How to Get the Last Character of a String in JavaScript
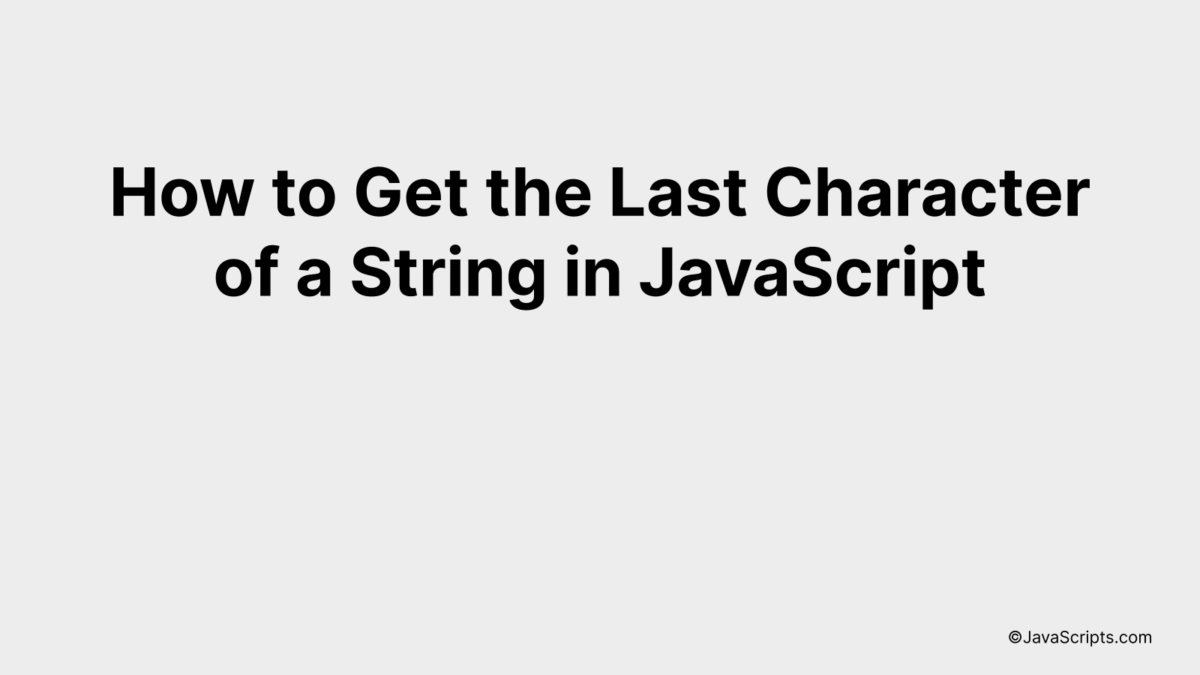
Engaging with JavaScript can sometimes feel like unraveling a ball of yarn. You tug a little, and suddenly you’re handling a plethora of functions and tools. One such useful trick involves extracting the last character from a string. It’s simpler than you might think!
You and I will walk through this fascinating journey together. Let’s dive into the world of JavaScript and unveil the mystery of extracting the last character from any given string. This knowledge will surely boost your problem-solving skills!
In this post, we will be looking at the following 3 ways to get the last character of a string in JavaScript:
- By using charAt() method
- By using slice() method
- By using bracket notation with length property
Let’s explore each…
#1 – By using charAt() method
The charAt() method in JavaScript returns the character at a specified index in a string. In this case, we will use it to retrieve the last character of a string, by passing the index of the last character (string’s length minus 1) to the charAt() method.
For example, if we have a string “Hello”, the last character is “o”. By calling “Hello”.charAt(“Hello”.length – 1), we can get the last character “o”.
let str = "Hello";
let lastChar = str.charAt(str.length - 1);
How it works
The charAt() method accesses a character from a string based on the index we provide. Here, we are retrieving the last character of a string by passing the index as string’s length minus 1, because string’s length is always 1 more than the index of the last character (as index starts from 0).
- First, we declare a variable ‘str’ and assign a string value “Hello” to it.
- Then, we declare another variable ‘lastChar’.
- To get the last character of the string, we call the charAt() method on the string ‘str’ and pass ‘str.length – 1’ as the argument. ‘str.length’ gives us the total number of characters in the string. As the index starts from 0, the index of the last character is ‘str.length – 1’.
- The charAt() method returns the character at the given index and assigns it to the ‘lastChar’ variable.
- So ‘lastChar’ now holds the last character of the string ‘str’.
#2 – By using slice() method
In JavaScript, you can obtain the last character of a string using the slice() method, which extracts a section of a string and returns it as a new string. In this case, we’ll be extracting the last character by passing -1 as the argument to the slice method, as it represents the index from the end of the string. For instance, if we have a string “Hello”, the last character “o” can be obtained by using slice(-1).
let str = "Hello";
let lastCharacter = str.slice(-1);
console.log(lastCharacter); // Outputs: "o"
How it works
The slice() method extracts a section of a string based on the provided start and end index values and then returns this portion as a new string. It doesn’t modify the original string. In our example, we are passing -1 as the argument to slice(), which means that we start slicing the string from the end (the last character).
- We declare a string “Hello” and assign it to the variable ‘str’.
- We call the slice() method on ‘str’ with an argument of -1. Since negative indexes count from the end of the string, -1 refers to the last character of ‘str’.
- The slice() method extracts this last character and returns it as a new string, which we assign to the variable ‘lastCharacter’.
- Finally, we log ‘lastCharacter’ to the console, which outputs “o”, the last character of the starting string “Hello”.
#3 – By using bracket notation with length property
In JavaScript, you can get the last character of a string by using bracket notation with the string’s length property. This approach works by specifying the position of the character you want, which in this case is the string’s length minus 1, due to zero-based indexing.
Here is a code snippet that demonstrates how to get the last character of a string:
let str = "Hello, World!";
let lastChar = str[str.length - 1];
How it works
In JavaScript, strings are treated as an array of characters, so you can access individual characters using bracket notation. The position of characters in a string (or any array) is zero-based. This means that the first character is at position 0, the second character at position 1, and so on. Therefore, to get the last character of a string, you need to access the character at position str.length – 1.
Here’s a step-by-step explanation of how the code works:
- First, a string is created and assigned to the variable str.
- Then, we’re using bracket notation to access an individual character of the string. Inside the brackets, we’re writing str.length – 1.
- str.length gives us the length of the string, which in this case is 13 (for “Hello, World!”).
- Since the indexing of characters starts from 0, the index of the last character is str.length – 1, which in this case is 12.
- So str[str.length – 1] gives us the last character of the string, which is “!” in this case.
- This value is then stored in the variable lastChar.
Related:
- How to Flatten Objects in JavaScript
- How to Check if a Variable is Defined in JavaScript
- How to Check if a Function Exists in JavaScript
In conclusion, getting the last character of a string in JavaScript is a simple task. The methods we’ve discussed, like using charAt and slice, make this easy even for beginners.
Mastering such basics will boost your overall programming efficiency. So keep practicing, ensure to test your codes, and you will get better day by day.