How to Iterate over Key-Value Pairs in JavaScript
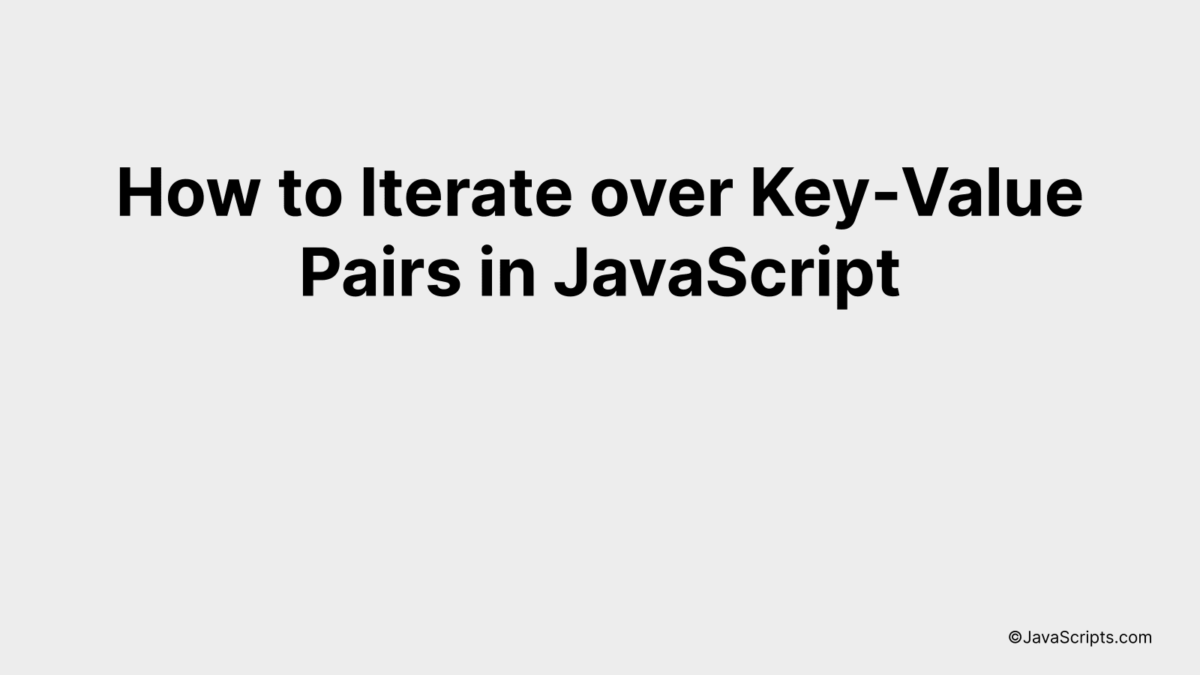
Navigating through JavaScript often requires working with objects, and more specifically, key-value pairs. You may find yourself needing to loop through these pairs, uncovering each key and its corresponding value. It’s an essential skill in the realm of web development and programming at large.
But how exactly do you go about this? We’ll answer that question together. We’ll take a simple, step-by-step approach to understanding how to iterate over key-value pairs in JavaScript, unraveling the techniques and code constructions that make this process straightforward and efficient.
In this post, we will be looking at the following 3 ways to iterate over key-value pairs in JavaScript:
- By using for…in loop
- By using Object.keys() with forEach()
- By using for…of loop with Object.entries()
Let’s explore each…
#1 – By using for…in loop
The for…in loop in JavaScript allows you to iterate over the properties, which are both keys and values, of an object. It is an efficient way to examine every property in an object and carry out operations. Let’s understand this with a code example.
let obj = {
name: "John",
age: 30,
city: "New York"
};
for (let key in obj) {
console.log(Key: ${key} - Value: ${obj[key]});
}
How it works
The for…in loop goes through the properties of the object, each time assigning the property’s key to the variable (in this case ‘key’), which you can then use in the block of code inside the loop. The value of each property can be accessed by using the key with the object.
- The loop starts with the ‘for’ keyword, followed by a set of parentheses. Inside these parentheses, you declare a variable (here, ‘key’) to hold the current key during each iteration.
- Following the ‘in’ keyword, you place the object over whose properties you want to iterate (here, ‘obj’).
- Within the loop, you can access the key of the current property (stored in ‘key’) and its corresponding value (retrieved with ‘obj[key]’).
- The specific operation within the loop (here, logging the key-value pair to the console) happens for each property in the object.
- The loop stops iterating once it has gone through all the properties in the object.
#2 – By using Object.keys() with forEach()
Object.keys() method in JavaScript is used to get an array containing all the keys present in the object, and forEach() is a method that allows you to iterate through this array, thus helping you to iterate key-value pairs in the object. Let’s understand this by using a code example.
let obj = {
"firstName": "John",
"lastName": "Doe",
"age": 30
};
Object.keys(obj).forEach((key) => {
console.log(Key: ${key} and Value: ${obj[key]});
});
How it Works
The Object.keys(obj) method is used to retrieve an array of all the keys in the object. The forEach() method then iterates over this array and for each key, it logs the key and corresponding value to the console.
- First, we create an object
obj
with some key-value pairs. - Then, we use the
Object.keys(obj)
method to return an array that contains all keys present in our object. - We then use the
forEach()
method on the array returned byObject.keys()
. ThisforEach()
method executes the provided function once for each array element. - Inside the
forEach()
method, we have a callback function that takes a key as a parameter. This key corresponds to each key in our object. - Finally, inside the callback function, we use console.log to print each key and its corresponding value.
#3 – By using for…of loop with Object.entries()
The ‘for…of’ loop in JavaScript combined with ‘Object.entries()’ allows you to iterate over an object’s key-value pairs. Let’s comprehend it with an example.
let student = {
'name': 'John Doe',
'age': 22,
'department': 'Computer Science'
};
for (let [key, value] of Object.entries(student)) {
console.log(${key}: ${value});
}
How it works
The ‘Object.entries()’ method returns an array of a given object’s own string-keyed property [key, value] pairs. This array can be iterated over with a ‘for…of’ loop.
- Step 1: An object named ‘student’ is created with properties ‘name’, ‘age’, and ‘department’.
- Step 2: ‘Object.entries(student)’ is called. This method returns an array of the object’s own property pairs, i.e., [[‘name’, ‘John Doe’], [‘age’, 22], [‘department’, ‘Computer Science’]].
- Step 3: The ‘for…of’ loop is used to iterate over each property pair from the array returned from ‘Object.entries(student)’.
- Step 4: Inside the ‘for…of’ loop, each property pair is destructured into ‘key’ and ‘value’ variables. The loop prints each key-value pair to the console.
Related:
- How to Get Viewport Width in JavaScript
- How to Get the Domain from a URL in JavaScript
- How to Generate Random Numbers Between Two Numbers in JavaScript
In conclusion, iterating over key-value pairs in JavaScript is a fundamental skill that every coder should master. This understanding facilitates efficient coding, enabling you to work with objects and arrays more effectively.
Remember, methods like ‘for…in’, ‘Object.keys()’, ‘Object.values()’, and ‘Object.entries()’ are your best tools for this task. Keep practicing, and in no time, traversing key-value pairs in JavaScript will become second nature to you.