How to Format JavaScript Datetime
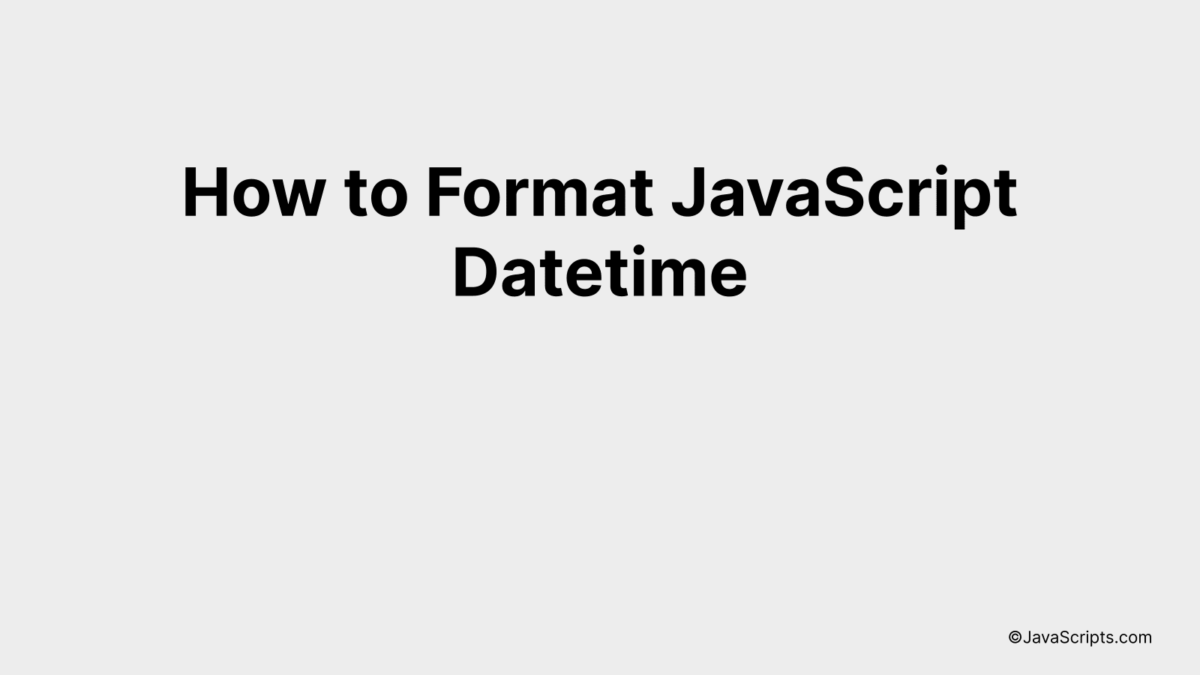
Dealing with date and time in JavaScript can sometimes feel like a tricky task, right? You may find yourself facing various challenges while formatting JavaScript datetime. But don’t worry, the solution lies within grasp.
Let’s dive into the world of JavaScript together. We’ll unravel the mysteries around datetime formatting and simplify the process. You’ll be handling date and time like a pro before you know it.
In this post, we will be looking at the following 3 ways to format JavaScript datetime:
- By using toLocaleString() method
- By using Date.prototype methods
- By using Moment.js library
Let’s explore each…
#1 – By using toLocaleString() method
The toLocaleString() method in JavaScript is used to convert a date object into a string, representing the date and time in human-readable format based on the locale settings. Let’s understand it better with an example.
let date = new Date();
let formattedDate = date.toLocaleString('en-US', { hour12: true });
How it works
The toLocaleString() method converts the date object into a readable string format based on the given locale (‘en-US’ in our case) and options (12 hour time format).
- First, we create a new date object by using the Date() constructor. This will give us the current date and time.
- Next, we use the toLocaleString() method on this date object. We pass ‘en-US’ as the locale which means we want the date and time in US English format.
- The second parameter to the toLocaleString() method is an options object. We pass ‘hour12: true’ which means we want the time in 12-hour format.
- Finally, the toLocaleString() method returns a string representing the date and time in human-readable format, which we store in the variable formattedDate.
#2 – By using Date.prototype methods
The following JavaScript code sample uses Date.prototype methods to obtain the current date and format it in the ‘YYYY-MM-DD’ format. This is demonstrated by creating a new Date object and using getFullYear, getMonth, and getDate methods to format the date accordingly.
const formatCurrentDate = () => {
let date = new Date();
let year = date.getFullYear();
let month = ("0" + (date.getMonth() + 1)).slice(-2); // getMonth() is zero-based
let day = ("0" + date.getDate()).slice(-2);
return ${year}-${month}-${day};
};
console.log(formatCurrentDate());
How it works
The JavaScript Date object represents a moment in time. By default, a new Date instance without arguments provided creates an object corresponding to the current date and time. The getFullYear, getMonth, and getDate methods are used to extract the year, month, and date from the Date object respectively, which are then formatted to ‘YYYY-MM-DD’ format.
- A new Date object is created which holds the current date and time.
- The getFullYear method is invoked on the Date object to get the current year.
- The getMonth method is invoked on the Date object, which returns the month (0-11, where 0 is January and 11 is December). Therefore, 1 is added to the result to align it with the usual human-readable format (1-12), and it’s padded with a leading zero if necessary.
- The getDate method is invoked on the Date object to get the current day of the month (1-31), and it’s padded with a leading zero if necessary.
- Finally, a string is returned in ‘YYYY-MM-DD’ format by using JavaScript’s template literals.
#3 – By using Moment.js library
With Moment.js, we will be formatting JavaScript datetime in a desired readable format. For instance, if we want to change the date format to ‘DD-MM-YYYY’, we can achieve it easily with Moment.js.
var moment = require('moment'); // require the Moment.js library
var date = new Date(); // get the current date
var formattedDate = moment(date).format('DD-MM-YYYY'); // format the date
console.log(formattedDate); // print the formatted date
How it works
The above code uses Moment.js to format a JavaScript datetime object into a more human-readable form. It first requires the Moment.js library, fetches the current date, formats it, and then logs the formatted date to the console.
- First, the Moment.js library is imported using ‘require’ function in Node.js.
- Then, the current date and time is retrieved using JavaScript’s ‘new Date()’ function, which is stored in the ‘date’ variable.
- The ‘date’ variable is then passed to the ‘moment()’ function of Moment.js. Following this, the ‘format()’ function is used to format the date in ‘DD-MM-YYYY’ format.
- Finally, the formatted date is printed to the console using ‘console.log()’ function.
Please replace require(‘moment’) with the appropriate import statement if you are using ES6 syntax or running this in a browser environment.
Related:
- How to Access JavaScript Text Content
- How to Check if an Array is Empty in JavaScript
- How to Break from a JavaScript ForEach Loop
In conclusion, properly formatting JavaScript Datetime is an essential task for developers, ensuring their applications display the right time-based data to users. Utilizing JavaScript’s built-in Date object, along with methods like getHours, getMinutes and getSeconds, can help you manage this effectively.
Remember, for complex formatting needs, libraries like Moment.js or Day.js offer valuable resources. With these techniques and tools, you can effectively convert, manipulate, and display dates and times in JavaScript to enhance your applications’ usability.