How to Set Background Color with JavaScript
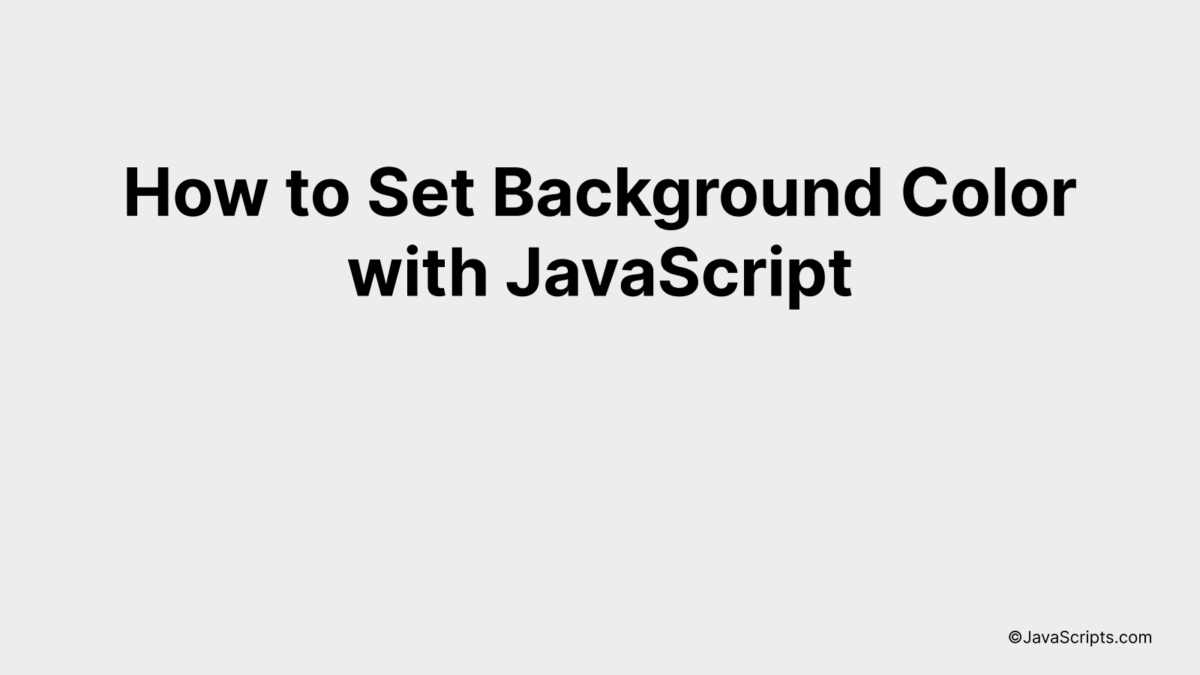
Welcome to a world where programming lets you breathe color into the canvas of your websites. Imagine the ability to change the background color of your web pages using just a few lines of JavaScript code. Fascinating, isn’t it?
You and I, together, will venture into this vibrant journey of JavaScript. We’ll explore the tricks of dynamically manipulating the background color of web elements, making your site interactive and lively. Buckle up for an exciting adventure!
In this post, we will be looking at the following 3 ways to set background color with JavaScript:
- By using the style property
- By using the querySelector method
- By using the getElementById method
Let’s explore each…
#1 – By using the style property
The task here is to change the background color of an HTML element using JavaScript’s style property. We’ll do this by accessing the element and then changing its ‘backgroundColor’ property. Here’s an illustrative example:
document.getElementById("myElement").style.backgroundColor = "blue";
How it works
This script works by first using the ‘getElementById’ method to select the HTML element with the id ‘myElement’, and then using the ‘style’ property to access the element’s style attribute, specifically the ‘backgroundColor’ property, which it then sets to the string ‘blue’.
- Step 1: JavaScript uses
document.getElementById("myElement")
to find the HTML element with the id ‘myElement’. - Step 2: The
style
property of the HTML element is accessed. This refers to the inline style of the element. - Step 3: The
backgroundColor
property is set to ‘blue’. This changes the background color of the selected element to blue.
#2 – By using the querySelector method
This task involves changing the background color of an HTML element using JavaScript’s querySelector method. By targeting the element via CSS selectors and then altering its style properties, we can easily modify the background color.
document.querySelector("#myElement").style.backgroundColor = "blue";
How it works
The JavaScript querySelector method allows us to select a specific HTML element using CSS selectors. After selection, we can manipulate the element’s style properties, such as its background color.
- document.querySelector(“#myElement”): This part of the code selects the HTML element with the id “myElement”. The “#” symbol is a CSS selector that allows us to target elements based on their id.
- .style.backgroundColor: After selecting the element, we use “.style.backgroundColor” to access the background color property of that element. The “style” is an object representing the element’s style attribute, and “backgroundColor” is one of the many properties that it has.
- = “blue”: Finally, we assign the new color value to the backgroundColor property. Here, we’re setting the background color of the selected element to blue.
#3 – By using the getElementById method
This method will allow you to change the background color of a specific HTML element by its ID using JavaScript. The ‘getElementById’ method is used to select the HTML element and then the ‘style.backgroundColor’ property is used to change the background color. The following code sample demonstrates this.
document.getElementById('yourElementId').style.backgroundColor = 'red';
How it works
This line of JavaScript code is accessing an HTML element using its ID and then changing its background color. The ‘getElementById’ method is part of the ‘document’ object, and it allows you to access the first element in the document with the provided ID. Once the element is accessed, ‘.style.backgroundColor’ is used to change the background color of the element.
- First, ‘document.getElementById(‘yourElementId’)’ is used. This accesses the HTML document and finds the first element with the ID ‘yourElementId’.
- Second, ‘.style.backgroundColor’ is appended to that. This accesses the style object of the selected HTML element.
- The ‘= “red”‘ at the end is assigning the value ‘red’ to the ‘backgroundColor’ property of the style object. This changes the background color of the selected HTML element to red.
Related:
- How to Replace DIV Content with JavaScript
- How to Remove Substrings from Strings in JavaScript
- How to Perform Case-Insensitive Includes in JavaScript
In conclusion, setting a background color with JavaScript is a straightforward and effective way to enhance the look of your webpage. With few lines of code, you can transform the user experience, offering a more dynamic and engaging interface.
Remember, it’s essential to pick colors that complement your site’s overall design. So, experiment with different shades till you find a perfect fit. Happy coding!