How to Add a Property to an Object in JavaScript
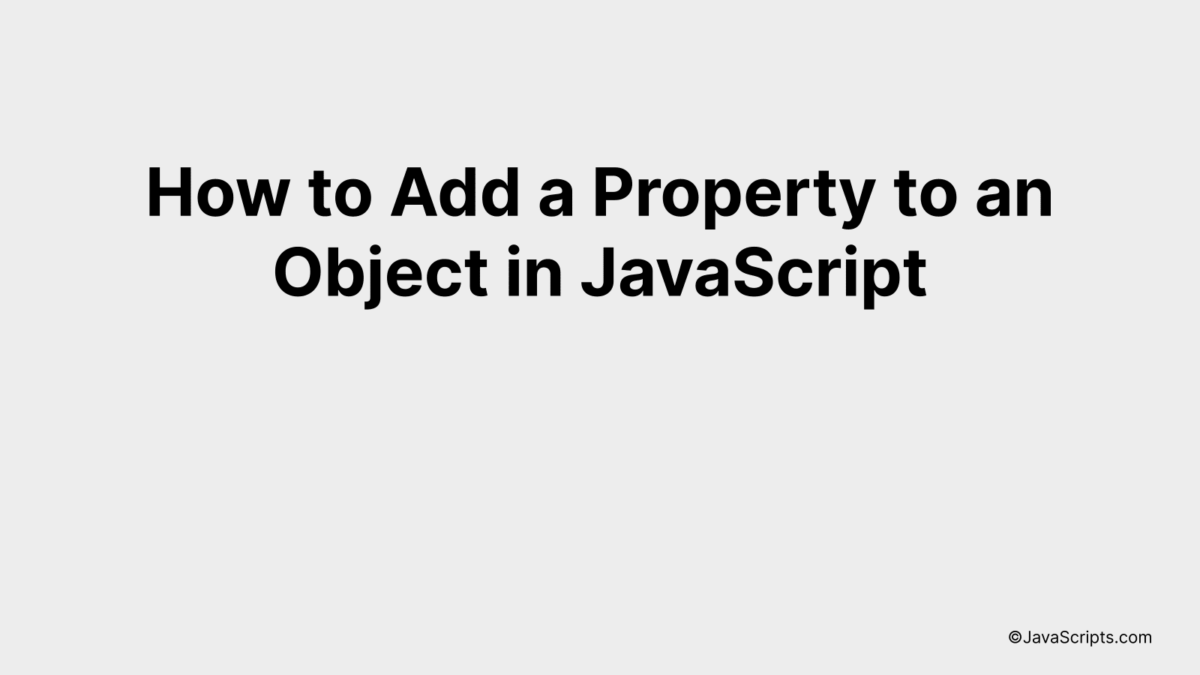
Navigating the world of JavaScript, you may have discovered the key role objects play in the language. Objects, like real-world containers, hold labeled data which can be manipulated, analyzed, and put to work.
But how do you add new properties to these objects? Perhaps you’re working on a project and need to incorporate an extra piece of information to an existing object. Whatever the case, we’ll delve into the process together, ensuring you have a solid grasp on adding properties to JavaScript objects.
In this post, we will be looking at the following 3 ways to add a property to an object in JavaScript:
- By dot notation
- By bracket notation
- By Object.defineProperty() method
Let’s explore each…
#1 – By dot notation
In JavaScript, adding a property to an object can be achieved using dot notation. It is a direct and straightforward way to add properties, where you simply follow the object’s name with a dot, then the new property’s name, and assign a value to it.
var obj = {};
obj.newProperty = 'New Value';
How it works
In the given script, we have created an object and added a new property to it using the dot notation. This method attaches a property directly to an object, which can then be accessed or modified.
- Step 1: An empty object named ‘obj’ is created using the literal notation
var obj = {};
. - Step 2: A new property ‘newProperty’ is added to the ‘obj’ object using the dot notation:
obj.newProperty
. Here, ‘newProperty’ is the name of the property, and the assignment operator is used to assign a value to it. In our case, the value assigned is the string ‘New Value’. - Step 3: The property can now be accessed from the object using the dot notation, like so:
console.log(obj.newProperty)
, and it will output ‘New Value’.
#2 – By bracket notation
In JavaScript, you can add a property to an object using bracket notation by defining the object name, followed by the new property name enclosed in square brackets and an equals sign to set its value. This method is particularly useful when the property name is dynamically determined (like in a loop), or when it cannot be a valid identifier. Let’s consider a simple example:
var obj = {};
obj['property1'] = 'Value of property1';
How it works
JavaScript objects are dynamic data structures, which means you can add properties to them post-creation. In this case, we used bracket notation to add a new property to the object.
- First, we create an empty JavaScript object using curly braces {} and assign it to the variable
obj
. - Next, we add a new property to the object using bracket notation. Inside the brackets, we provide the name of the new property as a string. In this case, it’s ‘property1’.
- Finally, we assign a value to the new property using an equals sign. The value can be any valid JavaScript value. In this example, we’re assigning the string ‘Value of property1’ to the
property1
. - Now,
obj
has one property –property1
– which holds the value ‘Value of property1’.
Make sure to replace the and at the start and end of the block respectively with < and > to render it correctly in HTML. The and are used here only to display the HTML content without rendering it.
#3 – By Object.defineProperty() method
The Object.defineProperty() method in JavaScript allows you to directly define or modify a property on an object, and control its configuration. By using an example we can understand it better.
var obj = {};
Object.defineProperty(obj, 'property1', {
value: 42,
writable: true,
enumerable: true,
configurable: true
});
How it works
The Object.defineProperty() method takes three arguments: the object on which the property is defined, the name of the property, and a descriptor object that defines the properties’ configuration. In the example above, we are defining a property ‘property1’ with a value of 42 on object ‘obj’.
- Step 1: An empty object ‘obj’ is created.
- Step 2: The Object.defineProperty() method is called. The first argument is the object on which the property will be defined, which is ‘obj’ in our case.
- Step 3: The second argument is the name of the property to be defined, ‘property1’ here.
- Step 4: The third argument is the descriptor object that defines the configuration of the property. This includes:
- value: This defines the value of the property. In our case, the value is 42.
- writable: This defines whether the property can be changed or not. In our case, it is set to true, which means the value of ‘property1’ can be changed.
- enumerable: This defines whether the property will be returned in a loop over the object properties. In our case, it is set to true, which means ‘property1’ will be returned in a loop over the properties of ‘obj’.
- configurable: This defines whether the property can be deleted or changed. In our case, it is set to true, which means ‘property1’ can be deleted or changed.
Related:
- How to Get Current Year in JavaScript
- How to Format JavaScript Datetime
- How to Access JavaScript Text Content
In conclusion, JavaScript gives us flexible and straightforward ways to add properties to objects. The dot and bracket notations are the most commonly used methods, and they help in dynamic and efficient data management.
Remember, practice is the key to mastering these techniques. Keep coding, and soon adding properties to JavaScript objects will become second nature to you.