How to Use Curly Braces in JavaScript
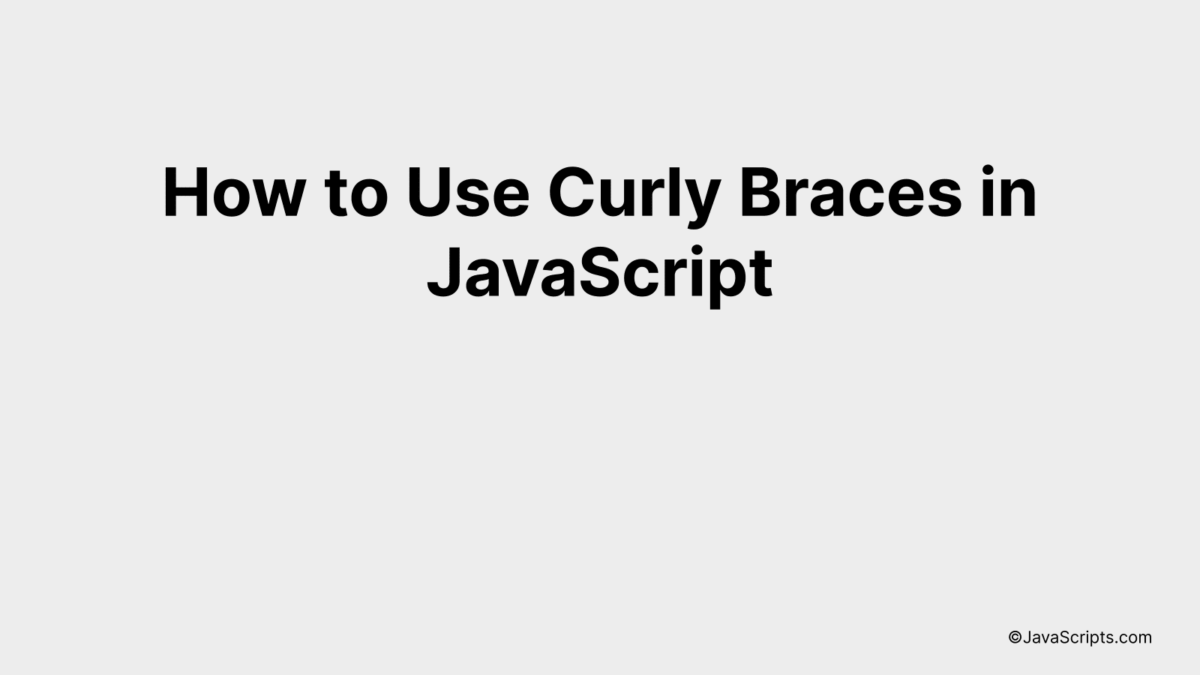
JavaScript, often simply referred to as JS, is an essential tool in your coding arsenal. Its power and versatility lie in its many features, one of which is the use of curly braces. These seemingly insignificant symbols play a vital role in structuring your code and facilitating complex operations.
Have you ever wondered why curly braces are so ubiquitous in JavaScript code? Or perhaps you are curious about how to use them more effectively? Let’s embark on a journey to demystify these curly wonders, as understanding them can pave the way to cleaner and more efficient code.
In this post, we will be looking at the following 3 ways to use curly braces in JavaScript:
- By defining an Object Literal
- By creating a Block of code
- By using Template Literals
Let’s explore each…
#1 – By defining an Object Literal
In JavaScript, an Object Literal is a way to define a collection of data where each value can be accessed via a unique identifier (property name). It is defined using curly braces and can help to organize and manage your data more effectively. Let’s look at an example to understand this better.
let student = {
name: "John",
age: 25,
course: "Computer Science",
getDetails: function() {
return this.name + ", " + this.age + ", " + this.course;
}
};
How it works
The above code creates an object ‘student’ with properties ‘name’, ‘age’, ‘course’, and a method ‘getDetails’. The method can be accessed like any other property.
- Step 1: We create an object ‘student’ using curly braces ‘{}’.
- Step 2: Inside this object, we define properties ‘name’, ‘age’, ‘course’ and each is separated by a comma. The properties are assigned values using the colon ‘:’ symbol.
- Step 3: We also define a method ‘getDetails’. In JavaScript, methods are functions that are stored as object properties. Here ‘getDetails’ is a method that returns the student’s details as a string.
- Step 4: The keyword ‘this’ is used within the method to refer to the current object, which is ‘student’ in our case. Thus ‘this.name’, ‘this.age’, ‘this.course’ refer to the properties of the ‘student’ object.
#2 – By creating a Block of code
In JavaScript, curly braces {} are used to define a block of code. A block of code is a set of instructions that are executed together when the block is invoked. This can be better understood with an example.
function greet() {
let greeting = 'Hello';
{
let name = 'John';
console.log(greeting + ', ' + name);
}
}
greet();
How it works
This block of code defines a function named ‘greet’. Within this function, a string ‘Hello’ is stored in a variable ‘greeting’. Then, a block of code is defined using curly braces, where another variable ‘name’ is defined and assigned the value ‘John’. The console.log statement inside this block combines ‘greeting’ and ‘name’ to output ‘Hello, John’. The function ‘greet’ is then invoked to execute this block of code.
- The ‘function’ keyword is used to define a function named ‘greet’.
- Inside this function, we define a variable ‘greeting’ using the ‘let’ keyword and assign it the value ‘Hello’.
- Next, we define a block of code using curly braces {}. Anything within these curly braces forms part of this block and is executed together.
- Inside this block, we define a variable ‘name’ and assign it the value ‘John’.
- We then use a console.log statement to output the combination of ‘greeting’ and ‘name’, which is ‘Hello, John’.
- Finally, outside the function definition, we invoke the function ‘greet’ by writing ‘greet()’. This executes all the code within the function’s definition.
#3 – By using Template Literals
In JavaScript, template literals are a way to output variables within a string. These are enclosed by the back-tick ( ) (grave accent) character instead of double or single quotes and variables you want to output within a string are enclosed within curly braces preceded by a dollar sign (${}).
let name = 'John';
let greeting = Hello, ${name}!;
console.log(greeting);
How it works
Template literals in JavaScript offer a powerful and convenient way to embed variables and expressions into strings. The primary purpose is to make it easier to use dynamic data in strings.
The script works as follows:
- We first define a variable name and assign it the value ‘John’.
- We then define a variable greeting to store our template literal. Within this template literal, we include the name variable within ${}.
- JavaScript recognizes the ${} syntax within the template literal and interprets it as an expression. It evaluates the expression (in this case a simple variable, name), converts the result to a string and embeds it within the resulting string.
- Finally, the script prints the result to the console using console.log(greeting). This will output Hello, John! to the console.
Related:
- How to Use CamelCase in JavaScript
- How to Translate Text with JavaScript
- How to Subtract Days from Dates in JavaScript
In closing, curly braces in JavaScript play a vital role. They embrace blocks of code in functions, conditionals, and loops, creating order and clarity. Their correct use makes your code functional and easy to understand.
Remember, consistency in using curly braces can improve readability and reduce potential errors in your code. Practice makes perfect, so keep coding and experimenting with curly braces in different scenarios.