How to Use CamelCase in JavaScript
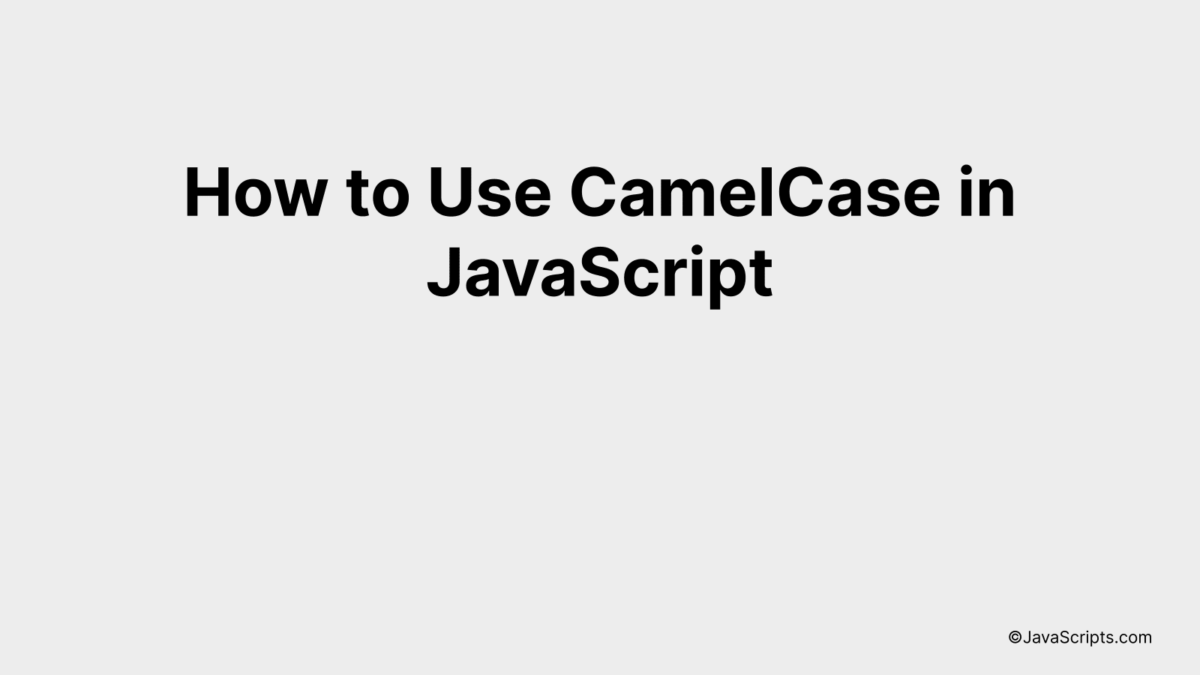
You’ve probably seen JavaScript variables or functions named like ‘myFirstVariable’ or ‘calculateTotalSum’. Ever wondered why they’re written in this peculiar way? This is what we call CamelCase, a popular naming convention across many programming languages, especially JavaScript.
You and I will dive into this fascinating world of CamelCase. Together, we’ll discover its significance, how to use it effectively, and why it’s a beloved norm among JavaScript developers. It’s simple, efficient, and once you’ve mastered it, you’ll find your code much easier to read and understand.
In this post, we will be looking at the following 3 ways to use camelcase in JavaScript:
- By using the replace() method with a regular expression
- By using the split() and map() methods
- By using a third-party library like lodash’s _.camelCase() function
Let’s explore each…
#1 – By using the replace() method with a regular expression
In JavaScript, you can convert a string to camelCase by using the replace() method with a regular expression. This approach involves finding space-separated words or dash-separated words and converting the initial letter of each subsequent word into uppercase while keeping the first word all lowercase.
const convertToCamelCase = (str) => {
return str.replace(/(?:^w|[A-Z]|bw|s+)/g, function(match, index) {
if (+match === 0) return "";
return index == 0 ? match.toLowerCase() : match.toUpperCase();
});
};
console.log(convertToCamelCase("hello world")); // output: helloWorld
console.log(convertToCamelCase("hello-world")); // output: helloWorld
How it works
The provided JavaScript function, convertToCamelCase, takes a string as an argument and employs the replace() method along with a regular expression to adjust letter casing and form a camelCased string.
- The replace() method is invoked on the input string. It takes a regular expression as the first argument, which matches every word boundary, every standalone word character, and every whitespace. The second argument is a function that gets called for each match.
- The function passed as the second argument to the replace() method takes the current match and its index as arguments.
- If the match equates to 0 (which happens for whitespaces and dashes due to the + operator), the function returns an empty string, effectively removing the match from the final output.
- If the match is at index 0 (which indicates the first word), it is converted to lowercase.
- If the match is not at index 0, indicating it’s a subsequent word, it’s converted to uppercase. This effectively capitalizes the first letter of each word after the first one.
- The result is a string in camelCase format.
#2 – By using the split() and map() methods
We will convert a sentence into camelCase in JavaScript by first splitting the sentence into words using the split() method, and then converting the first letter of every word, except the first word, to uppercase using the map() method. For instance, if we have the string “hello world”, the output will be “helloWorld”.
let sentence = "hello world";
let camelCase = sentence.toLowerCase().split(' ').map((word, index) => index !== 0 ? word.charAt(0).toUpperCase() + word.slice(1) : word).join('');
How it works
The split() method breaks the sentence into individual words, and the map() method then iterates over these words. If the word is not the first word in the sentence (index !== 0), it converts the first character of the word to uppercase and then adds the rest of the word. The words are then joined back together without spaces to form a camelCased string.
- The toLowerCase() method converts the entire string to lowercase to ensure consistency.
- The split(‘ ‘) method splits the string into an array of words.
- The map() function loops through this array of words.
- In the map() function, for every word except the first one (index !== 0), the first character is capitalized using charAt(0).toUpperCase() and the rest of the word is appended using slice(1).
- If it’s the first word (index === 0), it remains in lowercase, as per camelCase convention.
- The join(”) method then joins all the words together, without spaces, forming a camelCased string.
#3 – By using a third-party library like lodash’s _.camelCase() function
The _.camelCase() function from lodash library in JavaScript converts a string to camel case. This is best demonstrated with an example where we convert a sentence with spaces or underscores to camel case.
// Import lodash library
const _ = require('lodash');
// The string to be converted
let myStr = "Hello_world this is a test-string";
// Use lodash's camelCase function
let result = _.camelCase(myStr);
// Output the result
console.log(result); // Outputs: 'helloWorldThisIsATestString'
How it works
The _.camelCase() function from lodash library takes a string as input and returns the string in camel case. It removes any spaces or special characters and starts each word after the first one with a capital letter.
- First, lodash’s _.camelCase() function is called with a string as parameter.
- The function internally splits the input string into words. It considers spaces, underscores, or hyphens as word separators.
- It then capitalizes the first letter of each word except the first one and makes the first letter of the first word lowercase. This forms the camelcase standard.
- All the words are then joined together without spaces to form the final camelCase string.
- The function then returns this camelCase string.
Related:
- How to Translate Text with JavaScript
- How to Subtract Days from Dates in JavaScript
- How to Read CSV Files in JavaScript
In conclusion, CamelCase is a powerful naming convention used in JavaScript. It’s simple to implement and helps with code readability. Both programmers and machines find this format easy to understand, making code maintenance less challenging.
Remember, the first letter of the first word is lowercase, while the first letter of each subsequent word is uppercase. These simple rules can make your JavaScript coding more efficient and your code much more user-friendly.