How to Read CSV Files in JavaScript
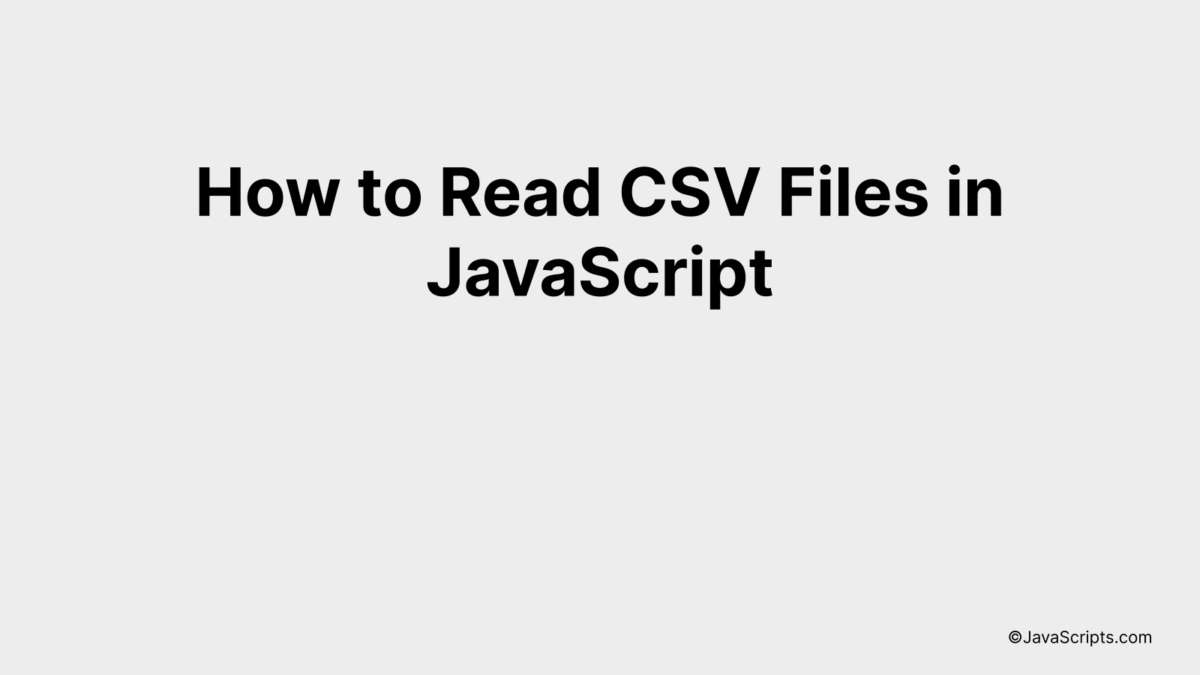
Reading CSV files in JavaScript might seem intimidating at first, but not to worry. You and I are about to embark on a journey that demystifies this process. We’ll explore the techniques together, making it clear and straightforward.
Do you know that JavaScript provides robust tools to manage CSV files efficiently? Yes, it does. So, let’s roll up our sleeves and start unlocking the power of handling CSV files using one of the most popular programming languages—JavaScript.
In this post, we will be looking at the following 3 ways to read CSV files in JavaScript:
- By using the Papa Parse library
- By using the d3.js library
- By using the FileReader API
Let’s explore each…
#1 – By using the Papa Parse library
We are going to read CSV files in JavaScript using the Papa Parse library, which is a powerful and flexible tool for parsing CSV data. We’ll first fetch the CSV file, parse it using Papa Parse, and then manipulate the parsed data according to our needs. To understand this better, we’ll go through an illustrative example.
var Papa = require('papaparse');
var file = 'path_to_your_file.csv';
Papa.parse(file, {
download: true,
header: true,
complete: function(results) {
console.log("Completed:", results.data);
}
});
How it works
The above code is using the Papa Parse library to read a CSV file and transform it into a usable data structure within JavaScript. The parse method is invoked with the file path and an options object as arguments, which details how the parsing should be conducted.
- Step 1: We require the Papa Parse library using the require function and store it into the variable named ‘Papa’.
- Step 2: We specify the path to our CSV file and store it in the variable ‘file’.
- Step 3: We call the parse method on the ‘Papa’ object with two arguments. The first argument is the ‘file’ variable that holds our file path. The second argument is an options object.
- Step 4: In the options object, we set ‘download’ to true to tell Papa Parse to go to the path specified in the ‘file’ variable and download the CSV data.
- Step 5: We set ‘header’ to true to tell Papa Parse that the first row of the CSV file contains the column names.
- Step 6: We define a ‘complete’ function that will be executed once the parsing is finished. This function receives the parsed data as an argument. In this function, we simply log the parsed data to the console.
#2 – By using the d3.js library
We will be using the d3.js library to read a CSV file in JavaScript. This can be easily understood by using a practical example of code where we load a specific CSV file and log the first row to the console.
d3.csv("https://example.com/data.csv").then(function(data) {
console.log(data[0]);
}).catch(function(error){
console.log(error);
});
How it works
This code uses d3.js library’s d3.csv() function to load a CSV file from a provided URL. The function returns a promise that resolves to an array of objects when the CSV file is successfully loaded. If there’s an error during the process, it is logged to the console.
- Step 1: The d3.csv() function is called with the URL of the CSV file as an argument. This function initiates a request to the provided URL to fetch the CSV file.
- Step 2: The d3.csv() function returns a promise. Using the then function, we handle the case when the promise is fulfilled (i.e., the CSV data is successfully loaded).
- Step 3: Inside the then function, we log the first row of the CSV file to the console using console.log(data[0]);. Here data is an array of objects, where each object corresponds to a row in the CSV file and the properties of the object correspond to the column names.
- Step 4: The catch function handles any errors that occur during the request. If an error occurs, it is logged to the console using console.log(error);
#3 – By using the FileReader API
This method involves using the FileReader API in JavaScript, which allows web applications to read the contents of files (or raw data buffers) stored on the user’s computer, using File or Blob objects to specify the file or data to read.
Here’s an example of how to do it:
const fileInput = document.getElementById('csv');
const reader = new FileReader();
fileInput.addEventListener('change', (e) => {
let file = e.target.files[0];
reader.onload = function(e) {
let contents = e.target.result;
let lines = contents.split('n');
lines.forEach(line => {
console.log(line);
});
};
reader.readAsText(file);
});
How it works
The FileReader API reads the contents of a file from your device. When a CSV file is selected, the FileReader reads its contents and splits it into lines, which are then logged to the console.
- First, we get the file input field from the HTML using document.getElementById(‘csv’) and create an instance of FileReader.
- Then we add an event listener to the file input. This event listener triggers when a user selects a file in the file input field.
- Inside the event listener, we get the file selected by the user with e.target.files[0].
- We define a function to execute when the FileReader finishes loading the file. This function logs the contents of the file to the console.
- Finally, we tell the FileReader to read the file as text with reader.readAsText(file). This triggers the load event when it finishes reading the file, which in turn triggers the function we defined.
Related:
- How to Print Variables in JavaScript
- How to Get the Last Character of a String in JavaScript
- How to Flatten Objects in JavaScript
In conclusion, reading CSV files in JavaScript is a handy ability for any web developer. It involves using either the FileReader API, which reads files stored on the user’s computer, or the Papa Parse library, which is a powerful and flexible solution for parsing CSV data.
To wrap it up, remember that understanding and properly implementing these methods can greatly enhance your application’s data processing capabilities. Don’t be afraid to experiment and refine your skills – practice makes perfect!