How to Flatten Objects in JavaScript
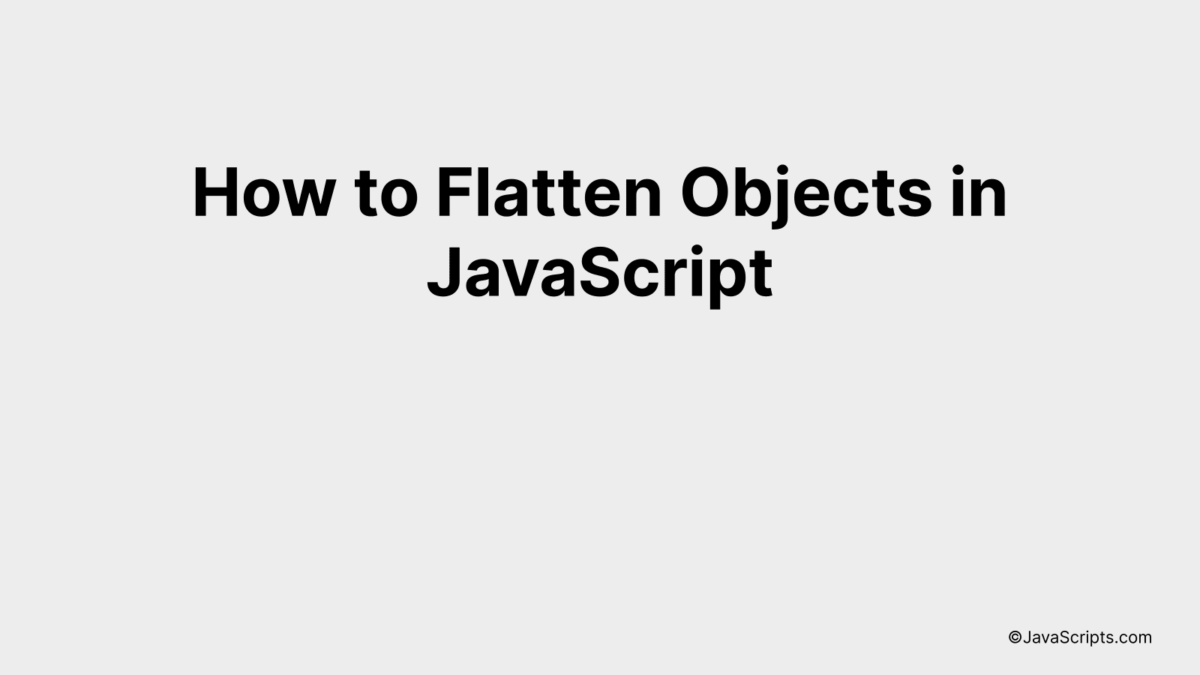
JavaScript objects, charming in their versatility, can sometimes become labyrinthine structures that defy easy understanding. Let’s face it, we’ve all been stumped by a deeply nested object that seems almost impossible to unravel.
That’s where the concept of ‘flattening’ comes in. Imagine if we could smoothen out these complex objects into a single level, making them far easier to navigate. Together, you and I will explore how to achieve this simplification.
In this post, we will be looking at the following 3 ways to flatten objects in JavaScript:
- By using JSON.stringify() and JSON.parse() method
- By using Object.assign() method
- By using the Spread Operator in JavaScript
Let’s explore each…
#1 – By using JSON.stringify() and JSON.parse() method
This approach involves converting a nested JavaScript object into a JSON string using JSON.stringify(), then parsing it back into a flat JavaScript object using JSON.parse(). This process will be better understood through an example.
var nestedObj = {
a: 1,
b: {
c: 2,
d: {
e: 3
}
}
};
var flattenObj = JSON.parse(JSON.stringify(nestedObj).replace(/({|,)s*(S+?)s*:/g, '$1"'+('$2')+'":'));
How it works
The JSON.stringify() method is used to convert the nested JavaScript object into a JSON string. Then, using a regular expression with the replace() method, all unquoted keys are quoted, which effectively “flattens” the object. Finally, JSON.parse() is used to convert the modified JSON string back into a JavaScript object.
Let’s break down the process step-by-step:
- JSON.stringify(nestedObj): This converts the nested JavaScript object into a JSON string.
- .replace(/({|,)s*(S+?)s*:/g, ‘$1″‘+(‘$2′)+'”:’): This method uses a regular expression to find all instances where object keys are not surrounded by quotes. All unquoted keys are then quoted, thus “flattening” the object.
- JSON.parse(): The JSON string, now with all keys quoted, is parsed back into a JavaScript object.
Please note that this method won’t fully flatten the object, it will only ensure all keys are quoted. If you need to flatten an object (i.e., no nested keys), a different approach would be required.
#2 – By using Object.assign() method
The Object.assign() method in JavaScript is used to flatten objects by copying the enumerable and own properties from one or more source objects to a target object. Below is an example to illustrate this.
let obj1 = {
a: 1,
b: {
c: 2,
d: 3
}
};
let obj2 = Object.assign({}, obj1.b, obj1);
How it works
The Object.assign() method is used to copy the properties and methods from one or more source objects to a target object. It returns the target object. In the example provided, we first declare a target object and then use Object.assign() method to copy all properties from the source objects to the target object. This effectively flattens the object by one level.
- First, an object obj1 is declared with a nested object within it.
- The Object.assign() method is called with an empty object as the target object.
- The source objects, in this case obj1.b (nested object) and obj1, are passed as parameters. Properties from these source objects are copied to the target object in the order they are provided.
- The properties of obj1.b get copied first, followed by the properties of obj1. Since obj1 contains a ‘b’ property itself, it overwrites the ‘b’ property copied from obj1.b, thereby achieving the flattening effect.
- Finally, the resultant object is stored in obj2.
#3 – By using the Spread Operator in JavaScript
The Spread Operator in JavaScript can be used to flatten objects by spreading an object into another object, thereby merging their properties. For example, if you have nested objects, you can flatten them into a single object with all properties at the same level.
let obj1 = {
a: 1,
b: {
c: 2,
d: 3
}
};
let flattenedObj = {...obj1, ...obj1.b};
How it works
The spread operator {…} effectively iterates over the properties of an object and includes them in a new object. In the example above, it is used twice to pull properties from two different parts of our nested structure and merge them into a single, flattened object.
- The variable
obj1
is defined with a nested structure. It has propertiesa
andb
, whereb
is an object with its own propertiesc
andd
. - The spread operator is then used to create a new object
flattenedObj
. It is used twice here: once forobj1
and once forobj1.b
. - When used with
obj1
, it includes the properties ofobj1
inflattenedObj
. - When used with
obj1.b
, it includes the properties ofobj1.b
inflattenedObj
. - The result is a flattened object that contains the properties of both
obj1
andobj1.b
at the same level.
Related:
- How to Check if a Variable is Defined in JavaScript
- How to Check if a Function Exists in JavaScript
- How to Use One-Line If Statements in JavaScript
In conclusion, the process of flattening objects in JavaScript can be effectively achieved through different methods such as using recursion or the built-in “reduce” and “Object.assign” methods. These techniques are essential tools in any JavaScript developer’s toolbelt and can greatly simplify complex nested objects.
Understanding how to flatten objects is fundamental for data manipulation and ensuring efficient code. So, don’t hesitate to dive deeper and experiment with these methods to become more proficient in JavaScript. Practice makes perfect after all!