How to Uncheck Checkboxes with JavaScript
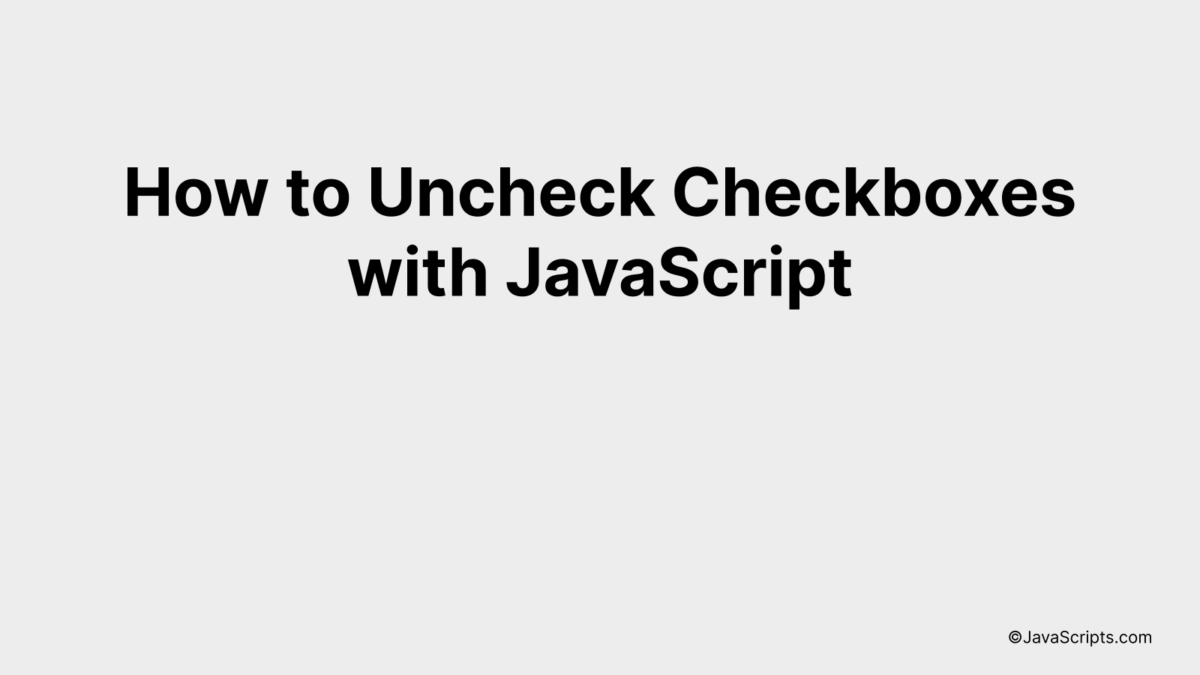
Navigating through JavaScript can seem like a daunting task, especially when you’re just getting started. But don’t worry, you and I are about to harness the power of this dynamic language to perform a simple, yet essential task: unchecking checkboxes.
Working with checkboxes is a common scenario in web development, often needed to control user interactions. Let’s embark on a journey to learn a straightforward way to uncheck checkboxes using JavaScript, making your website more interactive and user-friendly.
In this post, we will be looking at the following 3 ways to uncheck checkboxes with JavaScript:
- By using the .checked property
- By using the setAttribute() method
- By using the removeAttribute() method
Let’s explore each…
#1 – By using the .checked property
The ‘.checked’ property in JavaScript is used to set or return the checked state of a checkbox. This means you can use it to check whether a checkbox is checked (true) or not (false), or to set the checkbox to checked or unchecked. In the following example, we will use JavaScript to uncheck a checkbox with the id ‘myCheckbox’.
document.getElementById('myCheckbox').checked = false;
How it works
The line of code selects an HTML element with the id ‘myCheckbox’, and sets its ‘checked’ property to false, effectively unchecking the checkbox.
- Firstly, the
document.getElementById('myCheckbox')
part of the code allows us to target or select the HTML element with the id ‘myCheckbox’. - Next, the
.checked
property is a property that belongs to checkbox elements. This property holds the current state (checked or unchecked) of the checkbox. - Lastly, by setting it equal to
false
, we are unchecking the checkbox. If it was set totrue
, the checkbox would be checked.
#2 – By using the setAttribute() method
The setAttribute() method in JavaScript allows you to change the value of an attribute in an HTML element. In the context of checkboxes, you can use it to uncheck a checkbox by changing the ‘checked’ attribute’s value to false. Here’s an example:
document.getElementById('checkboxId').setAttribute('checked', false);
How it works
The setAttribute() method is used to modify the value of the specified attribute of an HTML element. In the given code example, it is used to uncheck a checkbox by changing the ‘checked’ attribute to false.
- Step 1: The
document.getElementById('checkboxId')
part of the code fetches the HTML element with the id ‘checkboxId’. Here ‘checkboxId’ should be replaced with the actual id of the checkbox you want to uncheck. - Step 2: The
.setAttribute()
method is a built-in JavaScript method that changes the value of an attribute of the selected HTML element. It takes two parameters: the name of the attribute to modify, and the new value for the attribute. - Step 3: Here, the attribute we are modifying is ‘checked’, and the new value we are setting is ‘false’. This effectively unchecks the checkbox, as the ‘checked’ attribute determines whether a checkbox is checked (true) or unchecked (false).
#3 – By using the removeAttribute() method
The removeAttribute() method in JavaScript allows us to remove a specific attribute from an HTML element. In this case, we will use it to remove the ‘checked’ attribute of a checkbox, effectively unchecking it. An example will clarify this concept further.
document.getElementById('myCheckbox').removeAttribute('checked');
How it works
The script targets a checkbox with a specific ID and removes its ‘checked’ attribute. This action has the effect of unchecking the checkbox.
- First, the script uses the document.getElementById() function to select the checkbox with the ID ‘myCheckbox’.
- Next, it applies the removeAttribute() method to this selected checkbox.
- The ‘checked’ attribute, which determines whether a checkbox is checked or not, is passed as a parameter to the removeAttribute() method.
- By removing this attribute, the checkbox is effectively unchecked.
Related:
- How to Split an Array into Chunks in JavaScript
- How to Set Background Color with JavaScript
- How to Replace DIV Content with JavaScript
In conclusion, JavaScript offers straightforward, efficient ways to uncheck checkboxes. Grasping these methods adds versatility to your web development skill set and allows you to create more dynamic forms.
This guide has shown you how easy it is to uncheck checkboxes using JavaScript. Start practicing these methods and see how they work for your unique web projects.