How to Wait for Elements to Exist in JavaScript
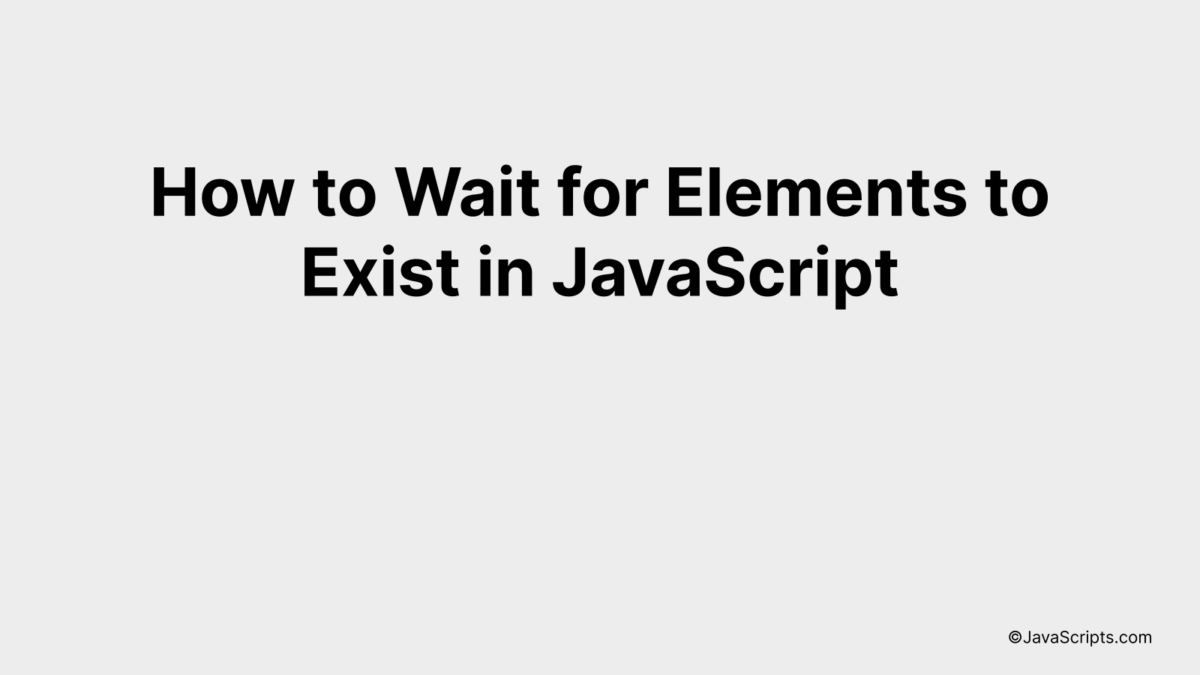
Let’s face it, web development can sometimes feel like a game of patience, especially when you’re waiting for elements to exist in JavaScript. It might feel like you’re a fisherman, casting your line out and waiting for the big catch.
Don’t worry, we’re in the same boat. Together, we’ll unravel the mystery of how to effectively wait for elements to exist in JavaScript. It’s simpler than you might think, and by the end, you’ll be navigating these waters with ease.
In this post, we will be looking at the following 3 ways to wait for elements to exist in JavaScript:
- By using the ‘setTimeout’ function
- By using the ‘setInterval’ function
- By using the ‘MutationObserver’ API
Let’s explore each…
#1 – By using the ‘setTimeout’ function
This method involves using JavaScript’s ‘setTimeout’ function to periodically check if a particular element exists in the DOM. If it does, we proceed with our operation; if not, we wait and check again after a specified time, thus creating a kind of polling mechanism. An example will make this clearer.
function waitForElementToDisplay(selector, time) {
if(document.querySelector(selector)!=null) {
console.log("The element is displayed, you can go on now.");
return;
}
else {
setTimeout(function() {
waitForElementToDisplay(selector, time);
}, time);
}
}
How it works
The function waitForElementToDisplay uses a selector to search for an element in the DOM. If the element is found, it logs a message to the console. If the element is not found, it waits for a time period before trying to find the element again. This creates a loop that continues until the element is found.
