How to Add Properties to Objects in JavaScript
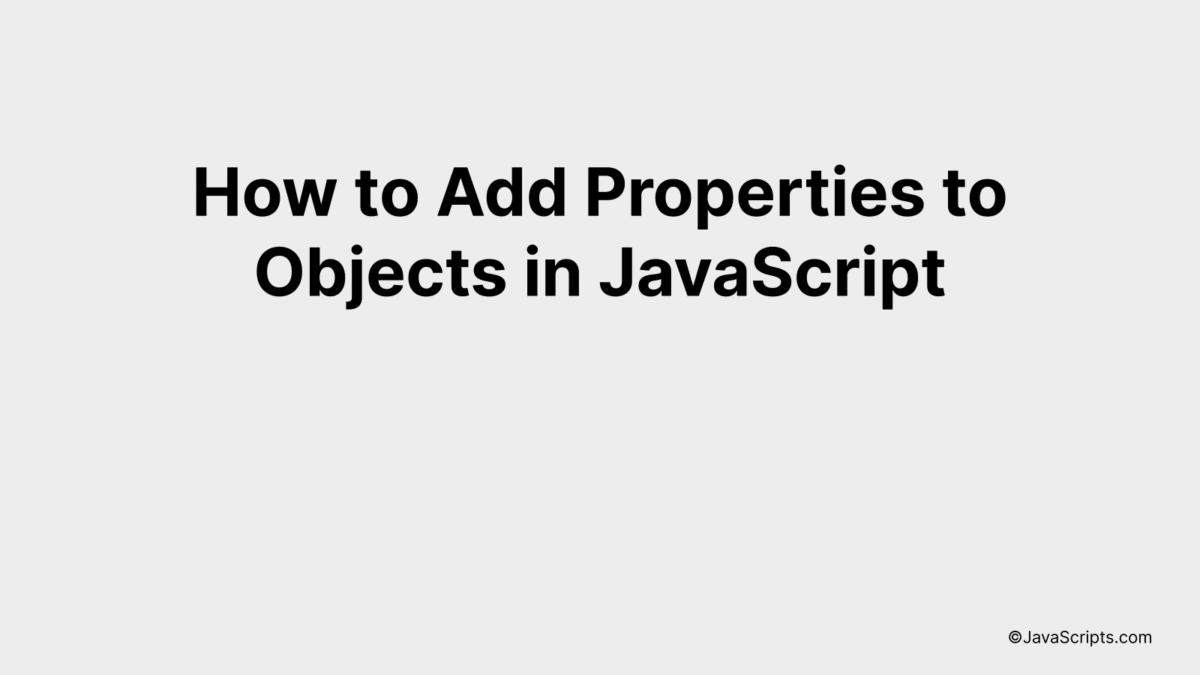
Adding properties to objects is a fundamental aspect of JavaScript, a dynamic and flexible programming language. Whether you’re a seasoned programmer or just starting out, mastering this concept can take your coding skills to the next level.
You might wonder, “Why are properties so important?” Well, properties in JavaScript provide a way to store, manipulate, and access data within objects. They are the building blocks that create the structure and outline of objects, making your code more organized and efficient.
In this post, we will be looking at the following 3 ways to add properties to objects in JavaScript:
- By Dot Notation
- By Bracket Notation
- By using the Object.defineProperty() method
Let’s explore each…
#1 – By Dot Notation
Adding properties to objects in JavaScript by using dot notation allows you to directly access and modify the object’s properties. It is as simple as specifying the object name followed by a dot (.), then the property name, and finally assigning the property a value, for example, objectName.propertyName = “Some Value”.
let car = {};
car.make = "Tesla";
car.model = "Model S";
car.year = "2022";
How it works
When you use the dot notation to add properties to an object in JavaScript, you are essentially extending the object’s structure by adding new fields (properties) to it. In our example, we added ‘make’, ‘model’, and ‘year’ properties to the ‘car’ object.
- Firstly, we create an empty object ‘car’ using let car = {};
- Then, we add a property ‘make’ to the ‘car’ object and assign it the value “Tesla” using car.make = “Tesla”;
- Next, we add a property ‘model’ to the ‘car’ object and assign it the value “Model S” using car.model = “Model S”;
- Finally, we add a property ‘year’ to the ‘car’ object and assign it the value “2022” using car.year = “2022”;
- Now, our ‘car’ object contains three properties make, model, and year, which can be accessed as car.make, car.model, and car.year respectively.
#2 – By Bracket Notation
In JavaScript, you can add properties to objects using bracket notation, where the property name (as a string) is enclosed within square brackets. An example will make it clearer.
var student = {};
student['name'] = 'John Doe';
student['age'] = 22;
How it works
In the above JavaScript code, we’re dynamically adding properties to an object using bracket notation.
- First, we create an empty object named ‘student’.
- Then, we use bracket notation to add a ‘name’ property to the ‘student’ object and set its value to ‘John Doe’.
- Similarly, we add an ‘age’ property to the ‘student’ object and set its value to 22. Bracket notation is particularly useful when the property name is not known until runtime or when it includes special characters or spaces.
#3 – By using the Object.defineProperty() method
The Object.defineProperty() method in JavaScript adds new properties to an object, or modifies existing ones, by directly defining them along with their attributes, providing greater control over how the property behaves. An example will provide a clearer understanding of its use.
var person = {};
Object.defineProperty(person, 'name', {
value: "John Doe",
writable: true,
enumerable: true,
configurable: true
});
How it works
The example above uses the Object.defineProperty() method to add a property named ‘name’ with the value “John Doe” to the ‘person’ object. It also sets the properties ‘writable’, ‘enumerable’, and ‘configurable’ to true, providing specific characteristics to the property.
- Step 1: A new JavaScript object ‘person’ is created.
- Step 2: The Object.defineProperty() method is called on ‘person’ to define a new property.
- Step 3: The second argument to the method is the name of the property, in this case ‘name’.
- Step 4: The third argument is an object that describes characteristics of the property. It defines ‘name’ with the value “John Doe” (value), makes it changeable (writable), visible when enumerating over the object’s properties (enumerable), and allows its characteristics to be altered (configurable).
Related:
- How to Wrap Elements with JavaScript
- How to Sort Maps in JavaScript
- How to Replace Multiple Strings in JavaScript
In conclusion, JavaScript offers various methods to add properties to objects, giving us the flexibility and control we need in our programming tasks. Whether you choose to use dot notation, bracket notation, or the Object.defineProperty method, the power to tailor your objects according to your needs is in your hands.
Remember that practice makes perfect. Keep exploring the properties and methods of JavaScript objects, as understanding them will significantly enhance your problem-solving skills in coding. Embrace the journey of learning JavaScript, and happy coding!