How to Calculate Average of an Array in JavaScript
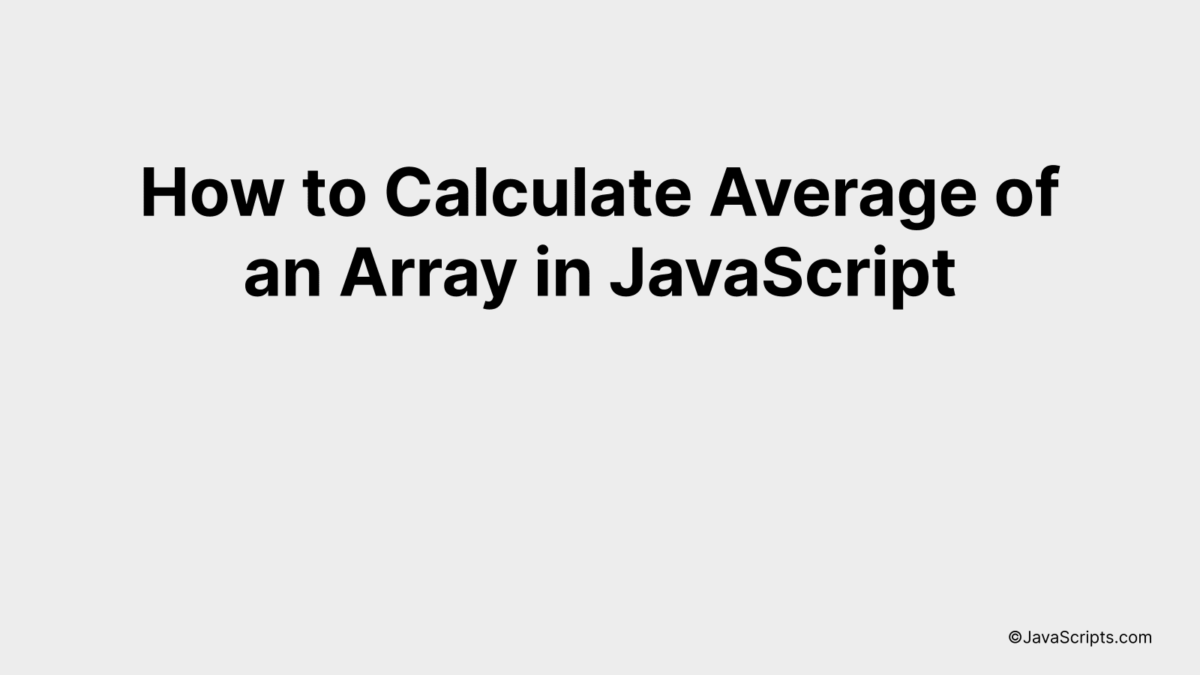
Understanding the fundamentals of a programming language is essential, especially when it comes to JavaScript. One such fundamental concept is calculating the average of an array, a common task that can be accomplished in various ways.
We’ll delve into the world of arrays and arithmetic in JavaScript, focusing particularly on one task – determining the average of numbers in an array. You might wonder, how complex can this task get? You’d be surprised at the flexibility JavaScript offers for such a seemingly simple task. Let’s explore together!
In this post, we will be looking at the following 3 ways to calculate average of an array in JavaScript:
- By using the for loop method
- By using the reduce() method
- By using the forEach() method
Let’s explore each…
#1 – By using the for loop method
We will calculate the average of an array in JavaScript using a for loop. This process involves summing all elements of the array and then dividing the total by the length of the array. For instance, if we have an array [1, 2, 3, 4, 5], the average would be calculated by summing all the numbers (1+2+3+4+5 = 15) and then dividing by the total quantity of numbers (5), giving us an average of 3.
var arr = [1, 2, 3, 4, 5];
var sum = 0;
for (var i = 0; i < arr.length; i++) {
sum += arr[i];
}
var avg = sum / arr.length;
console.log(avg);
How it works
Using a for loop, we're iterating over each element in our array, adding it to our sum. After iterating through all elements, we find the average by dividing the sum by the total number of elements in the array.
- First, we declare the array we are going to calculate the average of.
- Secondly, we initialize a variable 'sum' to 0. We will use this variable to store the sum of all the elements of the array.
- Then, we use a for loop to iterate over each element in the array. Within the for loop, we add each array element to the 'sum' variable.
- After the for loop completes its execution, we have the total sum of all the array elements in the 'sum' variable.
- Finally, we calculate the average by dividing the calculated sum by the length of the array (i.e., the total count of numbers) and store this value in the 'avg' variable.
- And at the end, we log it onto the console to check the calculated average value.
#2 - By using the reduce() method
The reduce() method in JavaScript can be used to calculate the average of an array by cumulatively adding each element in the array and then dividing the sum by the total number of elements. This concept will be easier to understand with an example.
let numbers = [1, 2, 3, 4, 5];
let average = numbers.reduce((total, num, index, array) => {
total += num;
if( index === array.length-1) {
return total/array.length;
} else {
return total;
}
}, 0);
How it works
In the above code, the reduce() method is used to calculate the sum of all numbers in the array and then find the average. The reduce() method takes a reducer function as its first parameter and an initial value as its second parameter.
- The reducer function itself accepts four parameters - the accumulator (total), the current value (num), the current index (index), and the original array (array).
- For each element in the array, the current value (num) is added to the accumulator (total).
- If we are at the last element of the array (checked by 'index === array.length-1'), the total is divided by the length of the array to find the average.
- If we are not at the last element, the total (with the current value added) is returned to be used as the accumulator value in the next iteration.
- The reduce() method returns the final value of the accumulator, which in this case, will be the average of all numbers in the array.
#3 - By using the forEach() method
In JavaScript, you can calculate the average of an array using the forEach() method by summing up all the values in the array and then dividing the sum by the length of the array. For example, if you have an array [1, 2, 3, 4, 5], you can find the average by adding up all the numbers (1+2+3+4+5 = 15) and then dividing by the total count of numbers (15 / 5 = 3).
var arr = [1, 2, 3, 4, 5];
var sum = 0;
arr.forEach(function(value) {
sum += value;
});
var average = sum / arr.length;
How it works
The forEach() method in JavaScript is a built-in array method that executes a provided function once for each array element. In the above code, we leverage this method to calculate the sum of all the elements in the array. We then divide this sum by the length of the array (the number of elements) to get the average.
- First, an array 'arr' and a variable 'sum' are declared. The array contains the numbers of which we want to find the average and 'sum' is initialized to 0.
- Then, the forEach() method is called on the array. For each element in the array, a function is executed which adds the current value to 'sum'.
- By the end of the forEach() method, 'sum' contains the total of all the elements in the array.
- Finally, we calculate the average by dividing the 'sum' with the length of the array (the total number of elements).
Related:
- How to Add Months to Dates in JavaScript
- How to Access Object Properties in JavaScript
- How to Zero Pad Numbers in JavaScript
In conclusion, calculating the average of an array in JavaScript is a straightforward process. With a basic understanding of array methods like 'reduce', you can easily sum all the elements of an array and find the average by dividing it by the total number of elements.
So, keep practicing with different arrays and build your confidence. Remember, the more you code, the better your understanding of JavaScript becomes. Happy coding!