How to Update Objects in Arrays with JavaScript
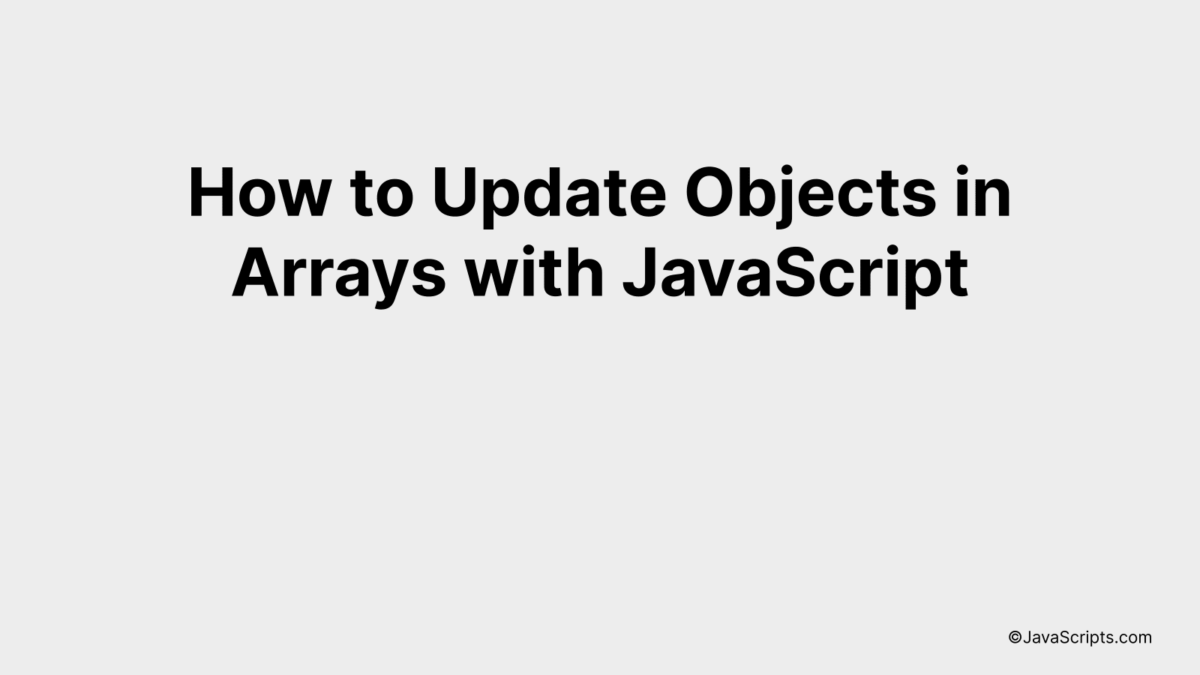
Are you a JavaScript enthusiast, or a beginner seeking to understand the nuances of updating objects in arrays? You’ve come to the right spot! We’ll untangle this concept together, making sure you get a solid grasp over it.
Imagine you’re standing in front of a wardrobe, looking for that perfect outfit. An array in JavaScript is just like that wardrobe, filled with different objects (outfits). Now, updating these objects is akin to customizing your clothes. We’re going to learn how to do so, with practical examples and easy-to-understand language.
In this post, we will be looking at the following 3 ways to update objects in arrays with JavaScript:
- By using the array map() function
- By direct index assignment
- By using the array forEach() method
Let’s explore each…
#1 – By using the array map() function
In JavaScript, you can use the array map() function to create a new array by applying a function to each element of an existing array. This method is particularly helpful when you need to update objects within an array. Let’s understand this concept with an example.
const fruits = [
{ name: 'apple', color: 'red' },
{ name: 'banana', color: 'yellow' },
{ name: 'grape', color: 'purple' }
];
const updatedFruits = fruits.map(fruit => {
if (fruit.name === 'banana') {
return { ...fruit, color: 'green' };
} else {
return fruit;
}
});
console.log(updatedFruits);
How it works
In the above example, we used the map function to create a new array of fruit objects. If the name of the fruit is ‘banana’, we change its color to ‘green’, otherwise, we keep the fruit object as it is.
- First, we define an array of fruit objects, each with a ‘name’ and ‘color’ property.
- Next, we call the ‘map’ function on the ‘fruits’ array. The ‘map’ function takes a callback function as an argument, which we use to specify the transformation we want to apply to each element in the array.
- In our callback function, we check whether the name of the current fruit object is ‘banana’.
- If it is, we return a new object that includes all properties of the current fruit object (using the spread operator ‘…’) and updates the color property to ‘green’.
- If the name of the fruit is not ‘banana’, we simply return the fruit object as it is.
- The ‘map’ function uses our callback function to create a new array, which we assign to the ‘updatedFruits’ variable.
- Finally, we log ‘updatedFruits’ to the console to inspect our updated array.
#2 – By direct index assignment
Updating objects in arrays by direct index assignment in JavaScript involves directly accessing the desired index of the array and then updating the object’s properties using property assignment. This method is efficient and straightforward, demonstrated with an example for clarity.
let users = [{ name: 'John', age: 20 }, { name: 'Sara', age: 22 }];
users[1].age = 25;
How it works
The above code updates the ‘age’ property of the second object in the ‘users’ array. It accomplishes this by accessing the array index directly and assigning a new value to the property ‘age’.
- First, an array of objects is declared and initialized, with each object containing properties ‘name’ and ‘age’.
- Next, the array ‘users’ is accessed directly at index 1. Remember, array indices in JavaScript start at 0, so index 1 refers to the second object in the array.
- After accessing the desired object, the ‘age’ property of that object is updated by assigning it a new value (25 in this case).
- The end result is an updated ‘users’ array where the second user’s age has been changed from 22 to 25.
#3 – By using the array forEach() method
The JavaScript array forEach() method iterates over each item in an array and allows us to manipulate or update each object based on our requirements. It’s very useful for traversing arrays, and this can be better understood with a practical example.
var cars = [
{ brand: 'Toyota', color: 'red' },
{ brand: 'BMW', color: 'white' },
{ brand: 'Audi', color: 'black' }
];
cars.forEach(function(car, index) {
car.color = 'blue';
car.index = index;
});
How it works
The forEach() method goes through each item in the ‘cars’ array. For each item (object), we change the ‘color’ property to ‘blue’ and additionally add an ‘index’ property with the current index value.
- First, we initialize an array of objects named ‘cars’, each having properties ‘brand’ and ‘color’.
- The forEach() method is then called on the ‘cars’ array. The function provided as an argument to forEach() is executed for each item in the array.
- Inside the function, we manipulate each ‘car’ object by changing its ‘color’ property to ‘blue’.
- We also add a new property ‘index’ to each ‘car’ object, assigning it the current index value.
- The forEach() method doesn’t return a new array, but modifies the original array ‘cars’ directly.
Related:
- How to Simulate Keypress Events in JavaScript
- How to Set Sizes with JavaScript
- How to Replace All Spaces in JavaScript
In conclusion, updating objects in arrays with JavaScript is a straightforward process. With the use of methods like ‘findIndex’, ‘map’, ‘forEach’, we can easily manipulate array objects. It’s all about understanding how these methods work and when best to use them.
Remember, practice makes perfect. So, it’s advisable to consistently work on your JavaScript skills. With time, your confidence in handling complex tasks would definitely increase. Happy coding!