How to Use One-Line If Statements in JavaScript
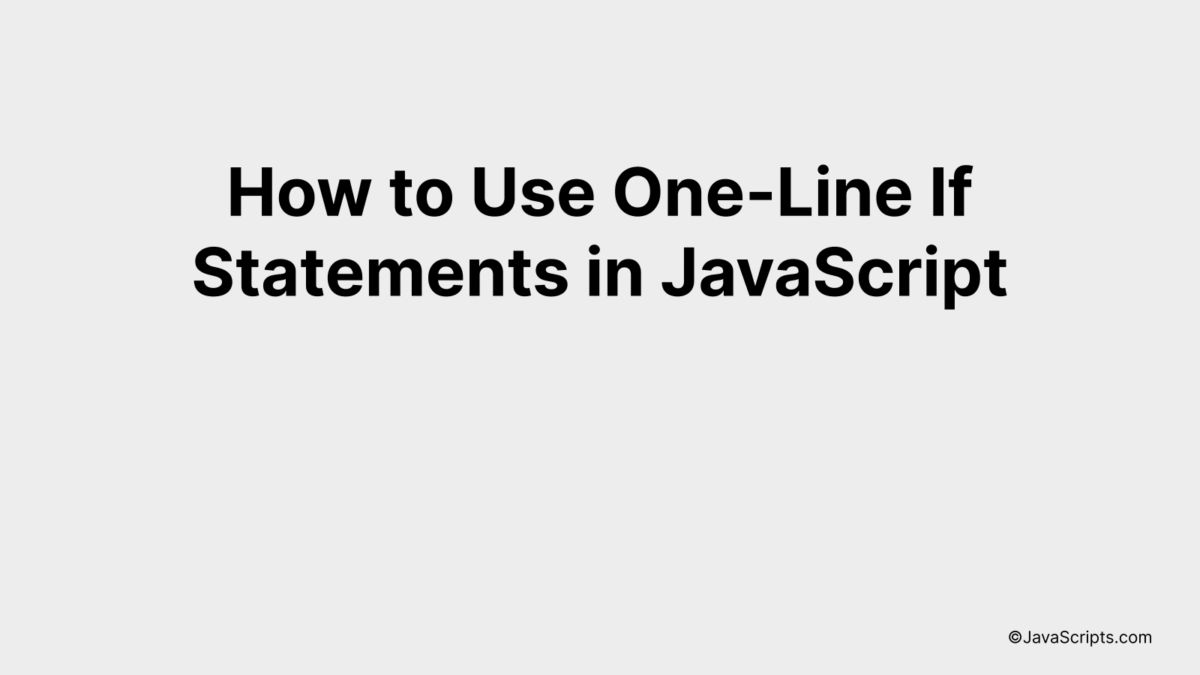
When we’re knee-deep in code, every keystroke matters. As we strive for efficiency and readability, JavaScript’s one-line if statements rise as unsung heroes. They’re concise, potent, and enhance the elegance of our code.
Let’s imagine you’re navigating the labyrinth of JavaScript for the first time or you’re already a seasoned coder. Either way, you’ll find one-line if statements to be beneficial. They save precious time, reduce your lines of code, and make your scripts easier to understand.
In this post, we will be looking at the following 3 ways to use one-line if statements in JavaScript:
- By using the Ternary Operator
- By using the short-circuit evaluation with Logical AND Operator
- By using the short-circuit evaluation with Logical OR Operator
Let’s explore each…
#1 – By using the Ternary Operator
The ternary operator in JavaScript allows us to write compact, one-liner conditional statements. It checks a condition and returns one value if the condition is true, and another value if it is false. For example, a ternary operator can be used to decide which text to show based on a boolean value.
Here’s an example:
let isDay = true;
let timeMessage = isDay ? "Good Morning!" : "Good Night!";
How it works
This one-line statement first checks if the isDay variable is true or false. If it’s true, it assigns the string “Good Morning!” to timeMessage. If it’s false, it assigns “Good Night!” to timeMessage.
Here’s the step-by-step explanation:
- The variable isDay is set to true.
- The ternary operator ? checks whether isDay is true or false.
- Since isDay is true, the expression before the colon (:) is returned, so “Good Morning!” is assigned to timeMessage.
- If isDay were false, the expression after the colon would be returned, so “Good Night!” would be assigned to timeMessage.
#2 – By using the short-circuit evaluation with Logical AND Operator
Short-circuit evaluation using the Logical AND Operator (&&) in JavaScript allows for executing a statement only if a certain condition is true. This works by leveraging how JavaScript evaluates the second operand in an AND operation only if the first operand is truthy.
let isAdult = true;
isAdult && console.log("You are allowed to enter!");
How it works
In JavaScript, the logical AND (&&) operator short-circuits. This means that it stops evaluating as soon as it encounters a false or falsy value. If the first operand is truthy, only then the second operand is evaluated and executed. In the example above, the console.log statement is only executed if isAdult is true.
Here is a step-by-step explanation:
- Let’s assume isAdult is a variable representing whether a person is an adult or not.
- When the && operator is encountered, it first evaluates the expression on its left, i.e., isAdult.
- If isAdult is true (a truthy value), it then evaluates and executes the statement on the right. In this case, it will execute console.log(“You are allowed to enter!”).
- If isAdult is false (a falsy value), it will not evaluate and execute the statement on the right. Hence, nothing will be logged to the console.
#3 – By using the short-circuit evaluation with Logical OR Operator
In JavaScript, we can use logical OR (||) operator in a one-line if statement, where the first operand is a condition check and the second operand is an action that occurs if the condition is false. This technique is known as short-circuit evaluation and is handy for preventing errors when trying to access properties on undefined or null references.
Here is an example:
let x;
let y = x || 'Default value';
How it works
In this example, if x is undefined, null, 0, NaN, false, or an empty string, y will be assigned the string ‘Default value’ due to the short-circuit behavior of the logical OR operator.
- First, the JavaScript engine evaluates the expression from left to right.
- If the first operand (x in our case) can be converted to true, it short-circuits and returns the value of x.
- If x is falsy (i.e., it’s undefined, null, 0, NaN, false, or an empty string), it proceeds to the second operand.
- Since x is undefined in this code sample, the OR operator moves to the next operand, ‘Default value’, and assigns this value to y.
Related:
- How to Uncheck Checkboxes with JavaScript
- How to Split an Array into Chunks in JavaScript
- How to Set Background Color with JavaScript
In conclusion, one-line if statements in JavaScript, also known as ternary operators, make your code more compact and neat. It’s an efficient way to assign values based on conditionals, with less syntax and greater readability.
However, while they offer brevity, remember to use them judiciously to keep the code understandable and maintainable. Like any tool, their real power lies in knowing when and how to use them effectively, keeping the balance between simplicity and clarity.