How to Check if an Object Has a Property in JavaScript
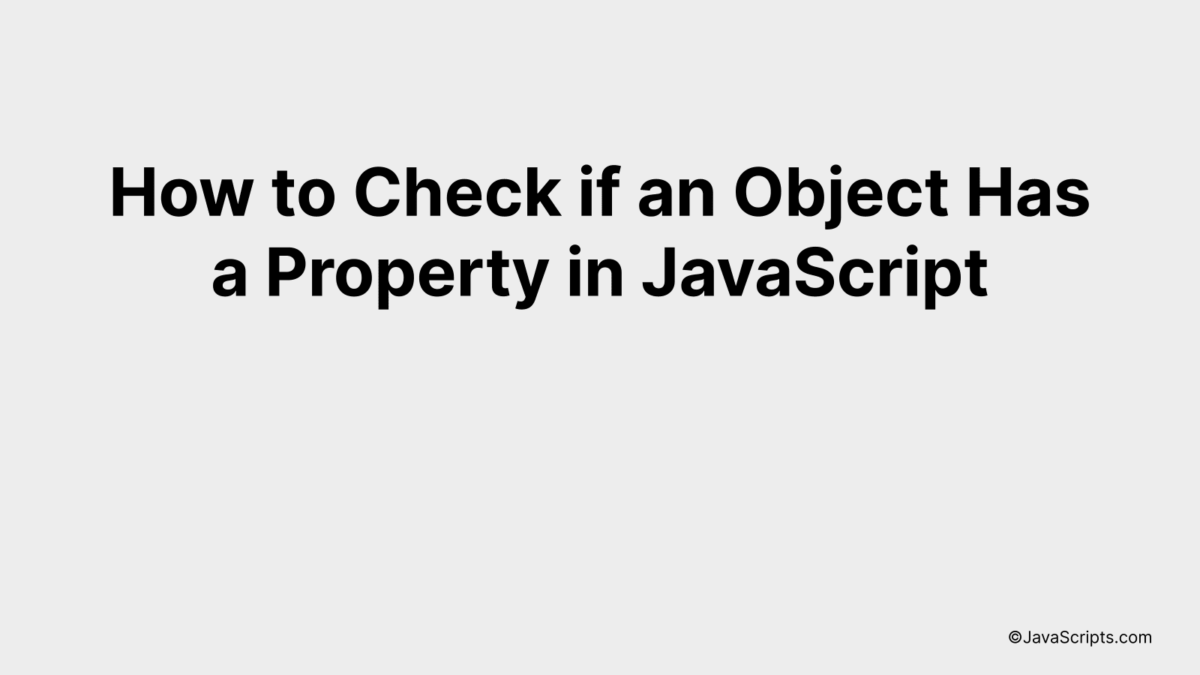
Understanding the properties of an object is a vital skill in JavaScript. Often, we find ourselves needing to check if an object possesses certain properties before performing operations. It’s like verifying if we have the right tools before starting a task.
As a JavaScript developer, you might have encountered such situations. Or perhaps you’re a beginner, still navigating your way. Regardless, this short guide will help you identify whether an object has a specific property. Simple yet powerful ways to check object properties are right on your fingertips!
In this post, we will be looking at the following 3 ways to check if an object has a property in JavaScript:
- By using the ‘in’ operator
- By using the ‘hasOwnProperty()’ method
- By using the ‘propertyIsEnumerable()’ method
Let’s explore each…
#1 – By using the ‘in’ operator
The ‘in’ operator in JavaScript is used to check if an object has a specific property. It returns ‘true’ if the property is in the object, and ‘false’ if it is not. The usage is straightforward as shown in the example below.
var myObject = {
property1: "Value1",
property2: "Value2"
};
console.log("property1" in myObject); // Output: true
console.log("property3" in myObject); // Output: false
How it works
The ‘in’ operator checks the existence of properties in an object in JavaScript. It goes through the entire prototype chain of the object, meaning it will return ‘true’ for properties not just directly on the object, but also on any of its ancestors in the prototype chain.
- Step 1: Initialize an object with properties. Here, myObject is initialized with property1 and property2.
- Step 2: Use the ‘in’ operator to check the existence of a property. In the code, we are checking for the existence of property1 and property3 in myObject.
- Step 3: The ‘in’ operator returns ‘true’ if the property exists in the object or in its prototype chain; otherwise, it returns ‘false’. Here, ‘true’ is returned for property1 as it exists in myObject, and ‘false’ for property3 as it is not present.
#2 – By using the ‘hasOwnProperty()’ method
The ‘hasOwnProperty()’ function in JavaScript is used to check if an object has a specific property. It returns a boolean value: ‘true’ if the property exists, and ‘false’ if it doesn’t. Let’s understand this with an example.
var myObject = {
name: "Alice",
age: 25
};
console.log(myObject.hasOwnProperty('name')); // true
console.log(myObject.hasOwnProperty('address')); // false
How it works:
The ‘hasOwnProperty()’ method in JavaScript is used to determine whether an object has a property with the specified name. It returns a boolean indicating whether the object has the specified property as its own property (as opposed to inheriting it).
- First, an object ‘myObject’ is created with two properties: ‘name’ and ‘age’.
- Then, ‘hasOwnProperty()’ method is called on ‘myObject’ with ‘name’ as an argument. As ‘name’ is indeed a property of ‘myObject’, the method returns ‘true’.
- Lastly, ‘hasOwnProperty()’ is called on ‘myObject’ with ‘address’ as an argument. Since ‘address’ is not a property of ‘myObject’, the method returns ‘false’.
#3 – By using the ‘propertyIsEnumerable()’ method
The ‘propertyIsEnumerable()’ method in JavaScript is used to check if a specific property in an object is enumerable or not. This basically means checking if the property can appear in a ‘for…in’ loop. To better understand, let’s take a look at an example.
var student = {
name: 'John',
age: 16
};
console.log(student.propertyIsEnumerable('name')); // true
console.log(student.propertyIsEnumerable('age')); // true
console.log(student.propertyIsEnumerable('toString')); // false
How it works
The ‘propertyIsEnumerable()’ method works by checking if the property is both present on the object and is set as enumerable. In JavaScript, object properties can be declared as non-enumerable, meaning they won’t be accessible in a ‘for…in’ loop and hence ‘propertyIsEnumerable()’ will return false.
- First, we define an object ‘student’ with properties ‘name’ and ‘age’.
- Then, we use the ‘propertyIsEnumerable()’ method on this object to check if the properties ‘name’, ‘age’, and ‘toString’ are enumerable or not.
- ‘propertyIsEnumerable()’ returns true for ‘name’ and ‘age’ because these properties are defined directly on the ‘student’ object and are enumerable by default.
- However, for ‘toString’, it returns false. That’s because ‘toString’ is a method inherited from the Object’s prototype, and it is not enumerable.
Related:
- How to Calculate Average of an Array in JavaScript
- How to Add Months to Dates in JavaScript
- How to Access Object Properties in JavaScript
In conclusion, checking for properties in a JavaScript object is a simple and essential skill in web development. By using the ‘hasOwnProperty’ method or the ‘in’ operator, we can easily determine if a specific property exists within our object.
Remember, every tool has its nuances. While ‘hasOwnProperty’ checks only for the object’s own properties, the ‘in’ operator checks through the entire prototype chain. Hence, understanding these differences can help create more efficient code.