How to Format Numbers with Commas in JavaScript
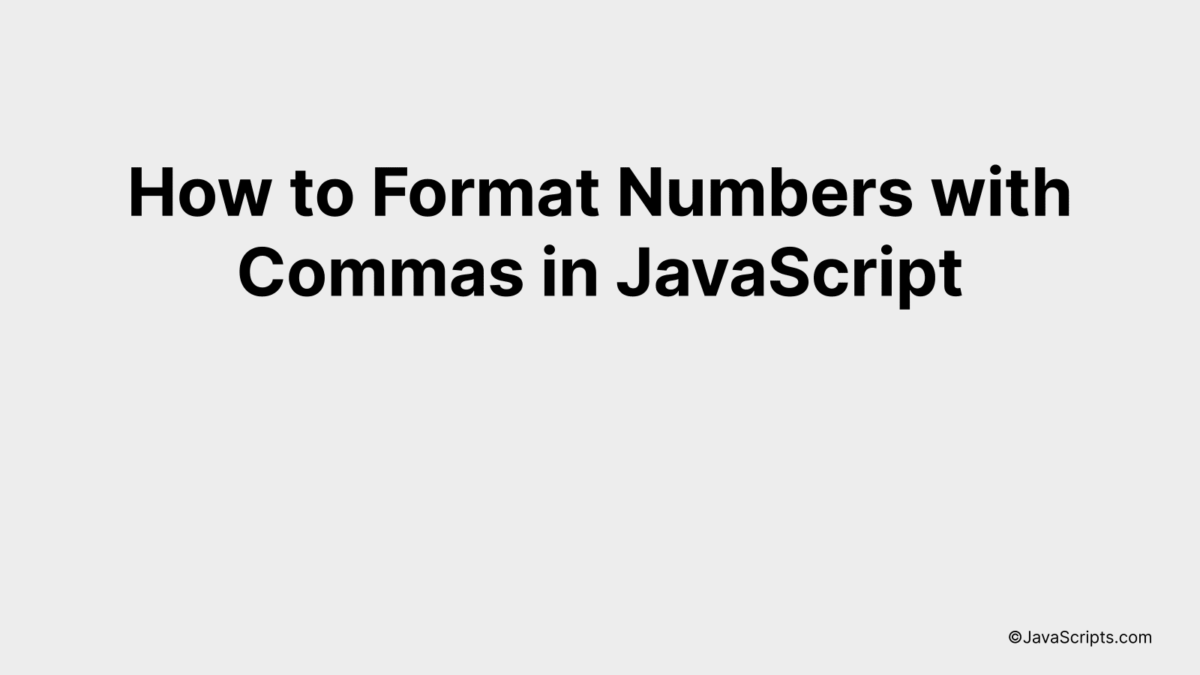
Ever wondered how to make numbers more readable in JavaScript? When dealing with large numbers, it can become hard to quickly understand their magnitude. A solution? Formatting numbers with commas!
You and I both know that JavaScript is a powerful language with vast capabilities. However, it might not be immediately obvious how to format numbers with commas. No worries, though! We’ll delve into an easy-to-understand method to accomplish this.
In this post, we will be looking at the following 3 ways to format numbers with commas in JavaScript:
- By toLocaleString() method
- By using regular expressions
- By using custom helper function
Let’s explore each…
#1 – By toLocaleString() method
The toLocaleString() method in JavaScript allows you to format numbers with commas as separators which helps in improving readability. For example, the number “1000000” would be transformed into “1,000,000”.
let num = 1000000;
let formattedNum = num.toLocaleString();
console.log(formattedNum); // Prints: 1,000,000
How it works
The toLocaleString() method in JavaScript converts a number into a string using the current or specified locale’s conventions.
- The variable
num
is declared and assigned the number “1000000”. - The
toLocaleString()
method is called on thenum
variable. This method converts the number to a string, and formats it according to the locale’s conventions. By default, it uses the current locale, and for most English-speaking locales, this means adding comma as the thousand separator. - The formatted string is assigned to the variable
formattedNum
. - The
console.log()
method is used to print the value offormattedNum
to the console. As you can see, the number is now formatted with commas as separators.
#2 – By using regular expressions
We will be using a regular expression with JavaScript’s replace function to format a number with commas as thousand separators. For example, the number 1234567 will be formatted as 1,234,567.
let num = 1234567;
let formattedNum = num.toString().replace(/B(?=(d{3})+(?!d))/g, ",");
How it works
The regular expression used in the replace function will match every position in the string (which is a number) that has a multiple of three digits to its right. Then, it replaces that matched position with a comma, thereby adding commas as thousand separators to the number.
- Step 1: Convert the number to a string using toString() method.
- Step 2: Apply the replace() method on the string. This method takes two parameters: the substrings to be replaced (which can be a regular expression) and the new substring.
- Step 3: In the regular expression, B is a word boundary that ensures that the match must not start at the beginning of the word.
- Step 4: (?=(d{3})+(?!d)) is a positive lookahead that matches every position in the string that has a multiple of three digits to its right and no digit at the rightmost of these three.
- Step 5: The g flag in the regular expression enables global search which means it will find all matches rather than stopping after the first match.
#3 – By using custom helper function
This function will convert a number into a string format that adds commas as thousands separators. For example, the number 1000000 will be formatted as ‘1,000,000’.
function formatNumberWithCommas(number) {
return number.toString().replace(/B(?=(d{3})+(?!d))/g, ",");
}
How it works
This function works by converting the number into a string and then using a regular expression to add commas at every thousandth place.
- The number is first converted to a string using the
toString()
method. - We then use the
replace()
method, which replaces parts of a string with another string based on a pattern. - The pattern used here is a regular expression
/B(?=(d{3})+(?!d))/g
, which targets every position in the string that has a multiple of three digits to its right and not followed by another digit. - Every matched position as per the pattern is then replaced by a comma, resulting in the insertion of commas at every thousandth place from the right.
Related:
- How to Format Dates as yyyy-mm-dd in JavaScript
- How to Check for Empty Strings in JavaScript
- How to Convert Timestamps to Dates in JavaScript
In conclusion, understanding how to format numbers with commas in JavaScript is quite simple yet crucial. It’s an essential skill in coding that enables you to present data more clearly to users.
This blog post has hopefully provided an easy-to-understand guide for that. With practice, you’ll soon master the art of manipulating numbers to improve user experience.