How to Access JavaScript Text Content
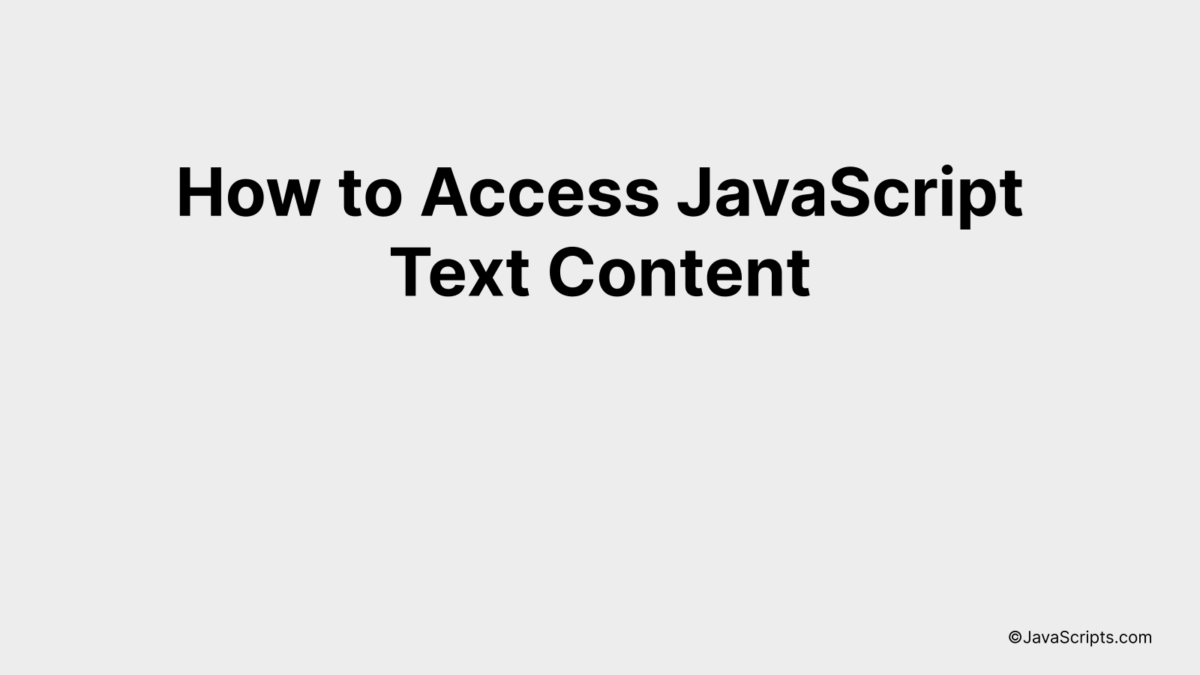
JavaScript is a powerful tool that helps us breathe life into static web pages, enabling interaction and dynamism. You and I both know that mastering its various facets can empower us to create remarkable websites and web applications.
One such crucial aspect of JavaScript is accessing text content. This skill is fundamental to manipulating and interacting with web page elements. Don’t worry if you’re not yet sure how to do this. Our journey today will delve into simple, understandable steps to master this important aspect.
In this post, we will be looking at the following 3 ways to access JavaScript text content:
- By innerText property
- By textContent property
- By using Node.textContent
Let’s explore each…
#1 – By innerText property
The JavaScript property ‘innerText’ retrieves the text content of the specified node and all its descendants. This means any text inside the HTML element can be accessed using this property. Let’s take an example to understand this better.
let element = document.getElementById('myElement');
let textContent = element.innerText;
How it works
The ‘innerText’ property in JavaScript is used to get the combined text contents of each node in the specified element. It differs from ‘textContent’ in that it ignores HTML tags, providing only the readable text content.
- The variable ‘element’ is assigned the HTML element with the ID ‘myElement’. This is done using the ‘getElementById’ method of the ‘document’ object in JavaScript. This method returns the first element in the document with the specified identifier.
- Then, the ‘innerText’ property of the ‘element’ object (the HTML element) is accessed and stored in the ‘textContent’ variable. This will contain all the text that is inside the HTML element, excluding any HTML tags.
#2 – By textContent property
textContent property in JavaScript is used to set or return the text content of the specified node, and all its descendants. Here’s an example where we get the text content of an HTML element with the id “myElement”.
var text = document.getElementById("myElement").textContent;
console.log(text);
How it works
The textContent property in JavaScript allows you to access or modify the textual content within an HTML element. It’s versatile as it can get or set the content for an element and all its children.
- First, the document.getElementById(“myElement”) code is used to locate the HTML element with the id ‘myElement’.
- Next, the .textContent is called on this HTML element. This property accesses the text contained within the ‘myElement’ element.
- The value of this text is then stored in the variable ‘text’.
- The console.log(text) line of code is used to print the value of ‘text’ to the console, displaying the text content of ‘myElement’.
#3 – By using Node.textContent
The ‘Node.textContent’ property in JavaScript is used to set or return the textual content of a node and its descendants. It allows you to retrieve or update the text content of an element without interfering with any child tags.
const element = document.getElementById('myElement');
let textContent = element.textContent;
How it works
The ‘Node.textContent’ property gets the text content of an element and its descendants. If you set the ‘textContent’ property, any child nodes are removed and replaced by a single text node containing the specified string.
- The ‘document.getElementById(‘myElement’)’ expression is used to select the HTML element with the id ‘myElement’.
- The ‘textContent’ property of ‘element’ (the selected HTML element) is accessed and its value is stored in the ‘textContent’ variable.
- If you wanted to set or change the text content, you could simply assign a new value to ‘element.textContent’.
Related:
- How to Check if an Array is Empty in JavaScript
- How to Break from a JavaScript ForEach Loop
- How to Round to 2 Decimal Places in JavaScript
In conclusion, accessing JavaScript text content is a fundamental skill for any web developer. The process is straightforward, using methods such as .textContent, .innerHTML or .innerText to retrieve or change the text within HTML elements.
These methods give us the ability to interact with website content, allowing us to make dynamic, user-responsive pages. Remember, consistent practice is the key to mastering these techniques. So, keep coding and experimenting!