How to Convert Timestamps to Dates in JavaScript
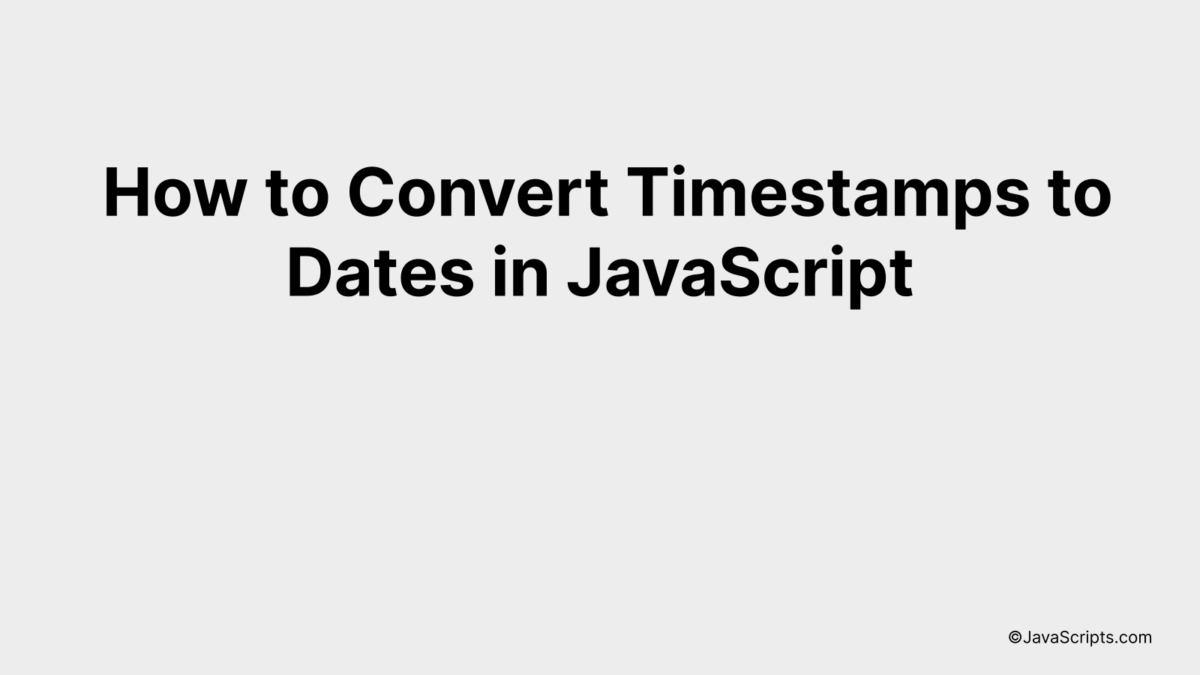
Have you ever found yourself wrestling with timestamps, wanting to extract meaningful dates from a string of number-filled codes? Don’t worry, you aren’t alone, and there’s a straightforward solution to this common problem. JavaScript, one of the most widely used programming languages, can be our trusty tool to accomplish this task.
The world of web development often requires us to handle timestamps, and understanding how to convert them into human-readable dates is key. Whether you’re a seasoned programmer or a beginner, this straightforward guide will help you decode timestamp data with ease and efficiency.
In this post, we will be looking at the following 3 ways to convert timestamps to dates in JavaScript:
- By using the Date constructor
- By using the toLocaleDateString() method
- By using Moment.js library
Let’s explore each…
#1 – By using the Date constructor
The JavaScript Date constructor can be used to convert a timestamp (in milliseconds) to a human-readable date format. As an example, consider a timestamp ‘1617988557000’, we can convert this timestamp into a date format like ‘2021-04-09T09:42:37.000Z’
var timestamp = 1617988557000; //sample timestamp in milliseconds
var date = new Date(timestamp); //converting timestamp to date
console.log(date.toISOString()); //logging the date in 'YYYY-MM-DDTHH:MM:SS.sssZ' format
How it works
This code snippet works by first defining a timestamp. It then uses the JavaScript Date constructor to convert this timestamp into a date object. Finally, it uses the built-in method ‘toISOString()’ to convert this date object into a readable string format ‘YYYY-MM-DDTHH:MM:SS.sssZ’.
