How to Escape Quotes in JavaScript
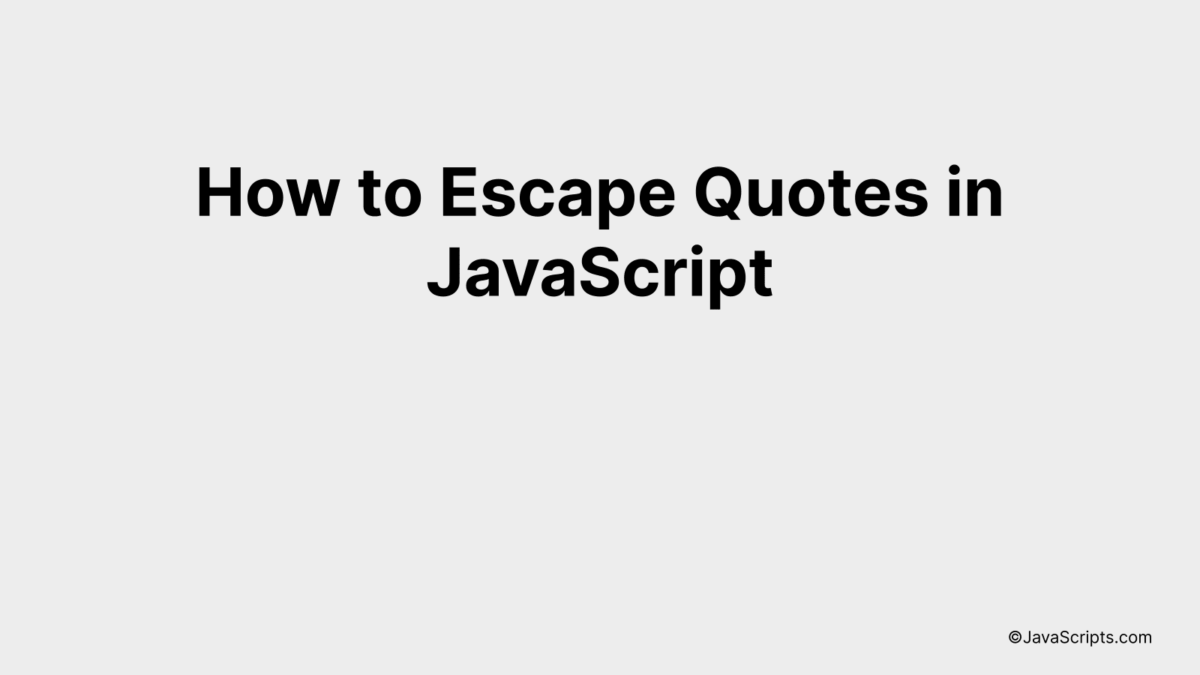
Escaping quotes in JavaScript is something you and I might overlook, yet it’s incredibly crucial. But don’t you worry! You’re not alone in this – many of us have found ourselves puzzled by this seemingly small detail.
Whether you’re an absolute beginner or a seasoned developer, understanding the ins and outs of this topic can save you from unnecessary coding headaches. Together, let’s dive into the world of JavaScript and unravel the mystery behind escaping quotes.
