How to Translate Text with JavaScript
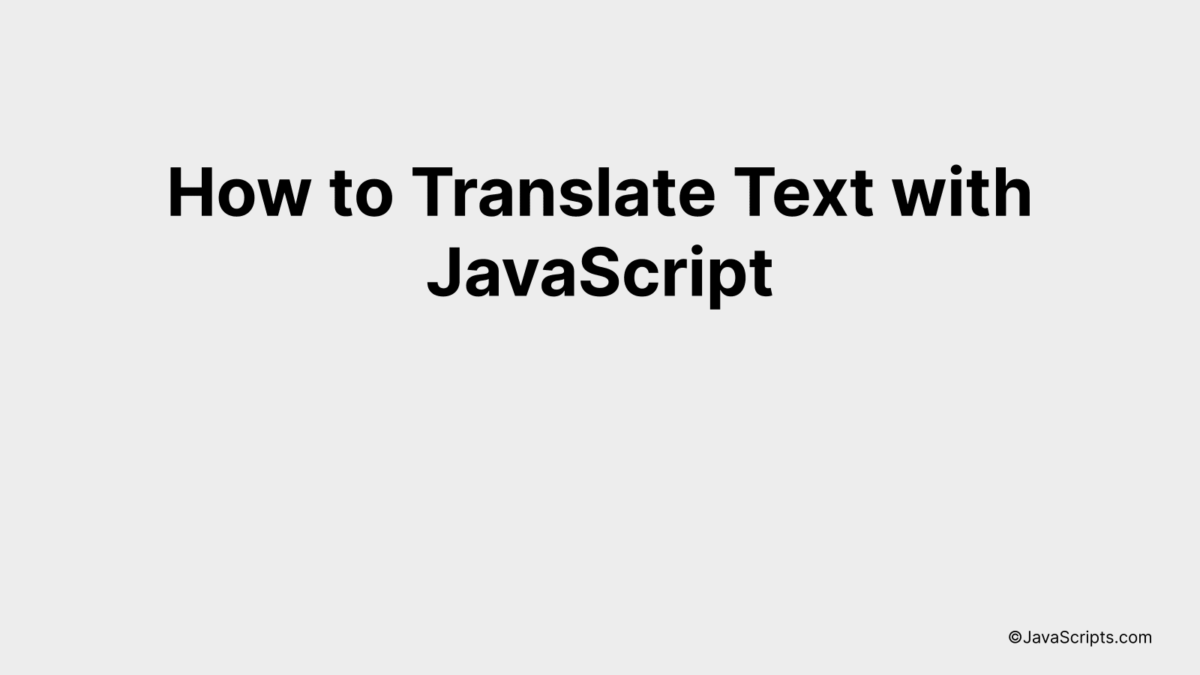
Ever wondered how to cross language barriers on the web using a tool you’re already familiar with? You’re in the right place. We’re about to explore the fascinating world of translating text using JavaScript, one of the most popular languages in web development.
You and I will step into the shoes of a linguist, armed with nothing but lines of code. Picture this: a webpage dynamically translating text, all with the power of JavaScript. Sounds exciting, right? Let’s dive into the magic that makes this possible.
In this post, we will be looking at the following 3 ways to translate text with JavaScript:
- By using Google Cloud Translation API
- By leveraging Microsoft Azure Text Translation API
- By implementing libraries like i18next or jquery-i18n
Let’s explore each…
#1 – By using Google Cloud Translation API
This process involves using Google Cloud Translation API in JavaScript to translate a given text from one language to another. For instance, translating ‘Hello World’ from English to Spanish.
// Import the Google Cloud client library
const {Translate} = require('@google-cloud/translate').v2;
// Your Google Cloud Platform project ID
const projectId = 'YOUR_PROJECT_ID';
// Instantiates a client
const translate = new Translate({projectId});
// The text to translate
const text = 'Hello, world!';
// The target language
const target = 'es';
// Translates some text into the target language
async function translateText() {
const [translation] = await translate.translate(text, target);
console.log(Text: ${text});
console.log(Translation: ${translation});
}
translateText();
How it works
The Google Cloud Translation API is a powerful tool that allows you to translate text from one language to another with a simple HTTP request. By using this API in JavaScript, you can build multilingual applications and services that are capable of dynamically translating text in real-time or in batch mode.