- The JavaScript Date constructor takes a timestamp as an argument and returns a Date object. In the provided code, we are passing ‘timestamp’ as an argument which represents the number of milliseconds since the Unix Epoch (January 1, 1970, 00:00:00 UTC).
- This Date object represents a single moment in time, which can be retrieved using various built-in methods. Here, ‘toISOString()’ method is used which returns a string in simplified extended ISO format (ISO 8601), which is always 24 or 27 characters long (YYYY-MM-DDTHH:mm:ss.sssZ or ±YYYYYY-MM-DDTHH:mm:ss.sssZ, respectively). The timezone is always zero UTC offset, as denoted by the suffix “Z” which stands for “zero meridian”.
- The ‘console.log()’ function is used to print this ISO string to the console.
#2 – By using the toLocaleDateString() method
The toLocaleDateString() method in JavaScript is used to convert a timestamp into a more human-readable date format based on the locale of the user’s browser. To better understand this, consider the following example where we convert a Unix timestamp into a date:
let timestamp = 1609459200; // Unix timestamp in seconds
let date = new Date(timestamp * 1000); // JavaScript uses milliseconds
let formattedDate = date.toLocaleDateString();
console.log(formattedDate);
How it works
The toLocaleDateString() method works by converting a Date object into a string, using the language and formatting conventions of the user’s locale (the region and language settings of their browser).
- First, we create a variable
timestamp
and assign it a Unix timestamp value. A Unix timestamp is a way of tracking time as a running total of seconds that have elapsed since the Unix Epoch (1st January 1970 at UTC). This timestamp is considered a universal format across different systems. - Next, we create a new Date object. However, the JavaScript Date object is based on a time value that is milliseconds since the Unix Epoch, so we have to multiply the Unix timestamp by 1000.
- We then use the method
toLocaleDateString()
on the Date object. This will give us a string representation of the date, formatted according to the user’s locale. - Finally, we log the formatted date to the console to verify that the conversion has been successful.
#3 – By using Moment.js library
We’ll be using the Moment.js library to convert UNIX timestamps into human-readable date formats. To make this concept clearer, we will illustrate it with an example where a UNIX timestamp is converted into a date format like “YYYY-MM-DD HH:mm:ss”.
var moment = require('moment');
var timestamp = 1633024268; //UNIX timestamp
var convertedDate = moment.unix(timestamp).format('YYYY-MM-DD HH:mm:ss');
console.log(convertedDate);
How it works
The provided JavaScript code leverages the Moment.js library to convert a UNIX timestamp into a date format. The UNIX timestamp signifies the number of seconds that have passed since January 1, 1970, at 00:00:00 UTC (minus leap seconds). The moment.unix() function parses this timestamp, and the format() function then converts it into a readable date/time format.
- Step 1: We import the Moment.js library using the require() function.
- Step 2: We declare a variable named ‘timestamp’ and set it to a UNIX timestamp.
- Step 3: In order to convert the UNIX timestamp into a readable date format, we use the ‘moment.unix()’ function to parse the UNIX timestamp. The ‘format()’ function is then applied to present the date and time in the ‘YYYY-MM-DD HH:mm:ss’ format.
- Step 4: Finally, we use ‘console.log()’ to print the converted date in the console.
Related:
- How to Convert CSV to Array in JavaScript
- How to Check for an Empty String in JavaScript
- How to Sort Dates in JavaScript
In conclusion, converting timestamps to date format in JavaScript is a task that can be accomplished easily with the use of built-in methods like ‘new Date()’. This method simply takes the timestamp as its argument, transforming it into a human-friendly date.
By understanding and applying these simple techniques, you can manipulate timestamp data with little to no difficulty. So go ahead, start experimenting with your codes and make your JavaScript journey even more interesting.