How to Split an Array into Chunks in JavaScript
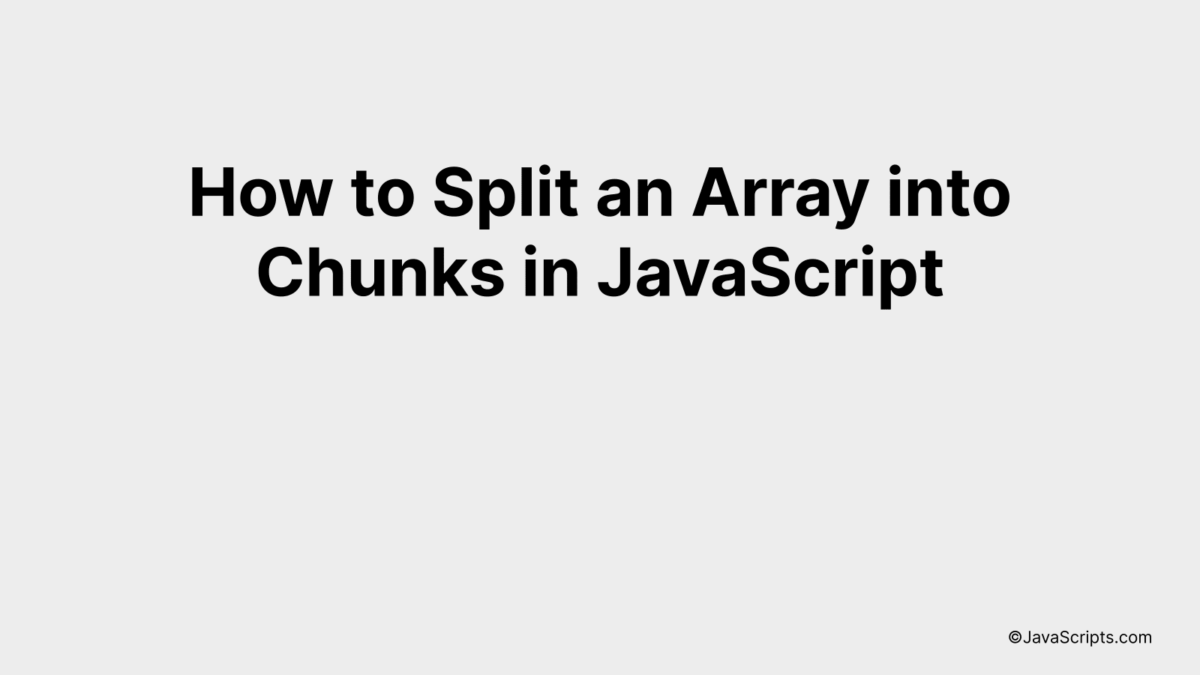
Are you trying to break down large data sets into manageable chunks in JavaScript? Don’t worry, you’re not alone. Many programmers face similar challenges when working with large arrays.
Let’s delve into an efficient method that can help you split an array into smaller chunks. You and I will unravel the magic of JavaScript together, making your code more readable and your processing tasks easier to handle.
In this post, we will be looking at the following 3 ways to split an array into chunks in JavaScript:
- By using the slice() method
- By using the splice() method
- By using a custom function with a loop
Let’s explore each…
#1 – By using the slice() method
We’re going to create a function in JavaScript that will split an array into chunks of smaller arrays using the slice() method. As an example, if we have an array of numbers from 1 to 10 and we want to split it into smaller arrays of 3 elements each, our function will help us do that.
function chunkArray(array, chunkSize) {
let chunks = [];
for (let i = 0; i < array.length; i += chunkSize) {
chunks.push(array.slice(i, i + chunkSize));
}
return chunks;
}
// example usage
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
let chunked = chunkArray(numbers, 3);
console.log(chunked); // outputs: [[1, 2, 3], [4, 5, 6], [7, 8, 9], [10]]
How it works
Our function takes an array and a chunk size as input. It then iterates over the array using a for loop, where the increment value is the chunk size we want. In each iteration, it uses the slice() method to get a "chunk" of the array from the current index up to the current index plus the chunk size, and adds it to a new array. This process continues until the end of the array, and finally, the function returns the new array containing all the chunks.
- Step 1: The function receives the array and chunk size as its parameters.
- Step 2: It creates an empty array, 'chunks', which will be used to store the chunks of the original array.
- Step 3: Using a for loop, the function starts iterating over the input array. The loop increment is the chunk size, meaning at each iteration it will jump forward by 'chunkSize' indices.
- Step 4: Inside the loop, the slice() method is used to get a chunk from the current index to the current index plus the chunk size, and this chunk is then added to the 'chunks' array.
- Step 5: This process continues until all elements in the original array have been processed, and 'chunks' now contains all the chunks.
- Step 6: Finally, the function returns the 'chunks' array, which now contains all the chunks of the original array.
#2 - By using the splice() method
We are going to create a function in JavaScript that will split an array into smaller arrays (chunks) using the splice() method. Every chunk will have a specified maximum size. For example, if we have an array [1, 2, 3, 4, 5] and we want to split it into chunks of size 2, the result will be [[1, 2], [3, 4], [5]].
function splitIntoChunks(array, chunkSize) {
let chunks = [];
while (array.length) {
chunks.push(array.splice(0, chunkSize));
}
return chunks;
}
How it works
This function works by continuously taking the first 'chunkSize' elements from the original array and pushing them into a new array. This process is repeated until the original array is empty.
- Firstly, an empty array 'chunks' is created to store all the split chunks.
- Then, a while loop is initiated which runs until the original array becomes empty.
- In each iteration, the 'splice' method is called on the array which removes the first 'chunkSize' elements from the array and returns them. The returned chunk is then pushed into the 'chunks' array.
- Finally, when the original array is empty, the while loop ends and the 'chunks' array is returned which contains the original array split into chunks of 'chunkSize'.
#3 - By using a custom function with a loop
This process involves creating a JavaScript function that utilizes a while loop to segment a given array into subarrays (or chunks) of a specified length. As an example, given an array [1,2,3,4,5,6,7,8,9] and a chunk size of 3, the output will be [[1,2,3],[4,5,6],[7,8,9]].
function chunkArray(array, chunkSize) {
let results = [];
while (array.length) {
results.push(array.splice(0, chunkSize));
}
return results;
}
How it works
This function works by continuously splicing chunks from the original array until it's empty, and pushing these chunks into a new 'results' array.
- An empty array
results
is declared to store the chunked arrays. - A while loop is set to run as long as the length of the initial array is not 0.
- Within the loop, the JavaScript
splice
method is used to removechunkSize
elements from the start of the array and push them into theresults
array. - The
splice
method modifies the original array and the loop continues until all elements have been chunked. - Finally, the function returns the
results
array containing the chunked arrays.
Related:
- How to Set Background Color with JavaScript
- How to Replace DIV Content with JavaScript
- How to Remove Substrings from Strings in JavaScript
In conclusion, splitting an array into chunks in JavaScript is a valuable technique for managing data effectively. It's a simple but powerful method that proves useful in many real-world programming situations.
Remember, practice and exploration are key to mastering this skill. Keep coding, keep exploring, and you'll find this technique becoming second nature to you.