How to Remove First Character from a String in JavaScript
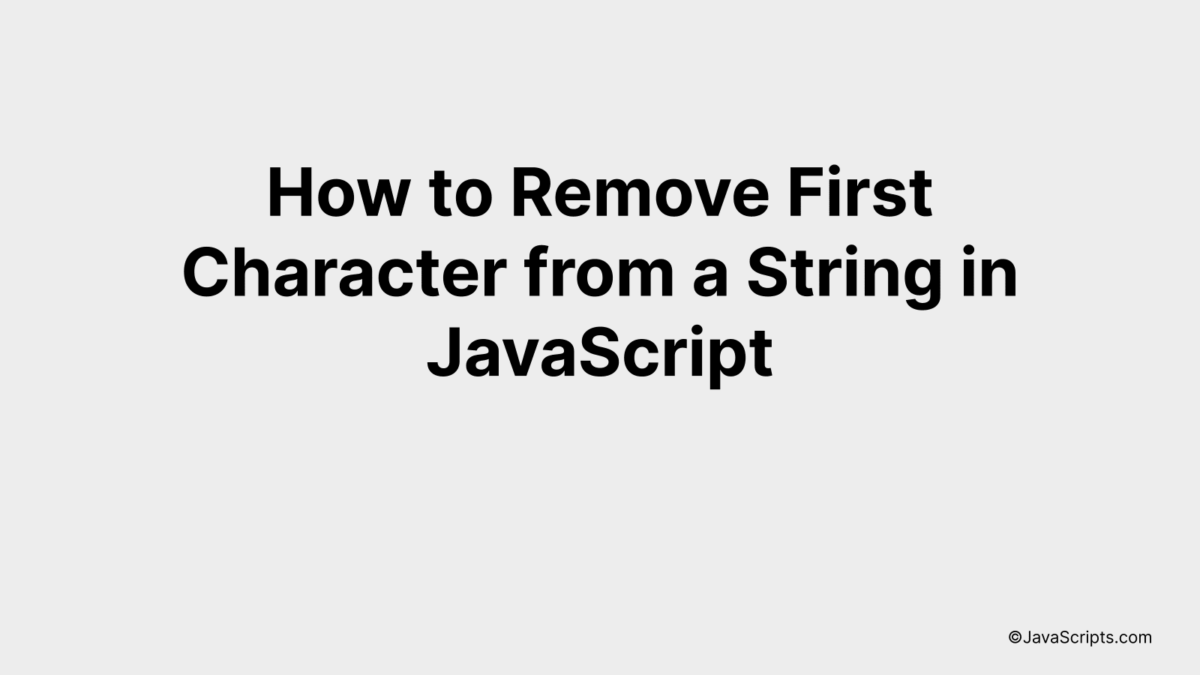
Manipulating text data is a common occurrence when programming, and JavaScript is no exception. Our primary focus today is on how you can efficiently remove the first character from a string using this versatile language.
Does your project involve string manipulation? Or perhaps, do you have strings where you need to get rid of the initial character? This guide will assist you in simplifying such tasks, making your coding journey smoother and more efficient.
In this post, we will be looking at the following 3 ways to remove first character from a string in JavaScript:
- By slice() method
- By substring() method
- By using array destructuring and join() method
Let’s explore each…
#1 – By slice() method
The slice() method extracts a section of a string and returns it as a new string. In our case, we use it to remove the first character by starting the slice from the second character (index 1). For instance, if we have a string “Hello”, after applying the method, we will get “ello”.
var str = "Hello";
var newStr = str.slice(1);
How it works
The slice() method in JavaScript takes two parameters: start and end index. In our case, we only provide the start index, 1. This tells JavaScript to start slicing from the second character and go all the way to the end of the string. The first character is therefore excluded.
- First, we declare a variable ‘str’ and assign it the string “Hello”.
- Next, we call the slice() method on ‘str’ with the starting index set as 1, meaning we want to start our new string from the second character of ‘str’.
- The slice() method then returns a new string starting from the second character to the end of ‘str’. This new string is stored in another variable ‘newStr’.
- As a result, ‘newStr’ now holds the string “ello”, which is the original string “Hello” but without the first character.
#2 – By substring() method
The substring() method in JavaScript can be used to remove the first character from a string by specifying the starting index as 1. This will return a new string from the original string starting from the second character up to the end.
Let’s consider an example: If we start with the string “Hello, World!” and want to remove the first character, the resulting string should be “ello, World!”. We can achieve this using the substring() method as follows:
var str = "Hello, World!";
var newStr = str.substring(1);
How it works
The substring() method in JavaScript enables manipulation of string data. In this case, it is used to remove the first character of a string. Here’s how this works:
- First, a variable ‘str’ is defined with the original string “Hello, World!”.
- Then, a new variable ‘newStr’ is defined. The substring() method is called on the original string ‘str’ with argument ‘1’.
- The argument ‘1’ with substring() method represents the starting index for the new string. As JavaScript indexes start from ‘0’, ‘1’ here means that the new string should start from the second character of the original string.
- Thus, the first character of the original string is effectively removed in the new string ‘newStr’.
Therefore, by using the substring() method in JavaScript, we can easily remove the first character from a string.
#3 – By using array destructuring and join() method
In JavaScript, we can remove the first character from a string using array destructuring and the join() method. Array destructuring allows us to separate the first character and the rest of the string, and join() is then used to merge the rest of the string back together.
let str = "Hello World";
let [, ...chars] = str;
let result = chars.join('');
How it works
In JavaScript, we are able to use an interesting feature known as array destructuring. This feature allows us to unpack items from arrays or properties from objects into distinct variables. In our case, we are using array destructuring to separate the first character from the rest of the string. We then use the ‘join()’ method to combine these remaining characters back into a single string, effectively removing the first character from our original string.
Let’s break this down:
- First, we declare the string from which we want to remove the first character: let str = “Hello World”;
- Next, we use array destructuring to separate the first character from the rest of the string: let [, …chars] = str;. Here, the , skips the first character, and …chars collects the rest of the characters into an array.
- Finally, we use the join(”) method, which combines all the elements of the chars array into a string, effectively creating a new string without the first character: let result = chars.join(”);
Related:
- How to Print Arrays in JavaScript
- How to Perform String Empty Check in JavaScript
- How to Perform Date Subtraction in JavaScript
In conclusion, working with strings in JavaScript is not as daunting as you may think. A simple method like substring() can easily help you remove the first character from any string. It does this by creating a new string from your original one, starting from the second character.
Understanding these basic string manipulation methods can unlock a lot of JavaScript’s potential. So, keep practicing and experimenting with different methods to enhance your coding skills. After all, practice makes perfect!