How to Handle Empty Strings in JavaScript
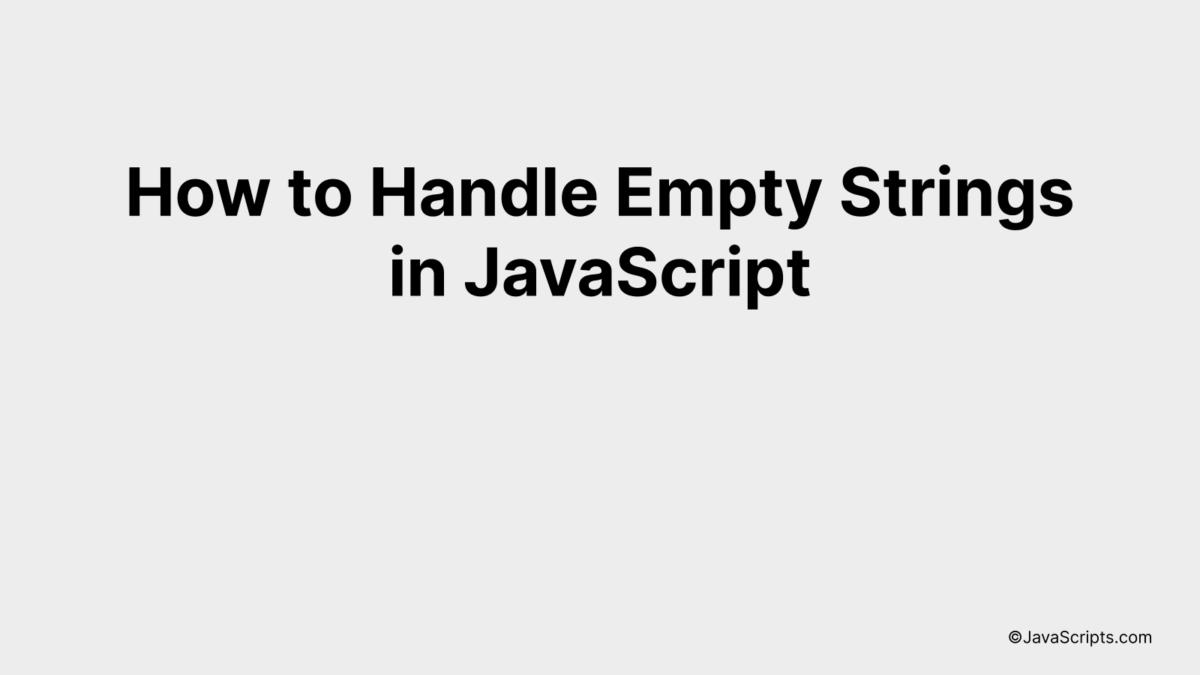
Navigating JavaScript can often be a challenge, especially when dealing with empty strings. They may appear simple, but these innocuous collections of zero characters can sometimes lead to unexpected results in your code.
Don’t fret! You and I will dive deep into understanding how to handle these tricky bits. We’ll learn to identify, validate, and properly manage empty strings to write cleaner and more efficient code.
In this post, we will be looking at the following 3 ways to handle empty strings in JavaScript:
- By using the .length property
- By using the .trim() method
- By comparing with an empty string (”)
Let’s explore each…
#1 – By using the .length property
The .length property in JavaScript can be used to check if a string is empty by comparing the length of the string to 0. If the length is 0, it implies that the string is empty. Here is an example to illustrate this:
var str = "";
if(str.length === 0) {
console.log("String is empty");
} else {
console.log("String is not empty");
}
How it works
The .length property of a string in JavaScript returns the number of characters in the string. In the scenario of an empty string, the length returned would be 0. This property helps us identify if the string is empty.
- The variable
str
is declared and initialized with an empty string. - In the if condition, we are checking the length of the
str
variable using the.length
property. - If the length is 0, it means that the string is empty. The condition
str.length === 0
would be true and “String is empty” will be logged to the console. - If the string is not empty, the length would be greater than 0. Hence, the condition
str.length === 0
would be false and “String is not empty” will be logged to the console.
#2 – By using the .trim() method
The .trim() method in JavaScript is used to remove whitespace from both ends of a string. It can be beneficial to handle empty strings or strings with extra spaces. Here’s an example:
let str = " Hello, World! ";
let trimmedStr = str.trim();
How it works
The JavaScript .trim() method is a built-in function that removes the white space from both ends (left and right) of a string. It does not alter the original string itself, but returns a new string that excludes leading and trailing white spaces.
Here’s a step-by-step explanation of how it works:
- The variable ‘str’ is defined with a string value that contains leading and trailing spaces.
- Next, the .trim() method is called on the ‘str’ variable. This method removes the spaces from both ends of the string.
- The result of ‘str.trim()’ is assigned to a new variable ‘trimmedStr’.
- ‘trimmedStr’ now holds the trimmed string without leading and trailing spaces.
#3 – By comparing with an empty string (”)
We can handle empty strings in JavaScript by using a simple comparison operation to determine whether the string is empty or not. This approach compares the given string with an empty string (”), and returns true if the string is empty and false otherwise. Let’s take a look at a code example for better understanding.
let str = '';
if(str === '') {
console.log('The string is empty');
} else {
console.log('The string is not empty');
}
How it works
The code checks whether the given string ‘str’ is empty or not. It uses the strict equality operator (===) to compare ‘str’ with an empty string (”). If the string is empty, it logs ‘The string is empty’, and if not, it logs ‘The string is not empty’.
- We first initialize a variable ‘str’ and assign an empty string to it.
- Then we use an if statement to check whether ‘str’ is empty or not.
- The if statement uses the strict equality operator (===) to compare ‘str’ with an empty string (”).
- If the condition is true (i.e., ‘str’ is an empty string), it executes the first console.log statement, indicating that the string is empty.
- If the condition is false (i.e., ‘str’ is not an empty string), it executes the second console.log statement, indicating that the string is not empty.
Related:
- How to Format Numbers with Commas in JavaScript
- How to Format Dates as yyyy-mm-dd in JavaScript
- How to Check for Empty Strings in JavaScript
In conclusion, handling empty strings in JavaScript is fairly straightforward once we understand the associated intricacies. It’s important to validate and sanitize inputs to avoid empty strings disrupting the flow of our code.
Practices like using trim(), type checking or using truthy and falsy values, can significantly reduce the possibility of errors. Be mindful of these approaches and your JavaScript code will be more robust and reliable.