How to Get the Domain from a URL in JavaScript
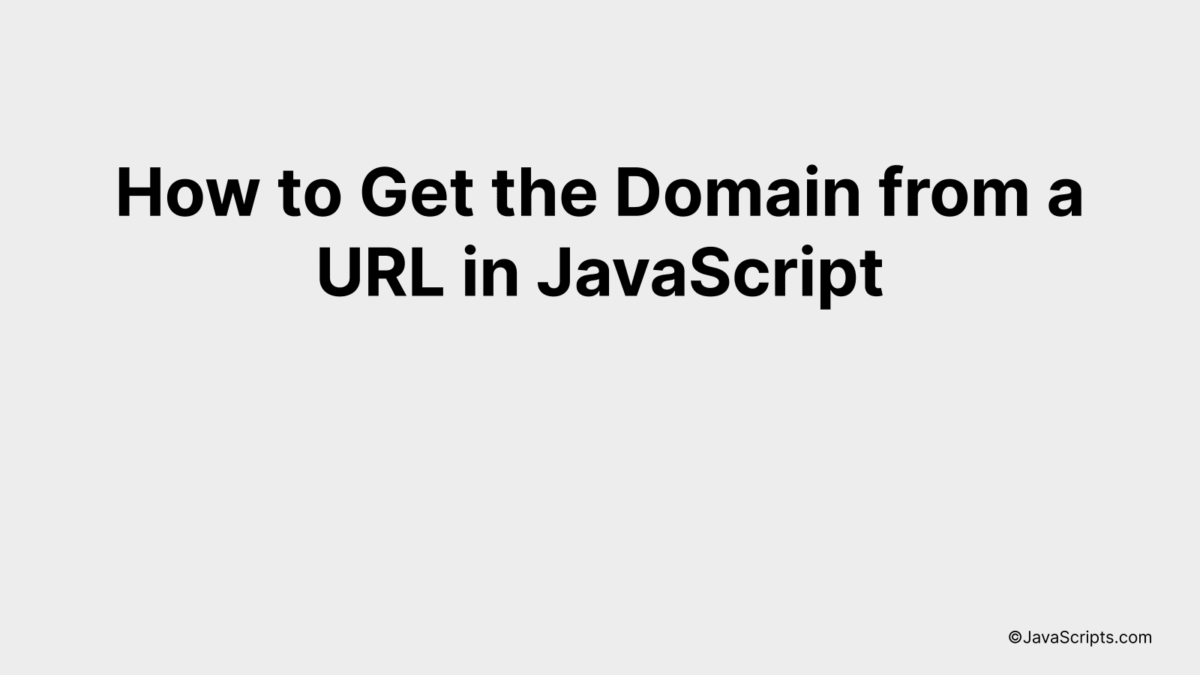
Navigating the realm of web development, you might often find yourself juggling with URLs. Whether you’re tracking site traffic or managing internal links, extracting the domain from a URL is a common task. This task could appear challenging, especially if you’re relatively new to JavaScript.
Don’t worry, we’ve got you covered. We’re going to unravel the mystery of how JavaScript can make this task a breeze for you. You’ll soon discover that with just a few lines of code, you can easily retrieve the domain from any given URL. It’s simpler than you might think.
In this post, we will be looking at the following 3 ways to get the domain from a URL in JavaScript:
- By using the URL API
- By using the anchor element in HTML
- By using the split() method in JavaScript
Let’s explore each…
#1 – By using the URL API
We are going to create a URL object from a string, then use the ‘hostname’ property to get the domain. For example, if the URL is ‘https://www.example.com/path’, the domain is ‘www.example.com’.
let url = new URL('https://www.example.com/path');
let domain = url.hostname;
How it works
This code works by using the built-in URL API in JavaScript. It parses the input string as a URL and exposes various parts of the URL (such as the domain) as properties.
- The ‘new URL()’ constructor is used to create a URL object from the input string. If the string is not a valid URL, it throws an exception.
- The ‘hostname’ property of the URL object is then accessed to get the domain. This includes the subdomain (if any), domain name, and domain extension.
#2 – By using the anchor element in HTML
We are going to create a HTML Anchor element virtually in JavaScript, assign the URL to its href attribute, and then use the hostname property of the anchor element to extract the domain. For instance, if our URL is “http://www.example.com/path?param=value”, the domain will be “www.example.com”.
let url = "http://www.example.com/path?param=value";
let a = document.createElement('a');
a.href = url;
let domain = a.hostname;
console.log(domain); // Outputs: "www.example.com"
How it works
This code utilizes the HTML Anchor element’s properties to extract the domain from a URL dynamically.
- First, the URL is assigned to the variable
url
. - The
document.createElement('a')
is used to create a new anchor element, which is stored in variablea
. - The URL is assigned to the
href
property of the anchor elementa
. - The
hostname
property of the anchor elementa
is used to extract the domain from the URL. It is assigned to the variabledomain
. - Finally, the domain is printed out using
console.log()
.
#3 – By using the split() method in JavaScript
This method involves splitting the URL at the slashes and using array indexing to retrieve the domain. For example, in the URL ‘http://example.com/path’, splitting at the slashes results in [‘http:’, ”, ‘example.com’, ‘path’], hence the domain is at index 2.
const url = "http://example.com/path";
const domain = url.split('/')[2];
How it works
The split() method in JavaScript is used to split a string into an array of substrings based on a specified separator, in this case, the slash (“/”). Since the domain is usually the third part of a URL (after ‘http:’ and an empty string ”), it is at index 2 of the resulting array. Hence, we use array indexing to retrieve the domain.
- The URL is defined as a string: “http://example.com/path”.
- The split(‘/’) function is called on this URL, splitting the string wherever a slash (“/”) appears. This results in an array: [‘http:’, ”, ‘example.com’, ‘path’].
- The domain name is then accessed by referring to the third item in this array (array index 2, as counting starts from 0 in JavaScript), which is ‘example.com’.
Related:
- How to Generate Random Numbers Between Two Numbers in JavaScript
- How to Find All Occurrences with JavaScript
- How to Extract Numbers from Strings in JavaScript
In conclusion, extracting a domain from a URL using JavaScript isn’t complex, but it’s a useful task. It’s about employing the right methods and understanding how URLs are structured. With the Web API’s URL interface, we can easily split the URL into its various components.
Keep practicing these techniques with whatever URLs you have. Remember, the more you practice, the more you’ll improve. Happy coding!