How to Use printf in JavaScript
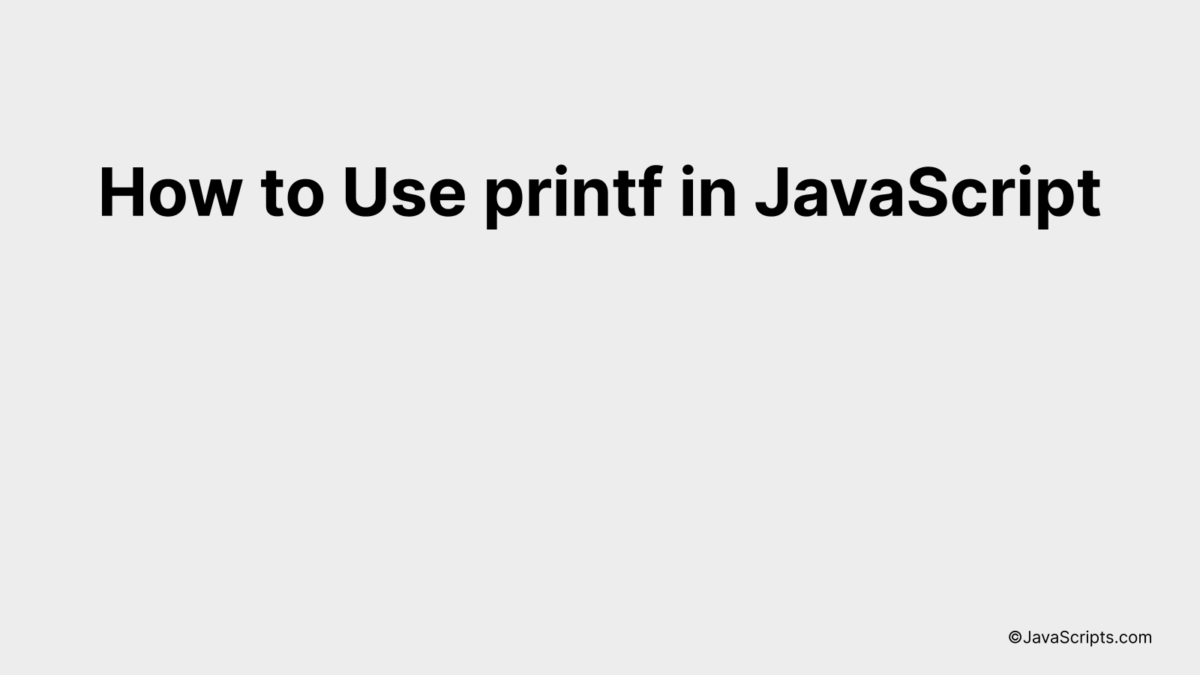
Ever needed a simple, efficient way to format your console output? JavaScript might just have the perfect solution for you! The printf method is a handy tool that could enhance your coding experience.
Don’t worry if you’re unfamiliar with printf! You and I will go on a discovery tour through its uses, how to implement it, and when it’s best to utilize this tool. By the end, we’ll have you formatting like a pro!
In this post, we will be looking at the following 3 ways to use printf in JavaScript:
- By using console.log() method
- By using process.stdout.write() in Node.js
- By using alert() method in browser-based JavaScript
Let’s explore each…
#1 – By using console.log() method
You can utilize JavaScript’s console.log()
function, similar to C’s printf()
function, to output formatted text in your console. You can insert variables into your output string using placeholders, like ‘%s’ for strings, and ‘%d’ for numbers. The provided variables will replace these placeholders in the order they are given. Let’s delve into an example to understand it better.
let name = 'John';
let age = 30;
console.log('Hello, my name is %s and I am %d years old.', name, age);
How it works
The console.log()
function in JavaScript takes a string as input and can also take an any number of additional arguments. These arguments can be variables that are then inserted into the string at the corresponding placeholders.
- First, we define two variables, a string
name
and a numberage
. - Next, we call the
console.log()
function. The first argument is a string with two placeholders, ‘%s’ and ‘%d’. - ‘%s’ is a placeholder for a string variable, which in this case is
name
. - ‘%d’ is a placeholder for a number variable, which in this case is
age
. - The
console.log()
function replaces the placeholders with the provided variables in the order they are given, resulting in the output: ‘Hello, my name is John and I am 30 years old.’
#2 – By using process.stdout.write() in Node.js
In Node.js, the process.stdout.write() function can be used like printf in other languages, allowing you to output formatted strings directly to the standard output (stdout). Here is an example:
process.stdout.write(Hello, ${name}n);
How it works
The process.stdout.write() function in Node.js works by sending the provided string directly to the standard output (stdout), which is typically the console. This approach is analogous to the printf function in languages like C.
- Firstly, process.stdout is a writable stream that represents the standard output for the Node.js process. It is a global object, hence, it’s available anywhere in the code without the need for any imports.
- Secondly, write() is a function of all writable streams in Node.js. It writes some data into the stream and handles backpressure (resource management when data comes in faster than it can be written).
- Lastly, the string provided to process.stdout.write() can contain variables, represented within ${}. These variables will be replaced by their respective values when the string is written to the standard output.
#3 – By using alert() method in browser-based JavaScript
In JavaScript, we can simulate the printf functionality using the alert() method to display formatted strings. We’ll show you an example of interpolating variables into a string using template literals.
let name = "John";
let age = 21;
alert(Hello ${name}, you are ${age} years old!);
How it works
The alert() function in JavaScript is used to show an alert box with a specified message and an OK button. In this case, we’re using template literals (denoted by backticks) to interpolate variables into a string.
- The variables “name” and “age” are declared and initialized with values “John” and 21 respectively.
- A string is created using the template literal syntax. This allows for the inclusion of embedded expressions, which are wrapped in “${}” brackets.
- The expressions inside the “${}” brackets are automatically converted to strings and are concatenated with the rest of the string literal.
- The result is then passed to the alert() function, which displays the final string in an alert box.
Related:
- How to Use Curly Braces in JavaScript
- How to Use CamelCase in JavaScript
- How to Translate Text with JavaScript
In conclusion, JavaScript doesn’t natively support printf, a function popular in languages like C. However, you can achieve similar functionality using various methods like ‘console.log’, string interpolation, or ‘util.format’ if you’re using Node.js.
Adapting these methods can help you format strings in a way that resembles printf. By practicing and experimenting, you can master these methods and streamline your JavaScript coding. Remember, consistent practice leads to perfect execution.