How to Check if a Property Exists in JavaScript
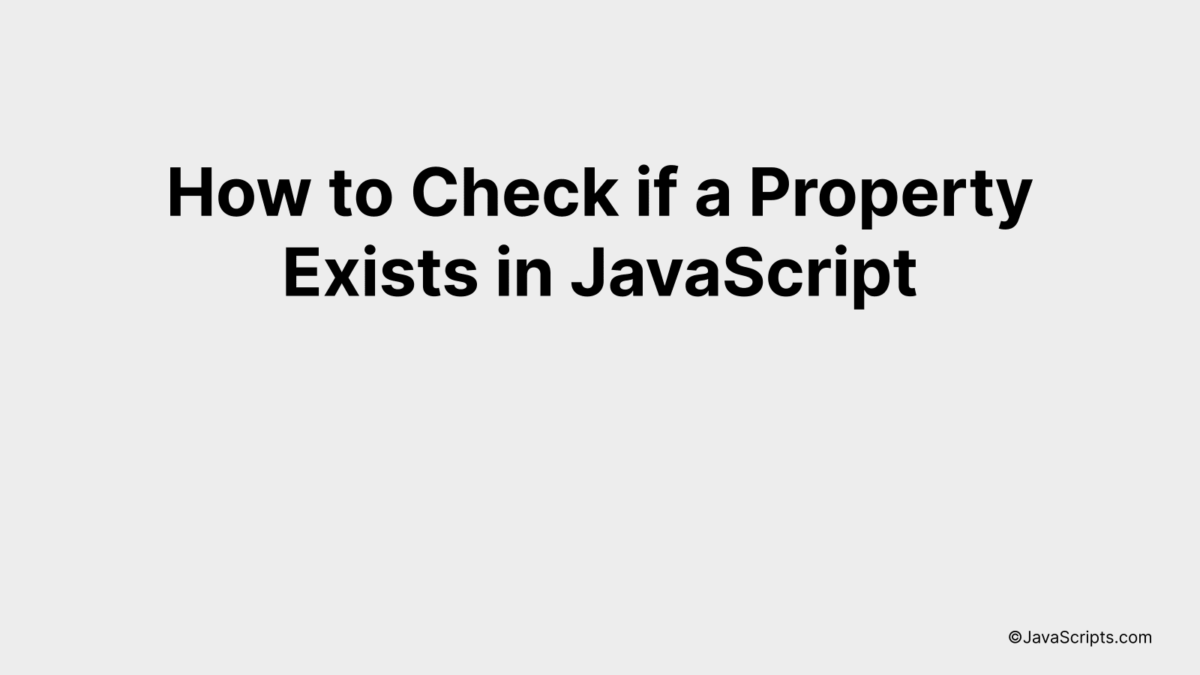
As you delve deeper into the world of JavaScript, you and I will often encounter situations where we need to confirm if a particular property exists in an object. You may be wondering why it’s important. Well, it’s crucial for error prevention and smooth execution of code, especially when dealing with complex objects or APIs.
Let’s make this journey together, as we learn how to verify the existence of a property in a JavaScript object. We’ll keep things simple and straightforward, breaking down the concepts into digestible bits, ensuring you grasp the essence of each method we discuss.
In this post, we will be looking at the following 3 ways to check if a property exists in JavaScript:
- By using the “in” operator
- By using the “hasOwnProperty()” method
- By checking if the value of the property is “undefined”
Let’s explore each…
#1 – By using the “in” operator
The “in” operator in JavaScript is used to check if a property exists in an object. It returns true if the property is in the object, and false otherwise. For example, if we have an object named “person” with a property “name”, we can check if “name” exists in “person” by using “name” in person.
let person = { name: 'John', age: 25 };
let result = 'name' in person;
console.log(result); // prints 'true'
How it works
The “in” operator checks for property existence within an object in JavaScript. In the context of the provided code, it is checking if the property “name” exists within the object “person”.
- First, an object “person” is created with properties “name” and “age”.
- Then, the “in” operator is used to check if the property “name” exists in the object “person”. The syntax here is ‘propertyName’ in object. If the property exists, the operator returns true; otherwise, it returns false.
- Since “name” is a property of “person”, ‘name’ in person returns true.
- Then, this result is logged to the console using console.log(). As expected, it prints ‘true’.
#2 – By using the “hasOwnProperty()” method
The “hasOwnProperty()” method is used in JavaScript to check if a specific property exists within an object. This method returns a boolean indicating whether the object has the specified property as its own property (as opposed to inheriting it). Here’s an example to understand it better.
let vehicle = {
brand: 'Toyota',
model: 'Corolla',
year: 2020
};
let result = vehicle.hasOwnProperty('brand'); // It will return true
How it works
In our code, we have an object ‘vehicle’ with properties ‘brand’, ‘model’, and ‘year’. We use the ‘hasOwnProperty()’ method on this object to check if it contains a property ‘brand’.
- First, we create an object ‘vehicle’ with properties ‘brand’, ‘model’, and ‘year’.
- Then, we use the ‘hasOwnProperty()’ method on this object by specifying the name of the property we are looking for. In this case, ‘brand’.
- The ‘hasOwnProperty()’ method then checks if this property exists as an own (not inherited) property of ‘vehicle’.
- If the property exists, the method returns true. If it doesn’t, the method returns false.
- In our case, since ‘brand’ is a property of ‘vehicle’, the ‘hasOwnProperty()’ method returns true.
#3 – By checking if the value of the property is “undefined”
In JavaScript, you can check if a property exists in an object by comparing the value of the property to “undefined”. If the property does not exist, its value will be “undefined”. This can be demonstrated with an example.
const obj = { prop1: 'value1', prop2: 'value2' };
if(obj.prop3 === undefined) {
console.log('prop3 does not exist');
} else {
console.log('prop3 exists');
}
How it works
In JavaScript, when you try to access a property that does not exist in an object, it returns “undefined”. So, when the value of a property is checked against undefined, and if it matches, it means that the property does not exist.
- First, we create an object ‘obj’ with properties ‘prop1’ and ‘prop2’.
- Then, we check if ‘prop3’ exists in ‘obj’ by comparing the value of ‘obj.prop3’ to undefined.
- If ‘obj.prop3’ is ‘undefined’, it means ‘prop3’ does not exist in ‘obj’, and the message ‘prop3 does not exist’ will be logged to the console.
- Otherwise, if ‘obj.prop3’ is not ‘undefined’, it means ‘prop3’ exists in ‘obj’, and the message ‘prop3 exists’ will be logged to the console.
Related:
- How to Add Properties to Objects in JavaScript
- How to Wrap Elements with JavaScript
- How to Sort Maps in JavaScript
In conclusion, checking if a property exists in JavaScript is a simple but crucial concept. It equips you with the ability to manage your code more effectively, avoiding potential issues that could arise from accessing non-existent properties.
There are several methods to do this, including using ‘in’ operator, ‘hasOwnProperty’ method, or simply checking if the property value is ‘undefined’. Remember, practice is key in mastering these techniques, so don’t hesitate to experiment and hone your JavaScript skills.