In this post, we will be looking at the following 3 ways to escape quotes in JavaScript:
- By using backslashes
- By using template literals ()
- By using escape() method
Let’s explore each…
#1 – By using backslashes
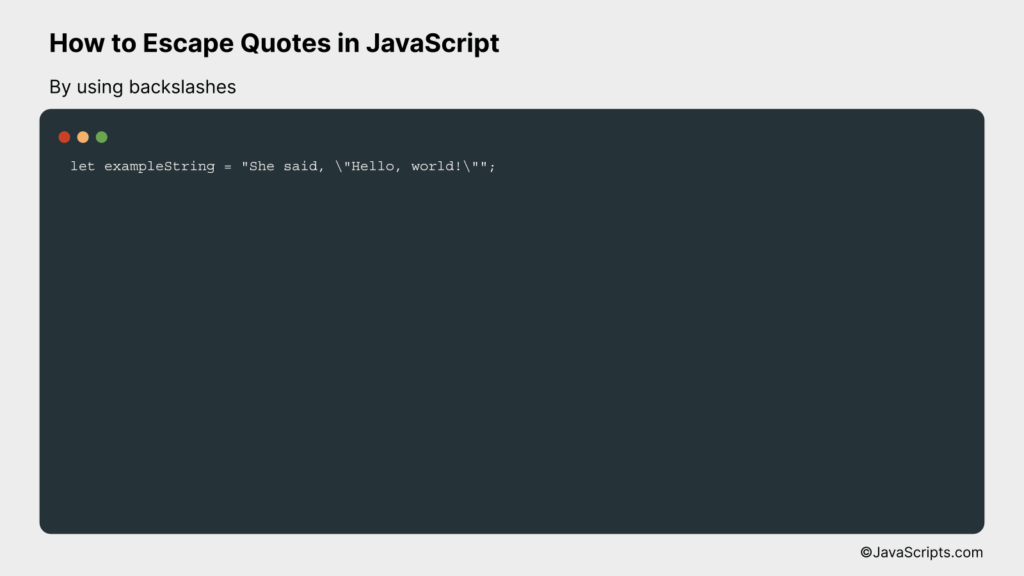
In JavaScript, you can escape quotes by using a backslash before the quote that you want to escape. Here’s an example where we escape double quotes inside a string that is enclosed with double quotes.
let exampleString = ""She said, \""Hello, world!\"""";
How it works
The backslash (\) in JavaScript is an escape character that changes the special character following it into a string character. It allows you to use reserved characters as string.
- The variable ‘exampleString’ is assigned a string value.
- In this string, we want to include some double quotes as part of the string content themselves.
- Simply writing double quotes within a string enclosed by double quotes would terminate the string prematurely, hence we need to ‘escape’ these internal quotes.
- We do this by prefixing each internal quote with a backslash (\). This tells JavaScript to interpret the following character (the quote) as a string character, rather than a special character.
- So, the string “”She said, \””Hello, world!\”””” translates to: She said, “”Hello, world!””
#2 – By using template literals ()
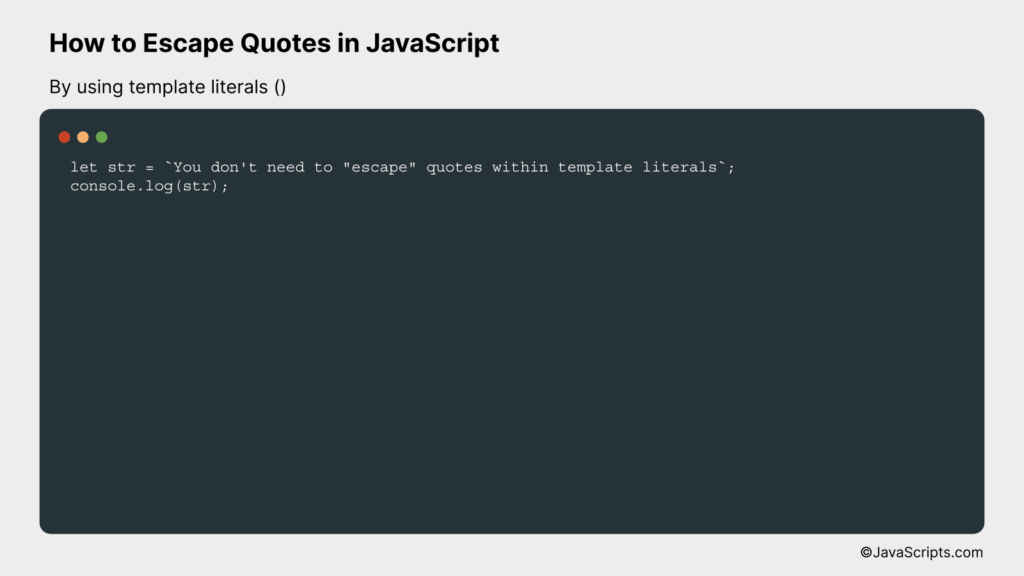
In JavaScript, you can use template literals (backticks ` `) to escape quotes. This process enables you to include special characters in a string without breaking the code. Here is an example:
let str = `You don't need to ""escape"" quotes within template literals`;
console.log(str);
How it works
Template literals (backticks ` `) in JavaScript allow the inclusion of variables, expressions, and special characters without the need for concatenation or escape sequences.
