How to Check for Empty Strings in JavaScript
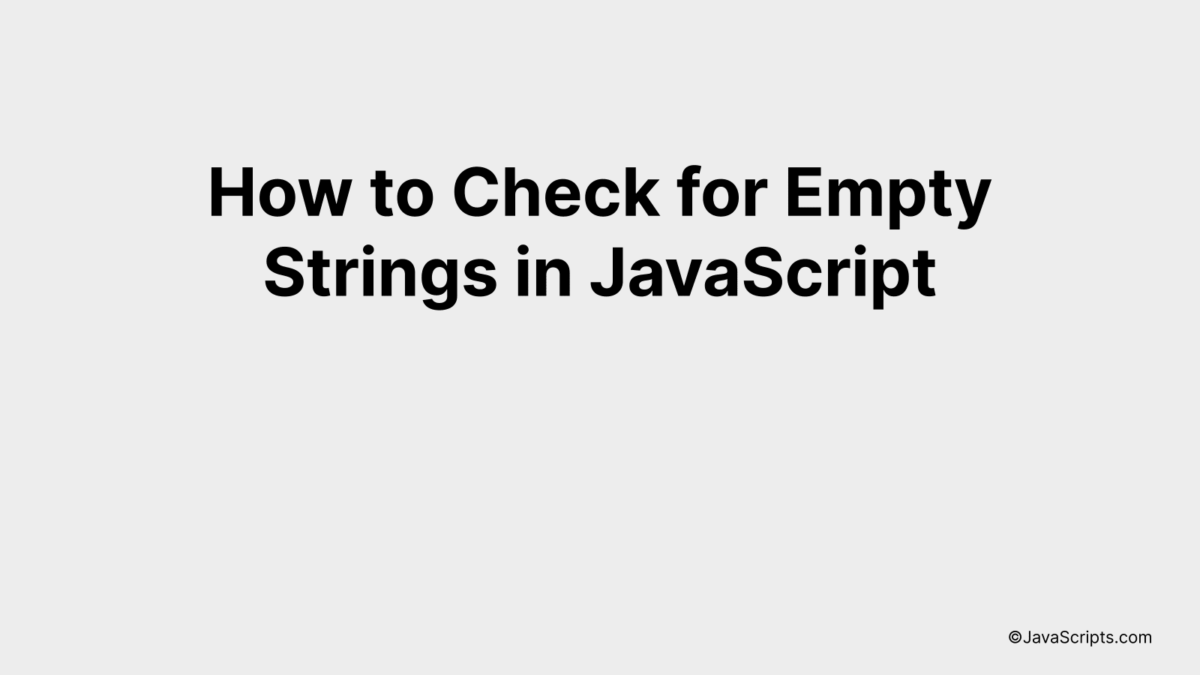
JavaScript, a dynamic computer programming language, is at the core of web development. It’s the tool that adds interactive elements to your website, making it more engaging for users. But as you delve deeper into JavaScript, you may come across some peculiarities, one being the concept of empty strings.
Have you ever wondered how to deal with empty strings in your JavaScript code? Let me assure you, it’s not as difficult as it may initially seem. Together, we’ll learn how to identify and handle these tricky elements, improving your coding skills and making your web applications more robust.
In this post, we will be looking at the following 3 ways to check for empty strings in JavaScript:
- By using the length property
- By using the trim() method
- By comparing with empty quotes
Let’s explore each…
#1 – By using the length property
The length property in JavaScript can be used to check for empty strings. When a string is empty, its length equals zero. By checking if the length of a string equals zero, we can accurately determine whether or not the string is empty. For example, “” is an empty string and its length will be zero.
function isEmpty(str) {
return str.length === 0;
}
console.log(isEmpty("")); // Expected output: true
console.log(isEmpty("Hello")); // Expected output: false
How it works
In this JavaScript code, a function named isEmpty is defined which takes a string str as the parameter. It returns a boolean value indicating whether the string is empty or not. The function checks for emptiness by comparing the length of the string with zero. If the length of the string is zero, it means the string is empty and the function returns true. Otherwise, it returns false.
- First, the function isEmpty is defined which takes a single parameter str which is the string to be checked.
- The length property of the string str is accessed using str.length. The length property of a string in JavaScript gives the number of characters present in the string.
- This length is compared with zero using the === operator. If the length of the string is zero, it means that the string is empty.
- The result of this comparison is a boolean value (true or false) which indicates whether the string is empty or not. This value is returned by the function.
- The function is then called with different strings for testing. An empty string “” returns true indicating it is indeed empty, while a non-empty string like “Hello” returns false indicating that it is not empty.
#2 – By using the trim() method
To check for empty strings in JavaScript using the trim() method, we will write a function that uses this method to remove any white spaces from the beginning and ending of a string, then checks if the resultant string’s length is 0. If it is, this means our input string was empty or contained only whitespaces. Let’s illustrate this with an example:
function isEmptyOrSpaces(str){
return str === null || str.trim().length === 0
}
console.log(isEmptyOrSpaces("")); // returns true
console.log(isEmptyOrSpaces(" ")); // returns true
console.log(isEmptyOrSpaces("Hello")); // returns false
How it works
The trim() method in JavaScript is used to remove white spaces from both ends of a string. If a string is empty or contains only white spaces, using trim() on it will result in an empty string. In JavaScript, an empty string’s length property returns 0. Therefore, by checking if the length of the trimmed string is 0, we can determine if the original string was empty or contained only white spaces.
- The function
isEmptyOrSpaces
is defined with a single parameterstr
, which is the string to check. - The function checks if the
str
is null or if the length of the trimmed string (i.e.,str.trim().length
) is 0. - If either condition is true, the function returns true, indicating that the string is either null, empty, or only contains white spaces.
- Otherwise, the function returns false, indicating that the string contains characters other than white spaces.
#3 – By comparing with empty quotes
To check for empty strings in JavaScript by comparing with empty quotes, you essentially compare the target string with an empty string (”). If it matches, it denotes that the string is empty. Let’s understand this better with a code example.
const str = '';
if (str === '') {
console.log('The string is empty');
} else {
console.log('The string is not empty');
}
How it works
In this piece of code, we’re comparing our string with an empty string using the equality operator ‘===’. If the string is empty (i.e., if it contains no characters), the comparison will be true and we will see ‘The string is empty’ in the console. If the string contains any characters, even a space, the comparison will be false and we will see ‘The string is not empty’ in the console.
- Step 1: We initialize a constant ‘str’ with an empty string.
- Step 2: We compare ‘str’ with an empty string using the equality operator ‘===’.
- Step 3: If the comparison evaluates to true (i.e., if ‘str’ is indeed an empty string), we log ‘The string is empty’ to the console.
- Step 4: If the comparison evaluates to false (i.e., if ‘str’ contains any characters), we log ‘The string is not empty’ to the console.
Related:
- How to Convert Timestamps to Dates in JavaScript
- How to Convert CSV to Array in JavaScript
- How to Check for an Empty String in JavaScript
In conclusion, checking for empty strings in JavaScript is a relatively simple procedure. Use either the traditional “if” conditional statement, the trim() method, or the length property to fulfill your needs.
Remember, empty strings can impact your code’s execution, so it’s crucial to handle them properly. With practice, you’ll become adept at checking and managing such cases in JavaScript.