How to Export Multiple Functions in JavaScript
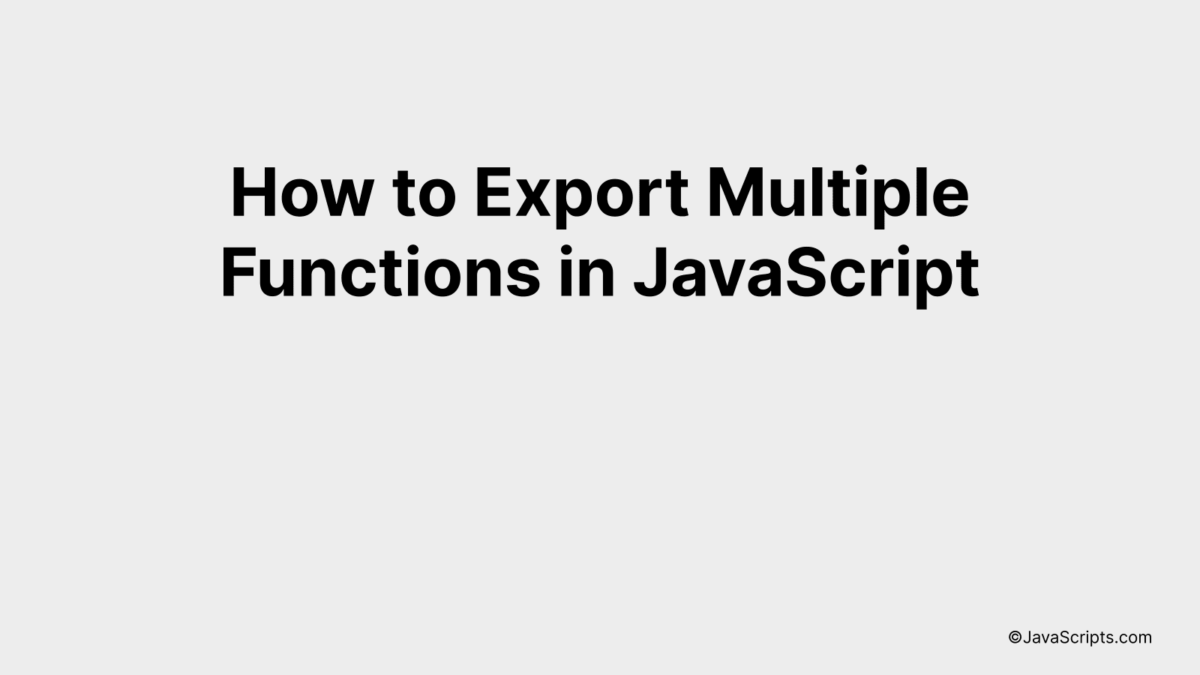
JavaScript’s versatility is one of its greatest strengths, allowing us to build dynamic and interactive web applications. A key aspect of this is the use of functions which, when properly managed, can significantly streamline our code.
Sharing these functions across different parts of the application or even across different applications is crucial. To achieve this, JavaScript provides us the ability to export multiple functions. Understanding this can be your stepping stone towards mastering efficient and modular coding.
In this post, we will be looking at the following 3 ways to export multiple functions in JavaScript:
- By using the Export Statement method
- By Assigning methods to module.exports object
- By using exports shortcut method.
Let’s explore each…
#1 – By using the Export Statement method
In JavaScript, we can use the “export” statement to export functions, objects or primitive values from a module so they can be used by other programs with the import statement. This enhances code modularity and reuse. Here’s an example where we export multiple functions.
// filename: sample.js
export function function1() {
return 'Function 1';
}
export function function2() {
return 'Function 2';
}
How it works
In the script above, we’ve declared two functions: function1 and function2. By using the ‘export’ keyword before each function declaration, we’re making these functions available to be imported by other modules or scripts.
- Step 1: We declare a function using the ‘function’ keyword followed by the name of the function. In our case, these are function1 and function2.
- Step 2: Each function is exported using the ‘export’ keyword. This makes the function accessible to other JavaScript files or modules.
- Step 3: Now, in any other JavaScript file, we can import these functions using the ‘import’ keyword followed by the name of the function and the relative path of the ‘sample.js’ file (assuming ‘sample.js’ is in the same directory as the file importing these functions).
#2 – By Assigning methods to module.exports object
In JavaScript, we can export multiple functions by attaching them as methods to the module.exports object. This will allow other modules to import and use these functions. The following example will help you understand this concept better.
// file: myModule.js
module.exports = {
function1: function() {
return "This is function1";
},
function2: function() {
return "This is function2";
}
};
How it works
In the code above, we’re creating an object with two methods – function1 and function2. This object is then assigned to module.exports. By doing this, we’re effectively saying that whenever this module is imported, these are the functions that will be exposed for use.
- The module.exports object is a special object which is included in every JS file in the Node.js application by default.
- We can add functions (or even variables) to this module.exports object that we want to make available for importing by other files.
- In the code above, we’ve added two functions – function1 and function2 – to the module.exports object.
- Now we can import this file myModule.js in another file using require(‘./myModule’). The imported module will be an object with function1 and function2 as its methods.
#3 – By using exports shortcut method.
In JavaScript, if you are working on a project that involves a modular structure, you can use the “exports” shortcut to export multiple functions or objects from one module so they can be used elsewhere. The following example will guide you through this process.
// script.js
exports.myFunction1 = function() {
return 'This is function 1';
}
exports.myFunction2 = function() {
return 'This is function 2';
}
How it works
The ‘exports’ object is a special object in Node.js that exposes functions or values from a module so that they can be imported and used in another module. It allows you to create a module of related code and functions, and expose only the parts you want to be public, keeping the rest private.
- Firstly, we define two functions, ‘myFunction1’ and ‘myFunction2’ in ‘script.js’.
- By prefixing these functions with ‘exports.’, we expose them so they can be imported into other modules.
- So when we import ‘script.js’ in another module, we have access to ‘myFunction1’ and ‘myFunction2’.
- This makes our code modular and easier to manage, as we can separate functionalities into different modules.
Related:
- How to Combine Two Objects in JavaScript
- How to Check if an Object Has a Property in JavaScript
- How to Calculate Average of an Array in JavaScript
In conclusion, exporting multiple functions in JavaScript can truly streamline your coding process. This strategy allows for a more organized, modular approach to writing code, thereby increasing efficiency and readability.
Remember, whether you’re using named or default exports, the key is to clearly specify what you’re exporting and effectively import them where required. This knowledge paves the way for better JavaScript coding practices.