- Firstly, We create a variable ‘str’ and assign a string to it. The string includes both single and double quotes.
- The string is enclosed in backticks (` `), which are located at the top left of most keyboards, usually under the tilde (~).
- Unlike traditional strings in JavaScript that require escape sequences (like \’) for special characters or different quotes, template literals automatically escape any special character or different types of quotes included within them.
- Finally, when we log the ‘str’ variable to the console, the output includes all the characters without any syntax errors, demonstrating that the quotes were successfully escaped.
#3 – By using escape() method
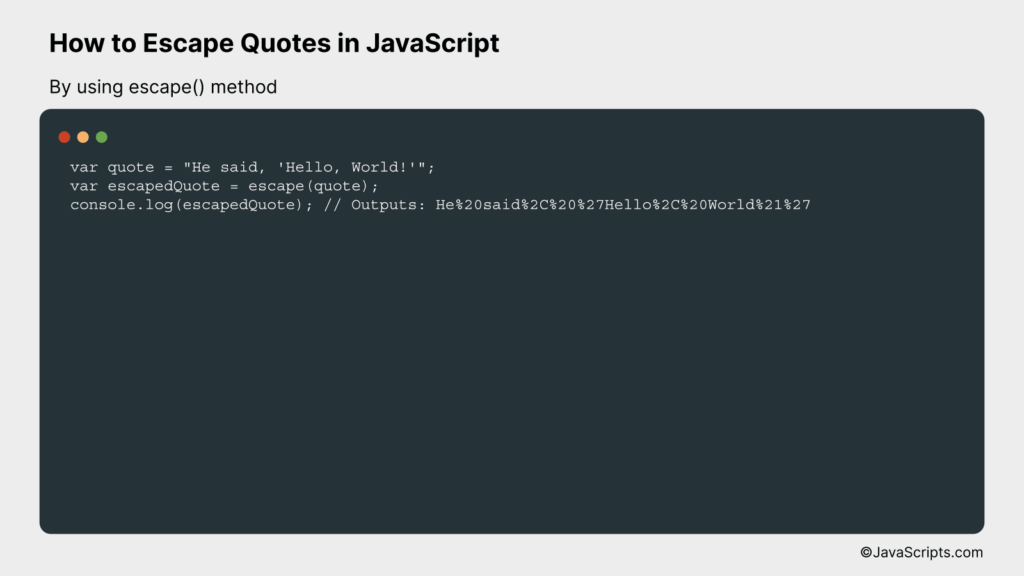
The escape() method in JavaScript allows you to convert special characters, including quotes, into their corresponding UTF-8 escape sequences, ensuring they are interpreted correctly in your code. For example, a single quote (‘), when escaped, becomes %27.
var quote = ""He said, 'Hello, World!'"";
var escapedQuote = escape(quote);
console.log(escapedQuote); // Outputs: He%20said%2C%20%27Hello%2C%20World%21%27
How it works
The escape() function works by reading the input string character by character and replacing special characters, like quotes, with their UTF-8 escape sequence.
- First, we define a string variable
quote
that contains a text with single quotes. - Then, we use the JavaScript
escape()
method on thequote
string, and we store the result in a new variable,escapedQuote
. - This method will replace any special characters in the string with their corresponding UTF-8 escape sequences.
- In our case, the single quotes (‘), spaces ( ) and the comma (,) in the
quote
string are replaced by%27
,%20
and%2C
respectively. - Finally, we log the
escapedQuote
to the console, where you can see the original string with the special characters now replaced by their escape sequences.
Related:
- How to Download Files from URLs with JavaScript
- How to Check if a String Contains a Substring in JavaScript
- How to Change Image Source in JavaScript
In conclusion, understanding how to escape quotes in JavaScript is vital for any coder. It helps maintain the integrity of your strings, preventing them from breaking your code due to unescaped quotes.
Remember, you can escape quotes by using a backslash before them. Practicing this often is key to making it second nature. Happy coding!