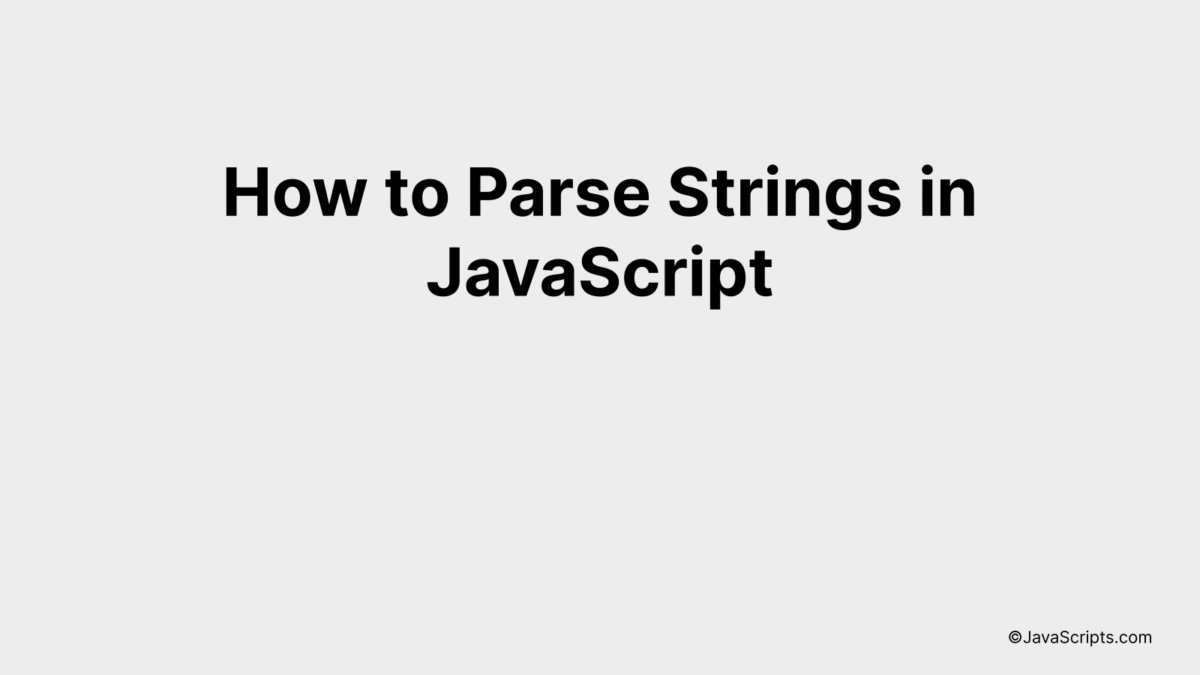
Understanding how to parse strings in JavaScript can open the door to a myriad of possibilities for your coding projects. It’s a crucial skill, allowing you to extract and manipulate data effectively. Don’t worry if you’re completely new to this; we’ll be breaking down everything in the simplest terms possible.
You and I will embark on a journey to master the art of parsing strings. We’ll delve into various methods JavaScript offers, understand their applications, and see them in action through practical examples. Rest assured, by the end, you’ll have this essential skill under your belt.
In this post, we will be looking at the following 3 ways to parse strings in JavaScript:
- By using String.split() method
- By using Regular Expressions with RegExp.exec() method
- By using String.indexOf() and String.substring() methods
Let’s explore each…
#1 – By using String.split() method
The String.split()
method in JavaScript is used to split a string into an array of substrings based on a specified separator, and returns the new array. For instance, if you have a string of “apple,banana,grape” and you want to separate each fruit into an array, you can do this by using the String.split(",")
method.
let fruits = "apple,banana,grape";
let fruitsArray = fruits.split(",");
console.log(fruitsArray); // ["apple", "banana", "grape"]
How it works
The String.split()
method divides a string into an ordered set of substrings, puts these substrings into an array, and returns the array. The division is done by searching for a pattern (specified separator) and separating the string whenever the pattern is found. If separator is an empty string, str is converted to an array of characters.
- The
split()
method is called on the string you want to divide. - The character or characters you want to use as the split point are passed as an argument to the method.
- The method then goes through the string and every time it finds the character or characters it splits the string at that point.
- It returns the sections of the string as elements in an array.
- In our case, the string “apple,banana,grape” is split at each comma, resulting in the array [“apple”, “banana”, “grape”].
#2 – By using Regular Expressions with RegExp.exec() method
The RegExp.exec() method in JavaScript is a powerful tool that can be used to match a string against a regular expression and return an array of results. A better understanding of its working can be attained through a practical example.
var myRegEx = /(d+)/g;
var myString = "I have 100 apples and 200 oranges";
var result = myRegEx.exec(myString);
console.log(result);
How it works
The RegExp.exec() method executes a search for a match in a specified string and returns an array of information or null on a mismatch.
- The regular expression
/(d+)/g
is created, where d matches any digit (equivalent to [0-9]), + causes the engine to match the preceding character 1 or more times, and g is for global search (doesn’t return after the first match). - A string, ‘I have 100 apples and 200 oranges’, is defined to be searched against the regular expression.
- The exec() method is used on the regular expression with the string as an argument, which returns an array of results.
- The array contains the matched strings along with their index positions and input strings. In this case, it contains ‘100’ from the position 7 and ‘200’ from the position 22.
#3 – By using String.indexOf() and String.substring() methods
We are going to use JavaScript’s String.indexOf() and String.substring() methods to locate a specific substring within a larger string and then extract it. For a better understanding, consider an example where we have a string “Hello, World!” and we want to extract “World” from it.
var str = "Hello, World!";
var index = str.indexOf("World");
var substring = str.substring(index, index + "World".length);
How it works
In the provided JavaScript code sample, we first locate the starting position of the target substring “World” in the source string “Hello, World!” using the indexOf() method. We then extract the substring starting from this position to the end of the target substring using the substring() method.
- Firstly, we define a string
str = "Hello, World!"
. - Then we use the
indexOf()
method on the stringstr
to find the index at which the substring"World"
begins. This will return the value 7, which is the position of"W"
in the stringstr
. - We store this index in the variable
index
. - Next, we use the
substring()
method on the stringstr
to extract the substring that starts at the index found in the previous step and ends at the index representing the end of the substring we want to extract. This end index is calculated asindex + "World".length
. - The string
substring
now holds the value “World”.
Related:
- How to Generate GUIDs in JavaScript
- How to Exit Functions in JavaScript
- How to Escape Quotes in JavaScript
In conclusion, parsing strings in JavaScript is a fundamental skill that can make your coding tasks more efficient and your programs more versatile. It enables you to manipulate and extract value from raw data, making it more meaningful and usable.
A good grasp of string parsing can transform your JavaScript programming journey. So, continue experimenting with different methods and scenarios to get better at it. Always remember, practice makes perfect!