How to Exit Functions in JavaScript
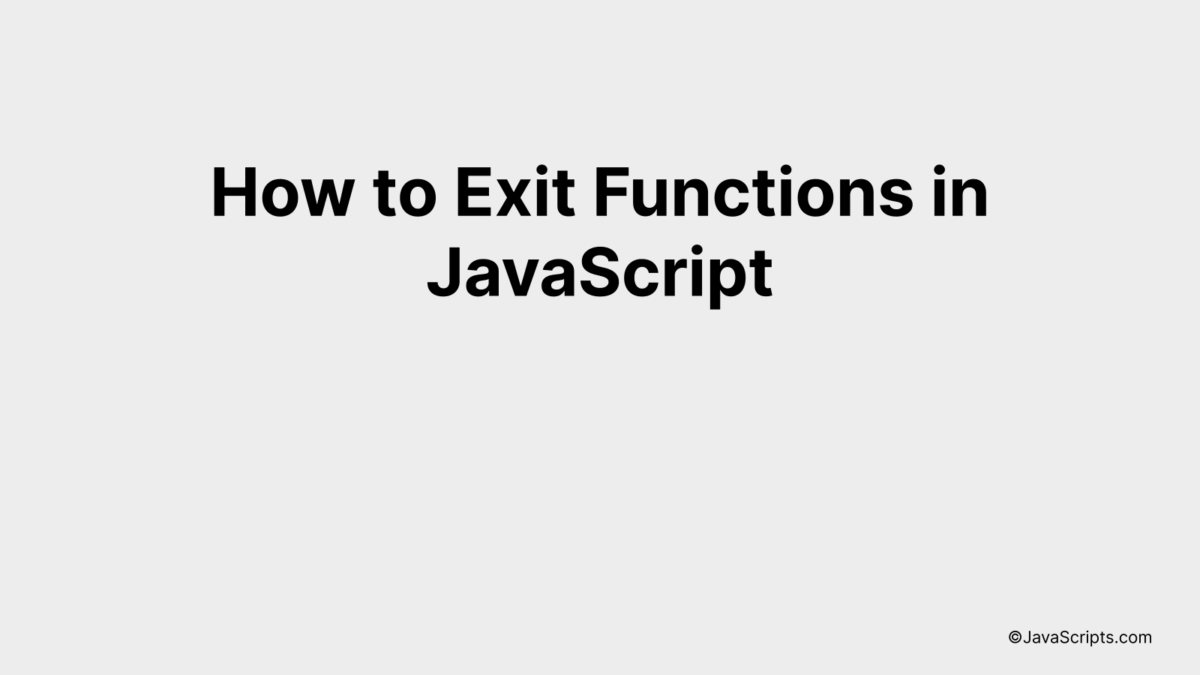
Navigating the world of JavaScript can often seem like a twisting maze with countless turns and dead ends. Don’t worry, though. You’re not alone. Many developers find themselves puzzled over one particular question: how to exit functions in JavaScript?
Imagine you’re in the middle of a script. Things seem to be going smoothly, but then you hit an obstacle. You want the function to stop, to cease its calculations and just exit. But how? That’s what we’re going to explore together.
In this post, we will be looking at the following 3 ways to exit functions in JavaScript:
- By using return statement
- By throwing an exception
- By calling process.exit() in Node.js
Let’s explore each…
#1 – By using return statement
The ‘return’ statement in JavaScript ends function execution and specifies a value to be returned to the function caller. This concept can be better understood by looking at a simple example of a function that adds two numbers.
function addTwoNumbers(num1, num2) {
return num1 + num2;
}
console.log(addTwoNumbers(5, 3)); // Outputs: 8
How it works
The ‘return’ statement used in the function ‘addTwoNumbers’ immediately stops the function and outputs the result of num1 + num2 to the location from where the function was called.
- The function ‘addTwoNumbers’ is defined with two parameters: ‘num1’ and ‘num2’.
- When the function is called, the arguments are passed in place of these parameters.
- The ‘return’ statement is encountered, which adds num1 and num2 and immediately exits the function, outputting the result to the caller of the function.
- In this case, the function is called within a ‘console.log()’ statement, so the output of the function (the sum of num1 and num2) is printed to the console.
#2 – By throwing an exception
We can halt execution of a JavaScript function by throwing an exception. This allows us to immediately exit the function when a certain condition is met. An example will help us understand this concept better.
function exitFunction() {
try {
// some code
throw new Error('Exiting function due to an error');
} catch(error) {
console.error(error.message);
}
}
How it works
The JavaScript throw statement allows us to create custom errors. When such an error is thrown, the function execution stops immediately, effectively exiting the function. The catch block then handles the exception.
- The function
exitFunction()
is declared. - Inside the function, we have a
try
block where our main code resides. If any exception is thrown within this block, it gets caught by the subsequentcatch
block. - The statement
throw new Error('Exiting function due to an error');
throws an error, stopping the execution of the function. - The function immediately exits and the control goes to the
catch
block. - The
catch
block catches the thrown error and handles it. In this case, it logs the error message to the console.
#3 – By calling process.exit() in Node.js
process.exit()
in Node.js is used to terminate a script or a function abruptly. It can be understood better with the following example where we will terminate a function based on a certain condition.
function doSomething() {
let condition = true;
if (condition) {
console.log('Condition met. Exiting...');
process.exit();
}
console.log('This will not run as the process has exited.');
}
doSomething();
How it works
When the process.exit()
function is called, it causes the node.js runtime to end the process. If this function is used within a specific function or block, it results in the termination of that block and doesn’t allow the execution of any code that follows.
- The function
doSomething
is defined first. - A variable
condition
is set totrue
. - Next, an
if
statement checks whethercondition
istrue
. - If the condition is met (which it is in this case), a message ‘Condition met. Exiting…’ is logged to the console.
- The
process.exit()
function is then called, which terminates the process. Any code following this call will not be executed. So the log message ‘This will not run as the process has exited.’ will not be printed to the console. - Finally, the function
doSomething
is invoked.
Related:
- How to Escape Quotes in JavaScript
- How to Download Files from URLs with JavaScript
- How to Check if a String Contains a Substring in JavaScript
In conclusion, exiting JavaScript functions is a central aspect of managing program flow control. By implementing return statements effectively, we can influence when and how a function ends, allowing us to create more versatile and efficient code.
Remember, using the ‘return’ keyword not only exits the function but also sends a value back to the spot where the function was called. The better you understand this concept, the more powerful and flexible your programming will be. Happy coding!