How to Check for an Empty String in JavaScript
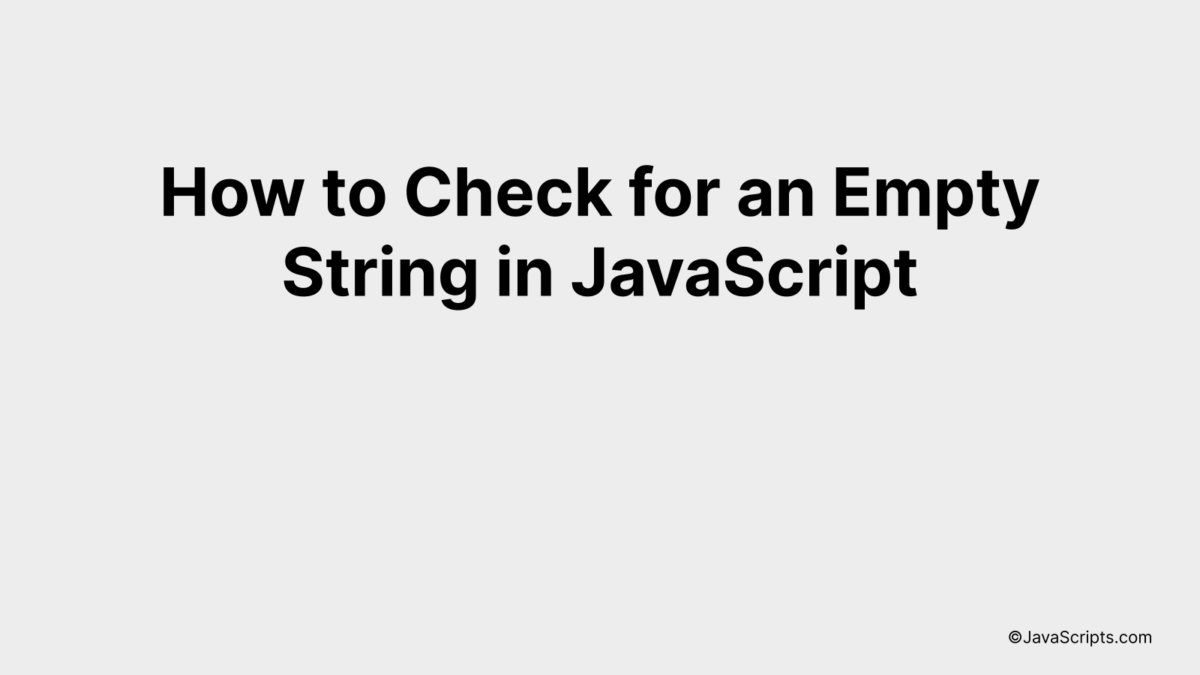
Managing data types is an essential part of any programming language, and JavaScript is no exception. Strings, in particular, are a common data type that we handle regularly. Sometimes, we need to verify whether a string is empty or not. But how do we do that?
You’ll be surprised to know it’s simpler than you might think. With a few straightforward methods, we can efficiently perform this check in JavaScript. Let’s delve into the specifics together, and by the end, you’ll be able to handle empty strings with great ease and confidence.
In this post, we will be looking at the following 3 ways to check for an empty string in JavaScript:
- By using the strict equality operator (===)
- By using the not operator (!)
- By using the String.prototype.trim() method
Let’s explore each…
#1 – By using the strict equality operator (===)
In JavaScript, you can use the strict equality operator (===) to check if a string is empty. This operator compares both the type and the value, so if a string is empty, it will return true, otherwise false. For instance, if we have a string variable, we can check if it’s empty by comparing it with an empty string (“”).
let myString = "";
if(myString === "") {
console.log("The string is empty!");
} else {
console.log("The string is not empty!");
}
How it works
This code uses JavaScript’s strict equality operator to determine whether a string is empty. If the string is indeed empty, it outputs a message saying “The string is empty!”, if not, it informs “The string is not empty!”.
- First, we declare a variable
myString
and initialize it with an empty string. - Next, we use an
if
statement to check ifmyString
is strictly equal to an empty string. - The strict equality operator (===) compares both the type and the value. So, if
myString
is an empty string, the conditionmyString === ""
will be true. - If the condition is true, then the message “The string is empty!” will be logged on the console.
- If the condition is false (meaning the string is not empty), then the message “The string is not empty!” will be logged on the console.
#2 – By using the not operator (!)
In JavaScript, you can check if a string is empty by using the not operator (!). This operator returns ‘false’ for a non-empty string and ‘true’ for an empty string. This is because an empty string is considered a falsy value and the not operator negates it.
let str = "";
if (!str) {
console.log("The string is empty");
} else {
console.log("The string is not empty");
}
How it works
In JavaScript, different values are considered as either “truthy” or “falsy”. An empty string is a falsy value. So when we use the not operator ‘!’ with a falsy value, it returns ‘true’. Hence, if a string is empty, ‘!str’ will return ‘true’ and if string is not empty, ‘!str’ will return ‘false’.
- The variable ‘str’ is assigned an empty string.
- The ‘if’ condition checks the negation of ‘str’. If ‘str’ is an empty string, ‘!str’ will be ‘true’ and the code inside the ‘if’ block will be executed.
- The ‘console.log’ inside the ‘if’ block will output “The string is empty” if ‘str’ is an empty string.
- If ‘str’ is not an empty string, ‘!str’ will be ‘false’ and the code inside the ‘else’ block will be executed. The ‘console.log’ inside the ‘else’ block will output “The string is not empty”.
#3 – By using the String.prototype.trim() method
The String.prototype.trim() method will check if a string is empty by removing white spaces from both ends of the string. If, after trimming, the string is empty, it means the original string was either empty or contained only white spaces. Let’s demonstrate this with an example.
function isEmpty(str) {
return !str.trim();
}
How it works
The function isEmpty(str) takes a string input and checks if it’s empty after being trimmed of leading and trailing white spaces. It does this by using the trim() method on the string and then negating the result. If the trimmed string is empty, the function will return true, otherwise it will return false.
- Firstly, the str.trim() method is called on the string input. This method removes white spaces from both ends of the string. It doesn’t affect the spaces between the words within the string.
- After trimming the string, we have either an empty string (if the original string was empty or contained only white spaces) or a non-empty string.
- Next, we use the logical NOT operator ! to negate the result. In JavaScript, an empty string is a falsy value, meaning it evaluates to false in a boolean context. Likewise, a non-empty string is a truthy value, so it evaluates to true.
- By using the NOT operator !, we’re effectively checking if the string is empty. If str.trim() is an empty string, !str.trim() will return true, indicating that the original string was empty or contained only white spaces. If str.trim() is a non-empty string, !str.trim() will return false, indicating that the original string was not empty.
Related:
- How to Sort Dates in JavaScript
- How to Perform Date Addition in JavaScript
- How to Group Elements by Date in JavaScript
To wrap up, checking for an empty string in JavaScript isn’t as daunting as it initially seems. With various methods available like the trim(), length property or type coercion, you can easily find if a string is empty.
In the end, your chosen method should align with the specific requirements of your code. Remember, practice makes perfect. So, the more you code and test, the better you’ll understand these concepts.