How to Access Object Properties in JavaScript
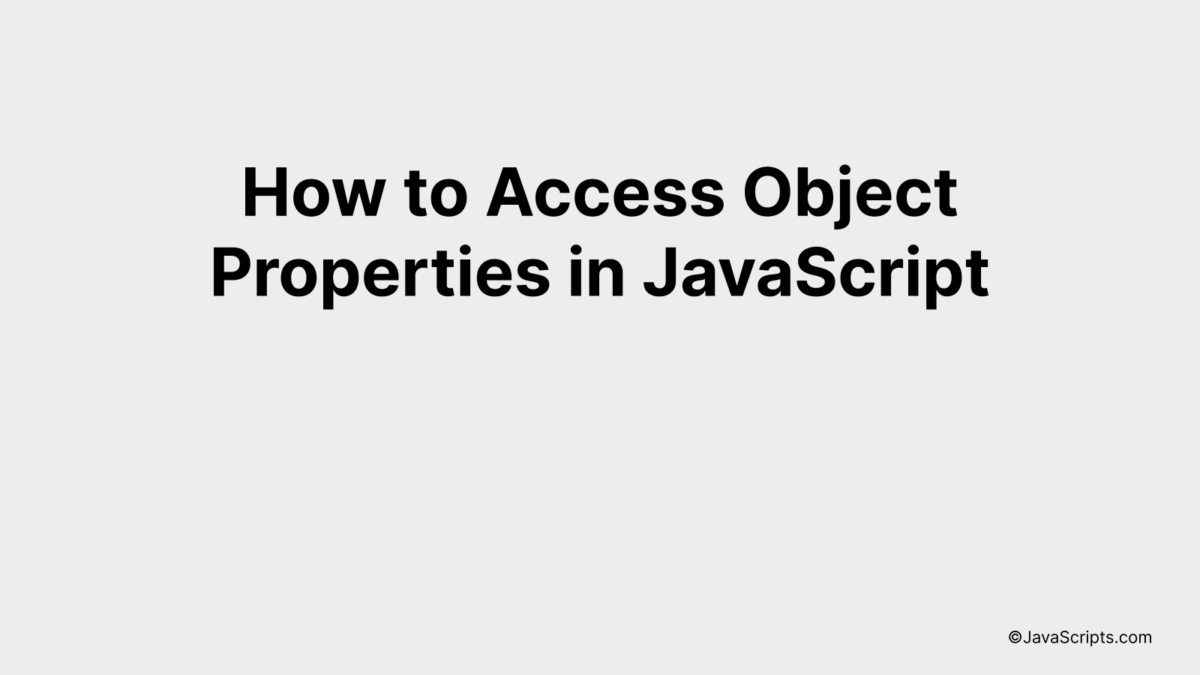
Navigating the world of JavaScript can seem like a daunting task, especially when you’re trying to understand how objects work. Trust me, once you get the hang of it, you’ll realize it’s not as complex as it seems.
Let’s break down the process of accessing object properties in JavaScript together. I’m here to guide you through the basics and demonstrate it in the most uncomplicated way possible. By the end, you’ll have a solid foundation to build upon as you continue to explore JavaScript.
In this post, we will be looking at the following 3 ways to access object properties in JavaScript:
- By Dot notation
- By Bracket notation
- By using the Object’s getOwnPropertyDescriptor method
Let’s explore each…
#1 – By Dot notation
In JavaScript, you can access an object’s properties using dot notation, where you simply place a dot after the object’s name followed by the property name. This allows you to retrieve or modify the value associated with that property. For example, if you have an object ‘person’ with a property ‘name’, you can access it as ‘person.name’.
var person = {
name: 'John Doe',
age: 30
};
console.log(person.name); // Outputs: John Doe
How it works
In the above code, we use dot notation to access the property ‘name’ of the object ‘person’. The dot notation provides a simple and readable way to retrieve or modify the value of a property within an object.
- We first create an object ‘person’ with properties ‘name’ and ‘age’ and assign it to the variable ‘person’.
- Next, we use dot notation (‘person.name’) to access the ‘name’ property of the ‘person’ object.
- The console.log statement prints the value of ‘person.name’ to the console, which is ‘John Doe’.
#2 – By Bracket notation
In JavaScript, bracket notation is a way to access object properties by using an expression or a variable inside square brackets. For instance, if we have an object named ‘student’ with a property ‘name’, we can access it as student[‘name’].
var student = {
name: 'John',
age: 15
};
var property = 'name';
console.log(student[property]); // Outputs: John
How it works
In the provided code, we create an object ‘student’ with properties ‘name’ and ‘age’. Then we create a variable ‘property’ to store the property we want to access, in this case ‘name’. Using bracket notation, we are then able to access the ‘name’ property of the ‘student’ object by using the ‘property’ variable.
- An object ‘student’ is created with properties ‘name’ and ‘age’.
- A variable ‘property’ is then declared and assigned the value ‘name’.
- To access the ‘name’ property of the ‘student’ object, we use bracket notation: ‘student[property]’.
- Since the value of ‘property’ is ‘name’, ‘student[property]’ is equivalent to ‘student[“name”]’ which returns the value of the ‘name’ property, in this case ‘John’.
- The value ‘John’ is then logged to the console.
#3 – By using the Object’s getOwnPropertyDescriptor method
The Object.getOwnPropertyDescriptor() method in JavaScript allows you to access the properties of an object and get details about them, including their value, if they’re writable, enumerable, or configurable. We’ll demonstrate this with a simple example using an object that has properties.
let person = {
name: "John",
age: 30
};
let descriptor = Object.getOwnPropertyDescriptor(person, 'name');
console.log(descriptor);
How it works
This method, Object.getOwnPropertyDescriptor(), accepts two arguments – the object you want to inspect, and the property name you want details about. It returns an object that contains the property attributes.
Here’s a step-by-step guide as to how this works:
- First, we create an object person with properties name and age.
- Then, we use Object.getOwnPropertyDescriptor(person, ‘name’) to get the descriptor of the property ‘name’.
- This method returns an object with details about the ‘name’ property.
- The returned object contains properties like value (the value of the property), writable (if the property can be changed), enumerable (if the property is visible during enumeration), and configurable (if the property can be deleted or its attributes changed).
- Finally, we log the descriptor object to the console to see the details.
Related:
- How to Zero Pad Numbers in JavaScript
- How to Wait for Elements to Exist in JavaScript
- How to Use a Variable as a Key in JavaScript
In conclusion, accessing object properties in JavaScript is pretty straightforward once you understand the basics. There are two main ways to do this: using dot notation or bracket notation. It’s all about understanding and using these two methods effectively.
Always remember, consistent practice is the key to mastering JavaScript. So, keep experimenting with these methods and in no time, you’ll find it second nature to access object properties in JavaScript. Happy coding!