- The function waitForElementToDisplay accepts two arguments: a selector (to identify the desired element on the web page) and a time period in milliseconds.
- Inside the function, we use document.querySelector(selector) to search for the element in the DOM.
- If the element is found (document.querySelector(selector)!=null), we log a message to the console and return from the function.
- If the element is not found, we set a timeout (setTimeout) to call waitForElementToDisplay again after the specified time period. This essentially means “wait for this amount of time, then try to find the element again”.
- This will continue in a loop until the element is found, at which point the function returns and the looping stops.
#2 – By using the ‘setInterval’ function
We are going to periodically check the existence of an element on a webpage using JavaScript’s ‘setInterval’ function, which continually calls a function at set time intervals until it is stopped. This method is particularly useful when you want your script to wait for an element to load or appear on the page before performing an action. Let’s understand this with an example.
var checkExist = setInterval(function() {
if (document.querySelector('#myElement')) {
console.log("#myElement exists!");
clearInterval(checkExist);
}
}, 1000); // check every 1000ms
How it works
The above JavaScript code uses the ‘setInterval’ function to periodically check if a specific HTML element (in this case, an element with the id of “myElement”) exists on the webpage. If the element exists, it prints a message to the console and stops checking. If not, it continues checking every second (1000 milliseconds).
Here is the step-by-step explanation:
- The ‘setInterval’ function is called with two parameters: a function to execute and the time interval between executions (in milliseconds).
- Inside the function, the ‘document.querySelector’ method is used to select the first element with the id of “myElement”.
- If ‘querySelector’ finds the element, it returns the element, and this value will be truthy (i.e., it will evaluate to ‘true’ in a boolean context).
- If the element exists, a message is printed to the console using ‘console.log’.
- Once the element is found, ‘clearInterval’ is used to stop the ‘setInterval’ function from running further.
- If the element does not exist, ‘querySelector’ returns ‘null’, which is a falsy value (it evaluates to ‘false’ in a boolean context), so the ‘console.log’ statement is not executed, and the ‘setInterval’ function continues checking every second.
#3 – By using the ‘MutationObserver’ API
The ‘MutationObserver’ API in JavaScript is used to watch for changes in the DOM of a webpage, such as when elements are added, modified, or removed. A practical example can help illustrate its usage and mechanics.
const observer = new MutationObserver((mutationsList, observer) => {
for(let mutation of mutationsList) {
if (mutation.type === 'childList') {
console.log('A child node has been added or removed.');
}
else if (mutation.type === 'attributes') {
console.log('The ' + mutation.attributeName + ' attribute was modified.');
}}});
// Start observing the target node for configured mutations
const targetNode = document.getElementById('some-id');
observer.observe(targetNode, { attributes: true, childList: true, subtree: true });
// Later, you can stop observing
// observer.disconnect();
How it Works
The MutationObserver API allows you to react to changes in the DOM in real-time by observing specific elements and triggering a callback function whenever they are mutated.
- The MutationObserver is instantiated by passing a callback function which will be called whenever a mutation occurs. The callback receives an array of MutationRecords and the observer instance itself.
- The ‘observe’ method is then used on the observer instance, specifying the targetNode to be observed and the type of mutations to observe for (in this case, changes to attributes, childList, and subtree).
- Within the callback function, we loop through each mutation in the mutationsList and check the ‘type’ property to determine what kind of mutation occurred. Depending on the type, an appropriate action is taken.
- If required, the observation can be stopped at any moment by using the ‘disconnect’ method on the observer instance.
Related:
- How to Use a Variable as a Key in JavaScript
- How to Update Objects in Arrays with JavaScript
- How to Simulate Keypress Events in JavaScript
In conclusion, waiting for elements to exist in JavaScript is an essential aspect of web development. Proper handling of these elements keeps your website responsive and efficient, enhancing user experience significantly. JavaScript provides multiple methods like ‘setInterval’, ‘MutationObserver’, and ‘setTimeout’ to deal with this aspect.
Understanding and implementing these methods is not complicated. All you need is to identify which method best suits your requirements. So, keep experimenting, keep learning, and make your web pages more dynamic and interactive.