How to Replace All Spaces in JavaScript
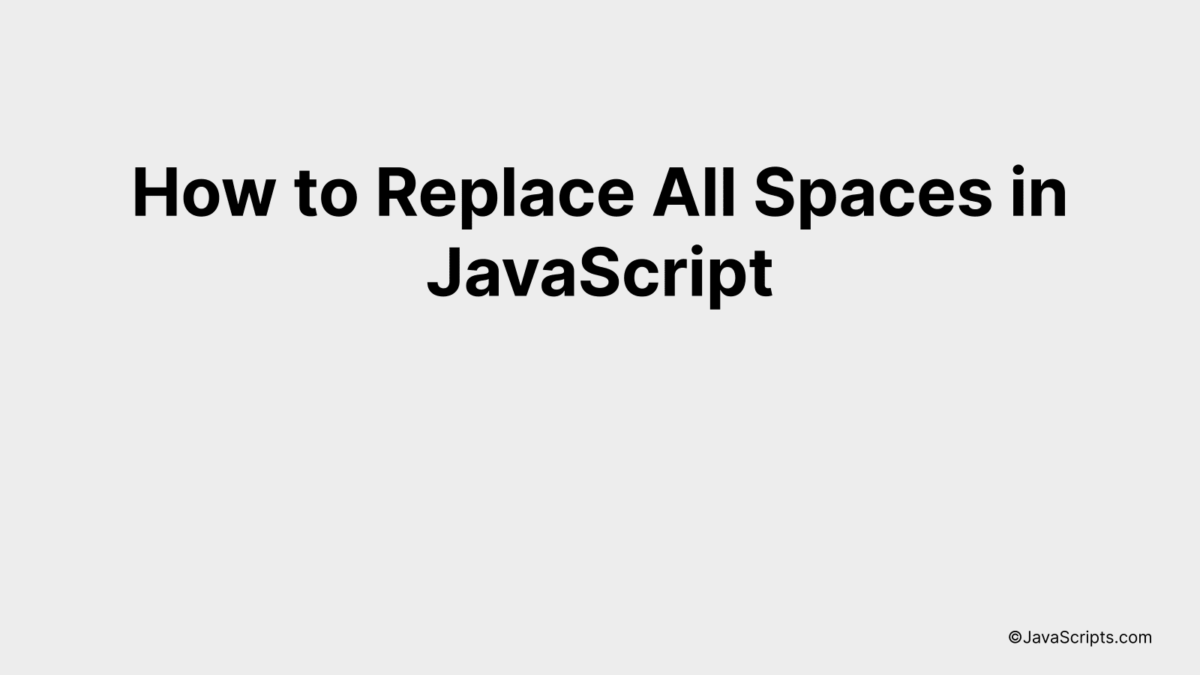
Manipulating strings in JavaScript is an essential skill, regardless of whether you’re a novice coder or a seasoned programmer. One common scenario you might encounter is needing to replace all spaces in a string. This task can seem challenging, but don’t worry! It’s simpler than you might think.
Together, we’ll explore a few effective methods to accomplish this goal. We’ll go over the use of built-in JavaScript methods like ‘replace()’ and ‘split()’ along with regular expressions. By the end of our journey, you’ll be able to handle spaces in strings with ease and confidence.
In this post, we will be looking at the following 3 ways to replace all spaces in JavaScript:
- By using the replace() method with a regular expression
- By using the split() and join() methods
- By using the replaceAll() method
Let’s explore each…
#1 – By using the replace() method with a regular expression
This task will involve using JavaScript’s built-in replace()
method with a regular expression (regex) to globally replace all spaces in a string with a defined substitute. For example, we can replace all spaces in the string “Hello World” with underscores “_”, resulting in “Hello_World”.
var str = "Hello World";
var newStr = str.replace(/s/g, "_");
How it works
The replace()
method in JavaScript is used to replace a specified value with another value in a string. When combined with regular expressions, it becomes a powerful tool for string manipulation. The /s/g
in the code is a regular expression that matches any white space character, and ‘g’ is the global flag which means to replace all occurrences found.
- We first define a string using
var str = "Hello World";
. This is the string we will be manipulating. - Next, we use
str.replace(/s/g, "_");
, thereplace()
method of the string. This method takes two parameters: the value to find (in our case, any whitespace), and the value to replace it with. - In the parameters,
/s/g
is a regular expression that matches any whitespace character. The/s/
is the whitespace character class in regex, and theg
after the second slash is a flag that tells the method to replace all occurrences of whitespace, not just the first one it finds. - The result of the replace method is a new string, which we store in
var newStr
. This string will have all spaces replaced with underscores.
#2 – By using the split() and join() methods
The split() and join() methods in JavaScript can be used to replace all spaces in a string. This is done by splitting the string into an array of substrings without spaces using split() method, then joining all the substrings into a single string with no spaces by using the join() method. Here is an example:
let string = "Hello World!";
let replacedString = string.split(" ").join("");
How it works
The provided JavaScript code first splits the input string wherever it encounters a space, creating an array of substrings. It then joins these substrings back together without any spaces, effectively replacing all spaces in the original string.
- The split() method is used to divide a string into an ordered list of substrings, and returns this new array. In this code, split(” “) is used to separate the string wherever there is a space.
- The join() method is then used to combine all the elements of the resulting array into a single string. Without specifying a separator in the join() method, it defaults to comma. However, in this code, join(“”) is used to specify that no character is used to separate the substrings in the resulting string. This effectively replaces all the spaces in the original string.
#3 – By using the replaceAll() method
The JavaScript replaceAll() method will replace all instances of a specified value (in this case, spaces) in a string. This can be better understood by considering a string “Hello World”, where we aim to replace all the spaces with hyphens (-), resulting in “Hello-World”.
let str = "Hello World";
let newStr = str.replaceAll(' ', '-');
console.log(newStr); // Outputs: "Hello-World"
How it works
The replaceAll() method scans the entire input string and replaces each instance of the specified value (a space in our case) with the new value (a hyphen in our case). It then returns the modified string.
- Step 1: Declare a string variable ‘str’ with the value “Hello World”.
- Step 2: Call the replaceAll() method on ‘str’, specifying a space (‘ ‘) as the value to be replaced and a hyphen (‘-‘) as the replacement value. The resulting string is stored in the new variable ‘newStr’.
- Step 3: Log the value of ‘newStr’ to the console. The output will be “Hello-World”, which is the original string with all spaces replaced by hyphens.
Related:
- How to Print DIVs with JavaScript
- How to Perform Reverse Shell in JavaScript
- How to Perform Merge Sort in JavaScript
In conclusion, JavaScript provides clear and straightforward methods to replace spaces in strings, enhancing your code’s efficiency and readability. The ‘replace()’ method with a global flag (‘g’) is a powerful tool to accomplish this task.
Practice makes perfect, so don’t hesitate to play around with these techniques. Comfort with string manipulation, such as replacing spaces, is a vital skill for any coder. Remember, patience and curiosity are key in your coding journey.