How to Clone Arrays in JavaScript
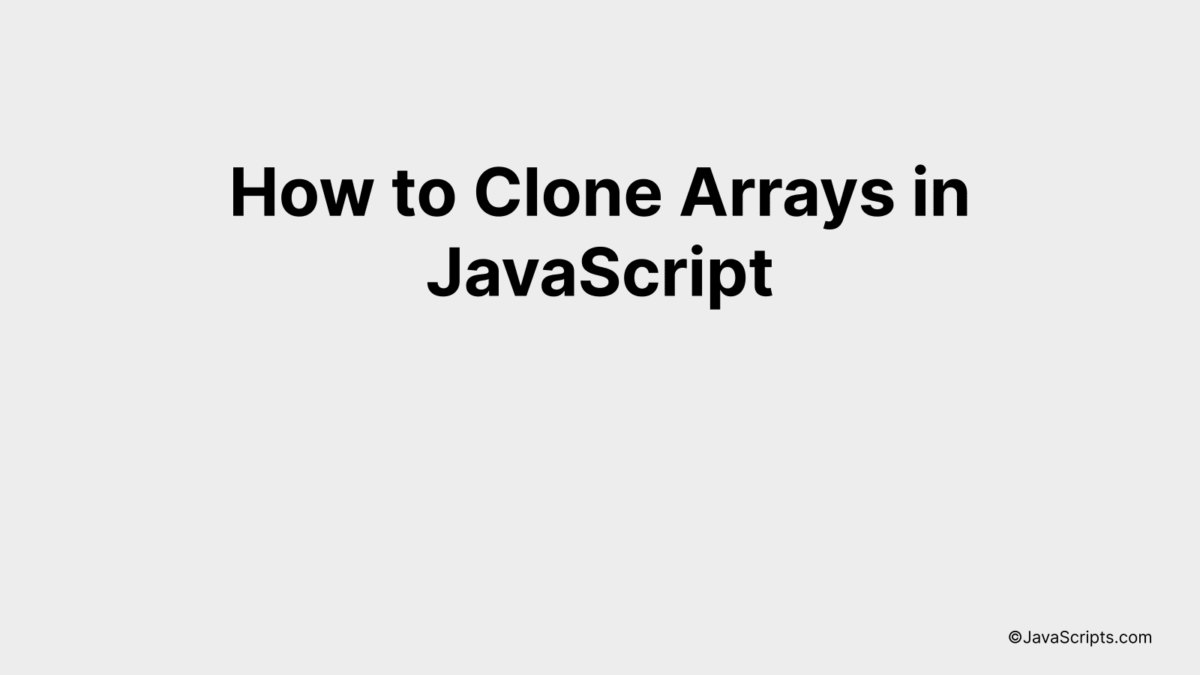
Cloning arrays, you ask? Well, JavaScript is known for its array manipulation capabilities, making it a powerful tool for web development. But sometimes, we may need to duplicate an array without affecting the original, a process known as cloning.
Why would you want to clone an array? Imagine working with a large set of data where you want to experiment with sorting or filtering, but need the original data to remain untouched. That’s where cloning comes to our rescue. Let’s delve into this concept and learn how to effectively clone arrays using JavaScript.
In this post, we will be looking at the following 3 ways to clone arrays in JavaScript:
- By using the spread operator […]
- By using the Array.from() method
- By using the slice() method
Let’s explore each…
#1 – By using the spread operator […]
The spread operator allows us to make a copy of an array by spreading the values of the original array into a new one; this is handy when you need a duplicate array without referencing the original one. Here’s an example:
let originalArray = [1, 2, 3, 4, 5];
let clonedArray = [...originalArray];
How it works
The spread operator essentially takes the values from the original array and puts them into a new array. Because a new array is created, changes in the cloned array do not affect the original array.
- Step 1: Declare a variable named ‘originalArray’ and assign it an array of numbers.
- Step 2: Declare another variable, ‘clonedArray’. To clone ‘originalArray’, we use the spread operator inside a new array declaration. It spreads the elements of ‘originalArray’ into ‘clonedArray’.
- Step 3: Now ‘clonedArray’ is a copy of ‘originalArray’. Any changes to ‘clonedArray’ won’t affect ‘originalArray’ because they are now two separate arrays.
#2 – By using the Array.from() method
The Array.from() method in JavaScript is used to clone an array. Here’s a simple example for better understanding:
const arr1 = [1, 2, 3, 4];
const arr2 = Array.from(arr1);
How it works
The Array.from() function creates a new, shallow-copied array instance from an array-like or iterable object. In this case, we are creating a clone of ‘arr1’ and assigning it to ‘arr2’.
- First, we declare an array ‘arr1’ with the elements 1, 2, 3, and 4.
- Then we use Array.from() to create a new array ‘arr2’ that is a clone of ‘arr1’.
- The Array.from() function iterates over ‘arr1’ and assigns each value to a new array, ‘arr2’.
- Finally, ‘arr2’ is a separate instance and any changes to ‘arr1’ will not impact ‘arr2’, and vice versa.
#3 – By using the slice() method
The slice() method can be used to clone an array in JavaScript by creating a new array and copying all elements of the original array to it without affecting the original one. For a better understanding, let’s consider an example.
let originalArray = [1, 2, 3, 4, 5];
let clonedArray = originalArray.slice();
How it works
The slice() method returns a shallow copy of a portion of an array into a new array object without modifying the original array. In this case, no start/end index is provided, so it copies the whole array.
- First, an array named ‘originalArray’ is defined with the values [1, 2, 3, 4, 5].
- Next, the slice() method is called on ‘originalArray’ without passing any arguments, which means it’s going to copy the entire array.
- As the slice() method does not mutate the original array but creates a new one, ‘clonedArray’ is a new array with the same elements as ‘originalArray’.
- As a result, you now have ‘clonedArray’ which is a clone of ‘originalArray’.
Related:
- How to Check if a Property Exists in JavaScript
- How to Add Properties to Objects in JavaScript
- How to Wrap Elements with JavaScript
In conclusion, cloning arrays in JavaScript can be achieved through a variety of ways, from the simple slice method to the more advanced spread operator and Array.from method. It’s all about choosing the right method that suits your specific requirements best.
Remember, while it may seem trivial, proper array cloning is vital to prevent unexpected mutations in your code. By cloning arrays, we can ensure changes to the new array won’t affect the original one, enabling more secure, efficient, and cleaner code.