How to Print Arrays in JavaScript
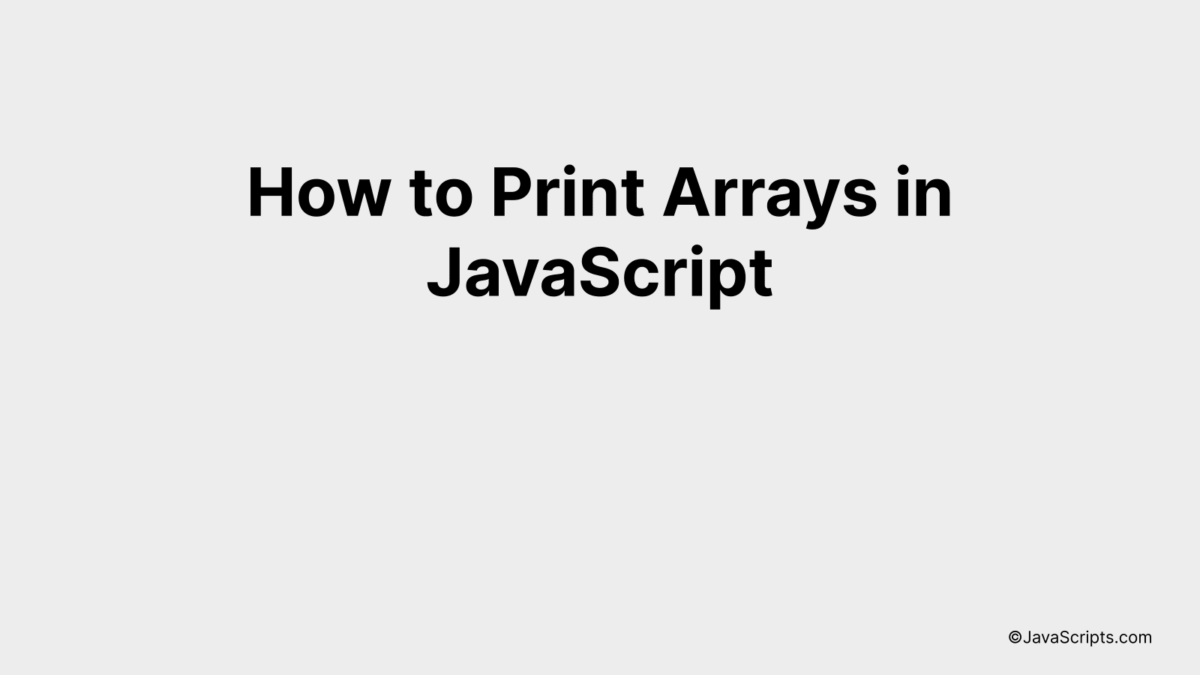
JavaScript is a powerful language that you and I use almost every day. It’s the engine behind most interactive web content, making sites dynamic and user-friendly. One of the cornerstones of JavaScript is its ability to handle arrays, which are lists of items that could be numbers, strings, or even other objects.
Printing these arrays may seem like a daunting task when you’re new to coding. However, it’s simpler than you think! With the right tools and techniques, you can easily print arrays in JavaScript and take your coding skills to the next level.
In this post, we will be looking at the following 3 ways to print arrays in JavaScript:
- By using console.log() method
- By using forEach() method
- By using join() method
Let’s explore each…
#1 – By using console.log() method
The console.log() function in JavaScript is used to print any kind of variables defined before in it or just to print any message that needs to be displayed to the user. To print an array, you simply pass the array variable to the console.log() function.
let arr = [1, 2, 3, 4, 5];
console.log(arr);
How it works
The array ‘arr’ is initialized with values 1, 2, 3, 4, 5 and then it’s printed in the console using the console.log() function. The output will be the array itself.
- First, the array ‘arr’ is defined and initialized with the values 1, 2, 3, 4, 5. This creates an array in memory.
- Then, the console.log() function is called with ‘arr’ as an argument. This tells JavaScript to print the contents of ‘arr’ to the console.
- The JavaScript interpreter sees the console.log() function and sends the value of ‘arr’ to the console.
- The console then displays the array [1, 2, 3, 4, 5].
#2 – By using forEach() method
In JavaScript, the forEach() method executes a provided function once for each array element. You can use this method to print each element of an array. For a better understanding, let’s consider an example.
let arr = [1, 2, 3, 4, 5];
arr.forEach(function(element) {
console.log(element);
});
How it works
The forEach() method iterates over the array and for each element, it executes the function. In this case, it prints each element to the console.
- Step 1: We declare an array (arr) with five elements – 1, 2, 3, 4, 5.
- Step 2: We call the forEach() method on this array. This method takes one argument, which is a function.
- Step 3: The function we pass to forEach() also takes one argument (element), which represents the current item being processed in the array.
- Step 4: Inside the function, we use console.log(element) to print the current item to the console.
- Step 5: The forEach() method does this for every item in the array, hence we see all elements printed out in the console.
#3 – By using join() method
The JavaScript join() method combines the elements of an array into a string and separates them with a specific separator. Here’s an example of how you can use it to print arrays:
let fruits = ['Apple', 'Banana', 'Cherry'];
let result = fruits.join(', ');
console.log(result);
How it works
The join() method is a built-in JavaScript function that takes all the elements of an array and combines them into a single string. In the example above, we used a comma followed by a space as the separator between the elements.
Here’s what happens step-by-step:
- Step 1: An array of fruits is created with three elements: ‘Apple’, ‘Banana’, and ‘Cherry’.
- Step 2: The join() method is called on the fruits array. It’s provided with a string ‘, ‘ which is used as the separator between elements in the resulting string.
- Step 3: The join() method returns a new string which is ‘Apple, Banana, Cherry’. This new string is assigned to the variable result.
- Step 4: The console.log() method is used to print the result to the console.
Related:
- How to Perform String Empty Check in JavaScript
- How to Perform Date Subtraction in JavaScript
- How to Handle Dates in UTC with JavaScript
In conclusion, printing arrays in JavaScript is a straightforward process. We have several methods to do this, such as by simply using console.log, or looping through each element in the array with for or forEach loops.
Remember, the method you choose depends on your specific needs and the complexity of your array. Practice with different methods to find what works best for your code. Happy coding!