- Step 1: We begin by importing the Google Cloud client library.
- Step 2: We then specify the Google Cloud Platform project ID.
- Step 3: After that, we instantiate a new client using our project ID.
- Step 4: We define the text that we want to translate.
- Step 5: We specify the target language to which we want to translate the text.
- Step 6: We create a function that uses the client to translate the text into the target language.
- Step 7: The translated text is then logged to the console for review.
#2 – By leveraging Microsoft Azure Text Translation API
This task involves using the Microsoft Azure Text Translation API within a JavaScript application to translate text from one language to another; for instance, translating English text into Spanish.
const axios = require('axios');
const subscriptionKey = 'your_subscription_key';
let translateText = async (text, toLanguage) => {
const url = https://api.cognitive.microsofttranslator.com/translate?api-version=3.0&to=${toLanguage};
const headers = {
'Ocp-Apim-Subscription-Key': subscriptionKey,
'Ocp-Apim-Subscription-Region': 'your_region',
'Content-type': 'application/json',
'X-ClientTraceId': uuidv4().toString()
};
const body = [{ 'Text': text }];
const response = await axios.post(url, body, { headers });
return response.data[0].translations[0].text;
};
translateText('Hello World!', 'es').then(translation => console.log(translation)).catch(err => console.log(err));
How it works
The above code uses the Microsoft Azure Text Translation API to translate text. It sends a POST request to the API with the text to be translated and the target language, and then retrieves the translated text from the response.
- We first import the axios library for sending HTTP requests and declare the subscriptionKey which is your Azure subscription key.
- We then define an asynchronous function translateText that takes in the text to translate and the target language as arguments.
- We construct the URL for the API endpoint. The to query parameter in the URL indicates the target language to which the text will be translated.
- We define the headers for our request. This includes the subscription key and region, the content type, and a randomly generated client trace ID.
- We then create a JSON body, which includes the text to be translated.
- We send a POST request to the API using axios’s post method, passing in the URL, body and headers.
- The API responds with a JSON object, from which we extract the translated text.
- Finally, we call the translateText function with the text ‘Hello World!’ and the target language ‘es’ (Spanish) and output the translated text. If an error occurs, it will be logged to the console.
#3 – By implementing libraries like i18next or jquery-i18n
Here’s an example of using i18next library for translating text in JavaScript:
By using i18next library, we are going to translate a given text into a selected language, for instance, translating “Hello” to French.
// Importing i18next
const i18n = require('i18next');
// Initializing i18next
i18n.init({
lng: 'fr', // Set the language to French
resources: {
fr: {
translation: {
"Hello": "Bonjour" // Translation mapping
}
}
}
});
// Use t function to translate text
console.log(i18n.t('Hello')); // Outputs: Bonjour
How it works
The i18next library allows you to translate text in JavaScript by setting a specified language and providing translation resources. The ‘t’ function is then used on the text you want to translate.
Step-by-step explanation:
- First, we import the i18next library using the
require
command. - Next, we initialize i18next with the
init
function. Inside this function, we set the language to French withlng: 'fr'
and provide the translation resources. - In the resources, we set ‘fr’ as a key and provide an object that contains the translation mapping. In the example, we mapped “Hello” to “Bonjour”.
- Finally, we use the
t
function provided by i18next on the text we want to translate, which in this case is ‘Hello’. As a result, it outputs ‘Bonjour’.
Related:
- How to Subtract Days from Dates in JavaScript
- How to Read CSV Files in JavaScript
- How to Print Variables in JavaScript
In conclusion, translating text with JavaScript has never been so smooth and straightforward. By employing APIs like Google Translate API or libraries like i18next, you can quickly translate text in numerous languages with just a few lines of code.
Remember, efficient web communication is all about breaking language barriers. With JavaScript, you’re just a few steps away from creating a more inclusive and accessible web environment. Happy coding!