How to Add Key-Value Pairs to Objects in JavaScript
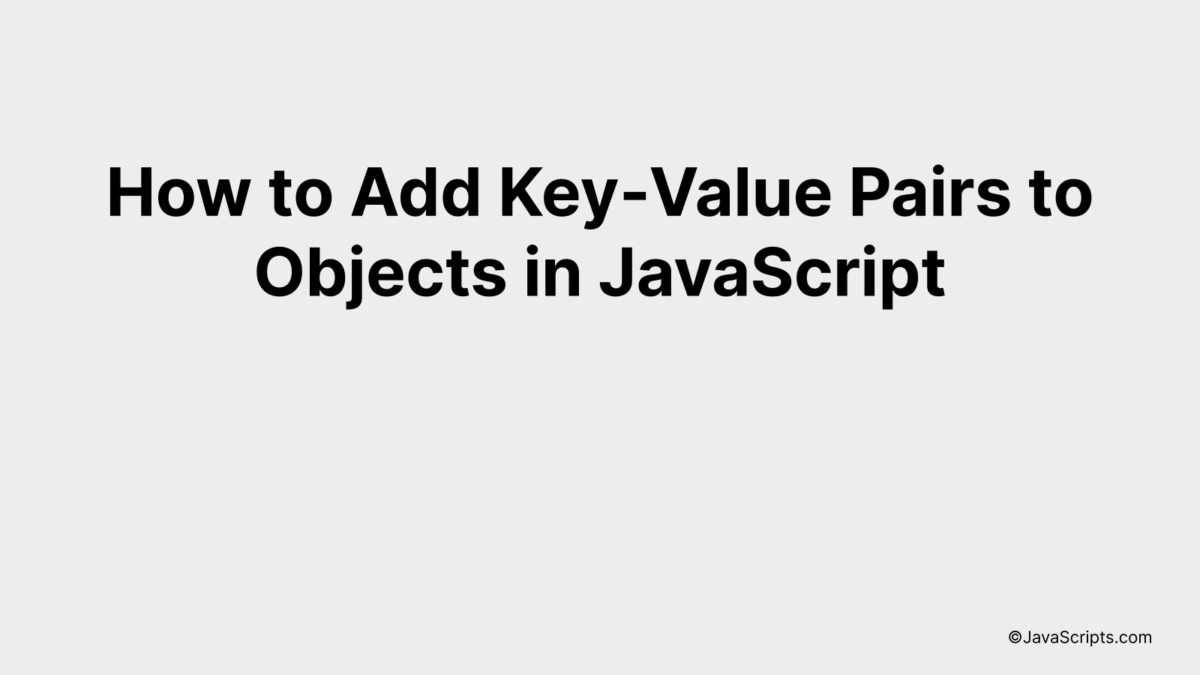
JavaScript stands as a pillar of modern web development, driving the dynamic, interactive websites that we interact with daily. Among its many features, JavaScript’s object-oriented structure offers a powerful way to organize and manipulate data.
You might be familiar with objects in JavaScript, a versatile data structure that allows the storage of multiple values in a single variable. This guide is all about interesting ways to add key-value pairs to these objects, a task that’s essential but might sometimes seem a bit tricky. Let’s dive in and explore this together!
In this post, we will be looking at the following 3 ways to add key-value pairs to objects in JavaScript:
- By using Dot notation
- By using Bracket notation
- By using Object.assign() method
Let’s explore each…
#1 – By using Dot notation
In JavaScript, you can add key-value pairs to an object using dot notation by just specifying the object name, followed by a dot, the key name, an equal sign, and the value. Here’s an example:
var myObject = {};
myObject.key = "Value";
How it works
Dot notation in JavaScript allows us to access or set the properties of objects. In the above example, an empty object is created and a new key-value pair is added to it using dot notation.
- First, an empty object, ‘myObject’, is created.
- Then, dot notation is used to add a new property (or key), ‘key’, to the object. This is done by writing ‘myObject.key’.
- The equal sign is used to assign a value to this key. In this case, the string ‘Value’ is assigned to ‘key’.
- Now, ‘myObject’ has one property, ‘key’, which holds the value ‘Value’.
#2 – By using Bracket notation
In JavaScript, bracket notation enables you to add key-value pairs to objects dynamically. This is particularly useful when the property names are not known until runtime. Here’s an example:
let obj = {};
let key = 'property1';
let value = 'value1';
obj[key] = value;
How it works
What this code does is create an empty object (obj), defines a key (property1) and a value (value1), and then uses bracket notation to add this key-value pair to the object.
- Step 1: An empty object obj is initialized. So, obj = {}.
- Step 2: The key and value are defined. Here, key = ‘property1’ and value = ‘value1’.
- Step 3: The key-value pair is added to the object using bracket notation. obj[key] = value is equivalent to obj[‘property1’] = ‘value1’. This adds a new property ‘property1’ to the object obj with the assigned value ‘value1’.
- Step 4: Now, if you log obj to the console, you should see {property1: ‘value1’}.
Using bracket notation, you can add properties to objects dynamically, even with property names that might not be valid variable identifiers or are only available as strings at runtime.
#3 – By using Object.assign() method
The Object.assign() method in JavaScript allows you to copy values from one or more source objects to a target object. It’s a useful method for adding new key-value pairs or updating existing pairs in an object. Let’s understand this better with an example.
let obj1 = { a: 1, b: 2 };
let obj2 = { c: 3, d: 4 };
let result = Object.assign(obj1, obj2);
How it works
The Object.assign() method copies properties from one or more source objects (obj2 in our case) and adds them to a target object (obj1 in our case). The method returns the target object which now includes properties from the source object(s).
- Step 1: We create two objects, obj1 with properties a and b, and obj2 with properties c and d.
- Step 2: We use Object.assign() and pass obj1 as the target object and obj2 as the source object.
- Step 3: Object.assign() iterates over the properties in the source object(s) and copies them to the target object. If a property already exists on the target object, it is updated with the new value.
- Step 4: The method returns the target object with the added or updated properties. In our case, the result object will now be { a: 1, b: 2, c: 3, d: 4 }.
Related:
- How to Use printf in JavaScript
- How to Use Curly Braces in JavaScript
- How to Use CamelCase in JavaScript
In conclusion, adding key-value pairs to objects in JavaScript is a straightforward process that can greatly enhance data management. It’s as simple as using dot notation or bracket notation, and it opens up vast possibilities for dynamic data interaction.
Remember, while working with objects in JavaScript, it’s important to keep track of your keys and values. Consistency and practice in manipulating objects will make your coding journey smoother and more efficient.