How to Check if a Variable is Defined in JavaScript
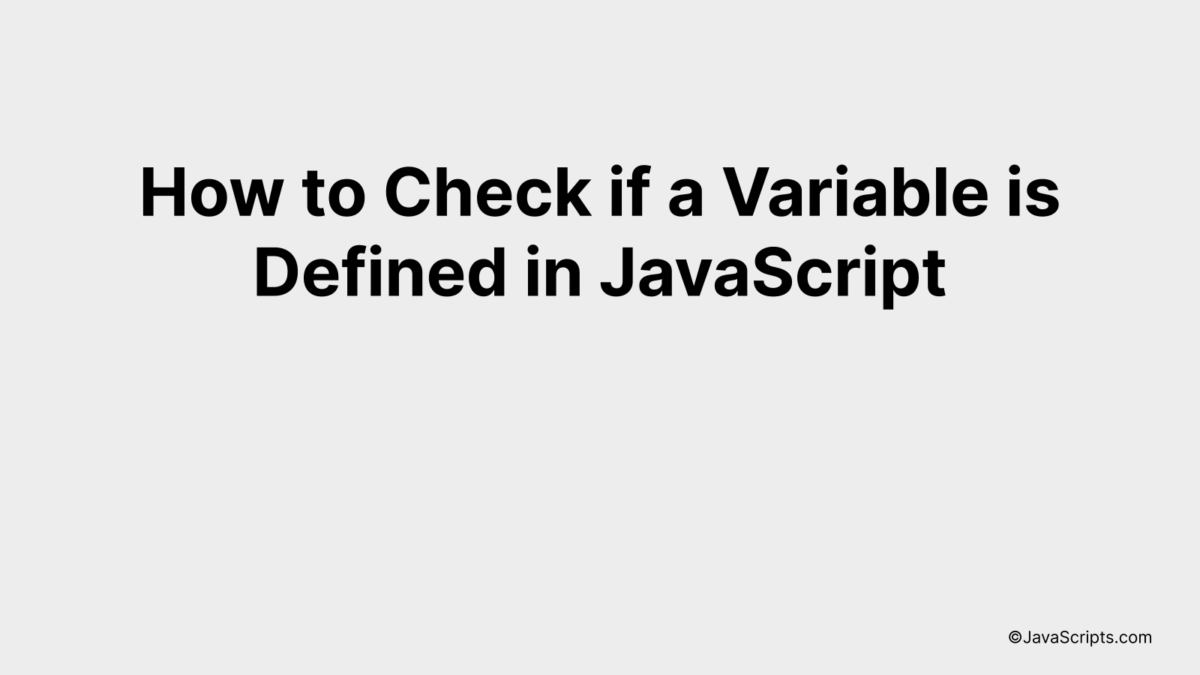
Have you ever stumbled upon a seemingly unexplained ‘undefined’ error while coding in JavaScript? You’re not alone. It’s a common issue, usually stemming from variables that have not been defined yet.
Guess what? There’s a straightforward way to avoid these pesky errors. The secret lies in learning how to properly check if a variable is defined. That’s exactly what you and I will discover together. We’ll keep it simple, so even if you’re new to JavaScript, you’ll find it easy to understand.
In this post, we will be looking at the following 3 ways to check if a variable is defined in JavaScript:
- By using the “typeof” operator
- By using the “in” operator
- By trying to catch a ReferenceError exception using a “try…catch” statement
Let’s explore each…
#1 – By using the “typeof” operator
The “typeof” operator in JavaScript allows you to check if a variable is defined or not. It returns a string indicating the type of the unevaluated operand, or “undefined” if the variable has not been declared or defined.
let exampleVariable;
console.log(typeof exampleVariable);
exampleVariable = "This is a string";
console.log(typeof exampleVariable);
How it works
The ‘typeof’ operator checks the data type of its operand (the variable or value you’re checking). If the variable has been defined, it returns a string indicating the type of the operand, such as ‘string’, ‘number’, etc. If the variable is not defined, it returns ‘undefined’.
- First, we declare a variable ‘exampleVariable’ without assigning any value to it.
- Then, we use the ‘typeof’ operator to check the type of ‘exampleVariable’. As no value was assigned to the variable, it’s ‘undefined’. The console output at this stage will be ‘undefined’.
- Next, we assign a string value to ‘exampleVariable’.
- Finally, we again use ‘typeof’ operator to check the type of ‘exampleVariable’. Now it has a value, which is a string, so ‘typeof’ returns ‘string’. The console output at this stage will be ‘string’.
#2 – By using the “in” operator
In JavaScript, you can use the “in” operator to check if a property exists within an object. If the property is found, it will return true; otherwise, it will return false. This can be used to conveniently check if a variable is defined in the global scope (i.e., as a property of the window object).
let exampleVariable;
let isVariableDefined = "exampleVariable" in window;
How it works
The “in” operator in JavaScript checks if a certain property exists within a given object. In the context of checking if a variable is defined, we use the “window” object, which represents the global scope in a browser environment. If the variable is defined in the global scope, it will be a property of the window object.
- The variable “exampleVariable” is declared. Without assigning a value, JavaScript treats it as “undefined”, but the variable itself is defined in the global scope.
- Then, the “in” operator is used to check if “exampleVariable” is a property of the window object. The name of the variable is passed as a string to the “in” operator.
- If “exampleVariable” has been defined, “isVariableDefined” will be set to true. Otherwise, it will be false.
#3 – By trying to catch a ReferenceError exception using a “try…catch” statement
In JavaScript, we can check if a variable is defined or not by utilizing the “try…catch” statement. This method works by attempting to access a variable in the “try” block. If the variable is not defined, it will throw a ReferenceError, which will be caught in the “catch” block, allowing us to handle the error in a controlled manner.
let isDefined;
try {
isDefined = typeof myVar !== 'undefined';
} catch (error) {
if (error instanceof ReferenceError) {
isDefined = false;
}
}
console.log(isDefined);
How it works
This code sample is trying to access the variable ‘myVar’. If ‘myVar’ is not defined in the scope, it throws a ReferenceError. This error is then caught in the catch block where we check if the error is an instance of ReferenceError. If it is, we assume that the variable is not defined, so we set ‘isDefined’ to false.
- The code initializes an ‘isDefined’ variable.
- It then enters a ‘try’ block where it tries to access the ‘myVar’ variable. The ‘typeof’ operator is used to check the type of ‘myVar’. If ‘myVar’ is undefined, ‘typeof myVar’ will return ‘undefined’.
- If ‘myVar’ is not defined, JavaScript will throw a ReferenceError.
- This error is then caught in the ‘catch’ block. Inside this block, there is a conditional statement which checks if the error is an instance of ReferenceError.
- If the error is a ReferenceError, it means that ‘myVar’ is not defined, so the code sets ‘isDefined’ to false.
- Finally, the value of ‘isDefined’ is logged to the console. If ‘myVar’ was not defined, ‘isDefined’ would be false, otherwise it would be true.
Related:
- How to Check if a Function Exists in JavaScript
- How to Use One-Line If Statements in JavaScript
- How to Uncheck Checkboxes with JavaScript
In conclusion, checking whether a variable is defined in JavaScript is a simple yet crucial aspect of coding. Using methods like ‘typeof’ can save you from potential errors and ensure that your program runs smoothly.
Remember, it’s always good to double-check your code for undefined variables. This way, your code stays clean, efficient, and error-free. Improving this habit will surely make you a better programmer in the future.