How to Use a Variable as a Key in JavaScript
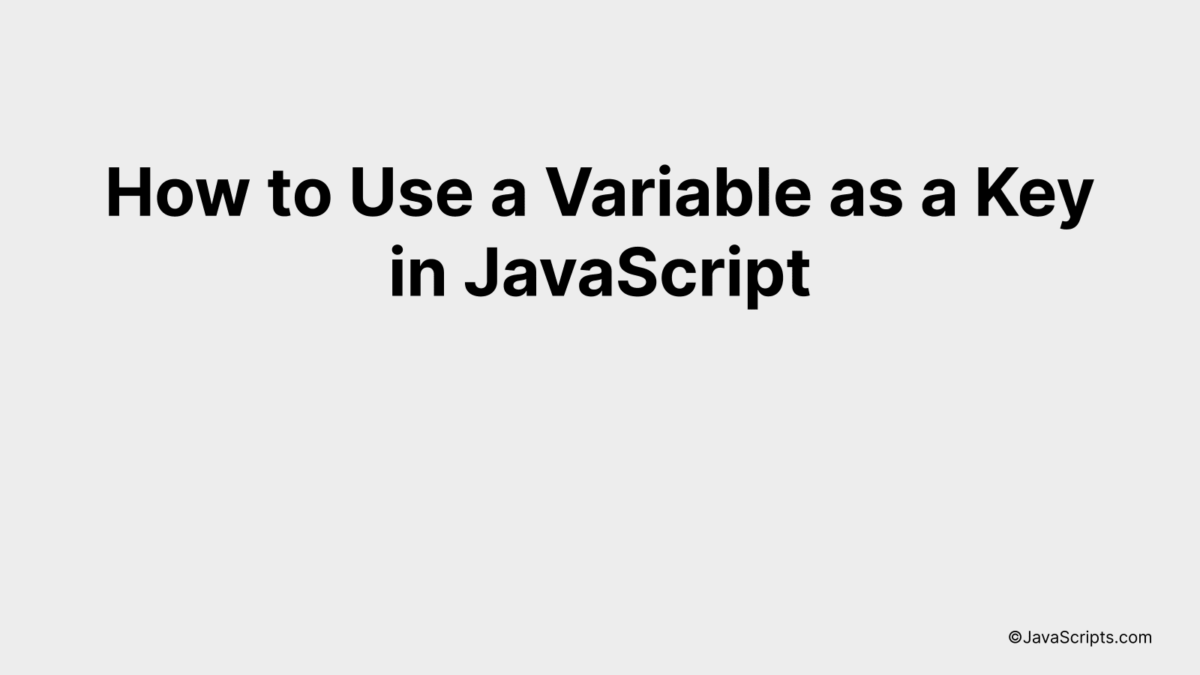
Navigating JavaScript can often feel like traversing an intricate labyrinth, especially when it comes to handling key-value pairs within objects. What if you want to use a variable as a key? This might seem challenging if you are new to programming, or if you have just started your JavaScript journey.
Let’s demystify this concept together. You and I will delve into a simple, yet powerful approach to using variables as keys in JavaScript objects. By the end of our exploration, the seemingly complex idea will become as clear as day. It’s time to turn your confusion into confidence!
In this post, we will be looking at the following 3 ways to use a variable as a key in JavaScript:
- By Dot Notation Method
- By Bracket Notation Method
- By using ES6 Computed Property Names
Let’s explore each…
#1 – By Dot Notation Method
In JavaScript, you can use a variable as a key by using the bracket notation where the variable name is enclosed within square brackets. This method allows dynamic property access on an object.
var key = 'username';
var obj = {};
obj[key] = 'JohnDoe';
How it works
Bracket notation in JavaScript can take in a variable that resolves to a string, to dynamically assign and access the properties of an object.
- The
key
variable is assigned the string value ‘username’. - An empty object
obj
is created. - The property
username
is added to the objectobj
by referencing the variablekey
inside the brackets. This is written asobj[key]
. Since thekey
variable holds the string ‘username’, it’s equivalent to writingobj['username']
. - The property
username
is then assigned the value ‘JohnDoe’. So, the objectobj
now has one property:username
with its value being ‘JohnDoe’.
#2 – By Bracket Notation Method
Bracket notation in JavaScript allows you to use a variable as a key in an object. The variable, when used in bracket notation, is evaluated to its associated value, which then becomes the key used to access the value in the object.
var customer = {};
var key = "firstName";
customer[key] = "John";
console.log(customer.firstName); // Outputs: John
How it works
In the provided example, a variable is used as an object’s key to assign and retrieve a value. The JavaScript engine evaluates the variable to its actual value, using this as the key in the object.
- We first declare a variable
customer
and initialize it as an empty object. - Next, we declare another variable
key
and assign the string"firstName"
to it. - Using the bracket notation, we assign the value
"John"
to the property incustomer
object. Here, the variablekey
is evaluated to its value"firstName"
which becomes the key in thecustomer
object. - Finally, we log the value of the
firstName
property of thecustomer
object to the console. Since we have assigned the value"John"
to this property, it logs"John"
.
#3 – By using ES6 Computed Property Names
In ES6, JavaScript introduced computed property names that allow you to dynamically create object keys using variables. This lets you create more dynamic and flexible data structures. Here’s an example:
let variable = "key";
let obj = { [variable]: "value" };
How it works
When creating an object in JavaScript, you typically define a set of key-value pairs. However, with ES6 computed property names, you can use a variable wrapped in square brackets ([variable]) instead of a static text key. The JavaScript engine will evaluate the variable, convert it to a string (if it’s not already one), and use that as the key in the object.
Let’s break it down:
- First, we define a variable with the string “key”.
- Next, we create an object where instead of using a hard-coded string as the key, we put the variable inside square brackets. This is the syntax for computed property names.
- When the object is created, JavaScript evaluates the expression inside the square brackets (in this case, it’s just our variable). It converts the result of that expression to a string and uses it as the key in our object.
- Finally, we have an object with one property, “key”, which was dynamically set using our variable. The value of this property is “value”.
Related:
- How to Update Objects in Arrays with JavaScript
- How to Simulate Keypress Events in JavaScript
- How to Set Sizes with JavaScript
In conclusion, using a variable as a key in JavaScript can be achieved straightforwardly. With ES6’s dynamic property names, we can assign and access variable keys in an object effortlessly.
This feature opens up newer, more flexible ways for developers to code. Remember, practice makes perfect so keep coding and experimenting with these concepts to gain a solid understanding.