How to Find All Occurrences with JavaScript
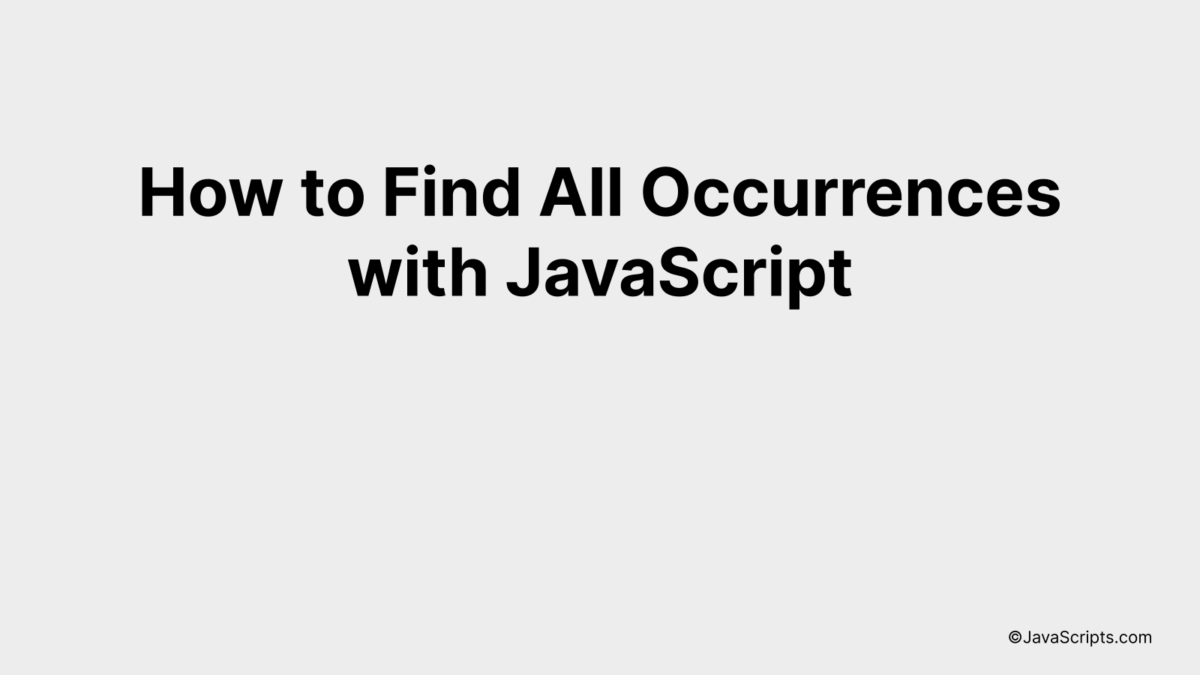
Welcome, fellow coders! Ever found yourself tangled in a complex web of code, trying to locate every instance of a specific element? You’re not alone. There’s a simple, yet powerful tool at your disposal – JavaScript.
Can JavaScript help you find all occurrences of a specific element in an array or a string? Of course, it can! Together, we’re going to unlock this mystery. Prepare to dive into the world of loops, methods and functions – all in the name of making your coding life easier.
In this post, we will be looking at the following 3 ways to find all occurrences with JavaScript:
- By using the indexOf() method in a loop
- By using the match() method with a global flag
- By using the split() method and subtracting 1 from the length
Let’s explore each…
#1 – By using the indexOf() method in a loop
The indexOf() method in JavaScript can be used inside a loop to find all occurrences of a specific string or character. We will use a simple string and a loop to demonstrate how to use indexOf() to find all occurrences.
var str = "The quick brown fox jumps over the lazy dog.";
var targetChar = "o";
var startIndex = 0;
var occurrences = [];
while (str.indexOf(targetChar, startIndex) > -1) {
var index = str.indexOf(targetChar, startIndex);
occurrences.push(index);
startIndex = index + 1;
}
console.log(occurrences); // outputs [10, 12, 26, 29, 43]
How it works
The indexOf() method is used within a while loop to continuously search for the target character in the string, starting from the last found position. Each time the target character is found, its index position is added to the occurrences array and the search continues from the next position. The loop ends when there are no more occurrences of the target character in the string.
- The variable
str
holds the string where we are searching for the target character. - The variable
targetChar
is the character we are looking for. - The variable
startIndex
is used to keep track of where we need to start looking for the next occurrence. - The variable
occurrences
is an array where we keep track of all the places where the target character is found. - The while loop continues as long as
indexOf(targetChar, start)
returns a value greater than -1, indicating that it found the target character in the string. - Inside the loop, we find the index of the target character and add it to the end of the
occurrences
array. - We then set
startIndex
to be one more than the last found index, so the next search starts from the next character in the string. - After the loop ends, we log the
occurrences
array to the console, which will contain the indexes of all occurrences of the target character.
#2 – By using the match() method with a global flag
The match() method in JavaScript is used with a global flag to find all occurrences of a specific pattern within a string. This method will return an array of all the matches found. Let’s understand this better with an example.
let string = "The cat sat on the mat.";
let pattern = /at/g;
let matches = string.match(pattern);
How it works
The match() method, when used with a global flag (‘g’), searches the entire string (‘The cat sat on the mat’) for all instances that match the specified pattern (‘at’). It returns an array of all matches found. If no matches are found, it returns null.
- The variable ‘string’ holds the string that we want to search in.
- The variable ‘pattern’ holds the regular expression pattern we’re looking for. In this case, the pattern is ‘at’. The ‘g’ following the pattern is the global flag, which tells the match() method to find all occurrences of the pattern in the string, not just the first one.
- The match() method is called on the ‘string’ with the ‘pattern’ as its parameter. The method searches ‘string’ for ‘pattern’.
- The results are stored in the ‘matches’ variable. If ‘at’ is found in ‘string’, ‘matches’ will hold an array of all occurrences. If ‘at’ is not found in ‘string’, ‘matches’ will hold the value null.
#3 – By using the split() method and subtracting 1 from the length
This method works by splitting the given string at every occurrence of the specified pattern, creating an array of substrings. The length of this array, subtracted by 1, will provide the number of occurrences of the pattern within the string. For example, if we have the string “apple, banana, apple, cherry”, and we want to find all occurrences of “apple”, this method would return 2.
let str = "apple, banana, apple, cherry";
let pattern = "apple";
let count = str.split(pattern).length - 1;
How it works
The JavaScript split() method divides a string into an array of substrings by using a specified separator string. The number of splits is determined by the number of occurrences of the separator. By subtracting 1 from the length of the array, we get the number of occurrences of the separator (in this case, our pattern) in the string.
- Step 1: A string (“str”) and a pattern (“pattern”) are defined.
- Step 2: The split() method is called on the string, with the pattern provided as the separator.
- Step 3: This method returns an array of substrings, divided at each occurrence of the pattern.
- Step 4: The length of this array is determined using the length property. This gives us the number of splits plus 1.
- Step 5: We subtract 1 from this number to get the number of occurrences of the pattern within the string.
Related:
- How to Extract Numbers from Strings in JavaScript
- How to Create Arrays with a Specific Length in JavaScript
- How to Count Elements with JavaScript
In conclusion, finding all occurrences within a string, array, or other data types is an essential skill to master in JavaScript. It’s not only useful for searching data but also for manipulating and analyzing it.
Remember, the methods we discussed like indexOf(), lastIndexOf(), match(), and filter() are your main tools for this task. With a bit of practice, you’ll be proficient in no time. Keep coding and exploring the power of JavaScript!