How to Check if a String Begins with in JavaScript
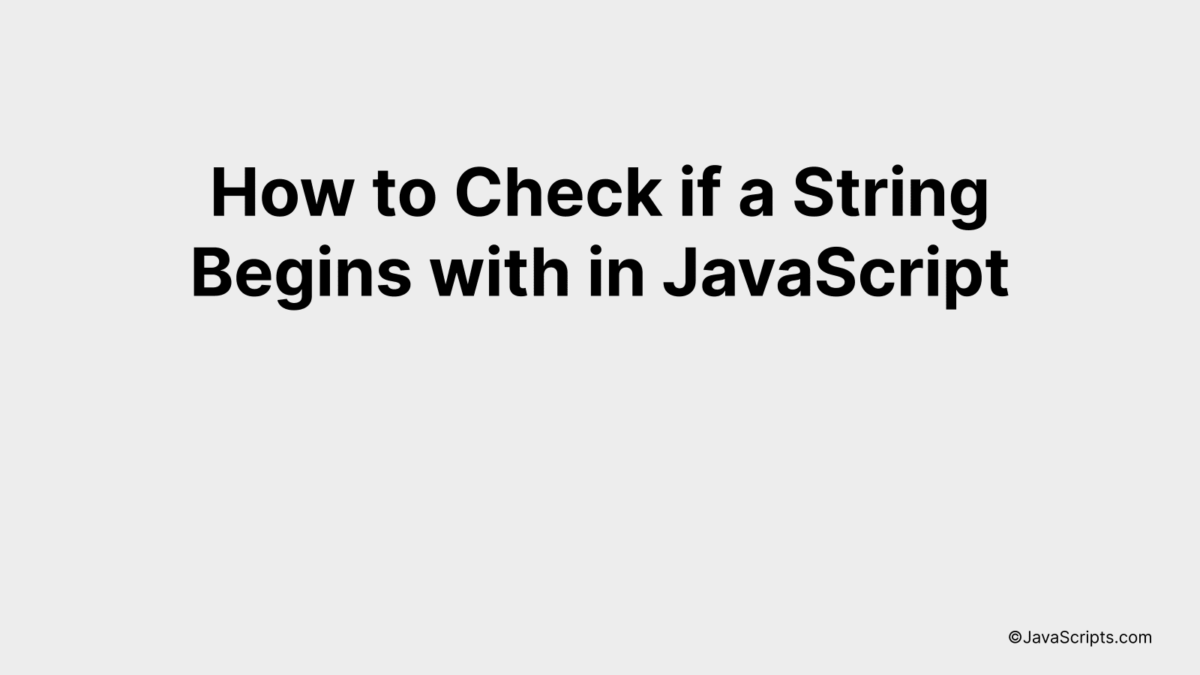
As a JavaScript enthusiast, you might have come across situations where you need to check if a particular string begins with a specific set of characters or a substring. This is a common scenario, especially when dealing with inputs or text data in web development.
Don’t worry, it’s a piece of cake with JavaScript! You and I will explore a simple yet powerful built-in method that JavaScript offers to tackle this task. Together, we’ll make your coding journey smoother and more enjoyable.
In this post, we will be looking at the following 3 ways to check if a string begins with in JavaScript:
- By using the startsWith() method
- By using the indexOf() method
- By using the charAt() method
Let’s explore each…
#1 – By using the startsWith() method
The JavaScript startsWith() method is used to check if a string starts with specified characters. It returns a boolean value – true if the string starts with specified characters, otherwise it returns false. We’ll illustrate this with an example.
let str = "Hello, World!";
let result = str.startsWith("Hello");
How it works
The startsWith() method works by comparing the initial characters of the string with the argument passed into the method. If the string starts with the specified characters, it returns true, otherwise it returns false.
- First, we have a string
str
with the value “Hello, World!”. - Next, we call the startsWith() method on the string
str
and pass “Hello” as the argument. This means we’re checking ifstr
starts with “Hello”. - The
startsWith()
method compares the starting part ofstr
with the string “Hello”. - Since “Hello, World!” does indeed start with “Hello”, the method returns true. The result (true in this case) is then stored in the variable
result
.
#2 – By using the indexOf() method
The indexOf() method in JavaScript returns the position of the first occurrence of a specified value in a string. This position is an integer between 0 and string.length-1. If the value to search for never occurs, it returns -1. Therefore, we can use this method to check if a string begins with a specified value by checking if the returned position is 0. Let’s understand this by using an example.
let str = 'Hello, World!';
let checkStr = 'Hello';
if(str.indexOf(checkStr) === 0) {
console.log('The string begins with Hello');
} else {
console.log('The string does not begin with Hello');
}
How it works
The indexOf() method checks if the specific substring (‘Hello’ in this case) exists at the beginning of the main string (‘Hello, World!’). If it does, indexOf() returns 0 (since string indices start at 0), and we print ‘The string begins with Hello’. If it doesn’t, indexOf() returns any index greater than 0 or -1, leading to the output ‘The string does not begin with Hello’.
Here are the step-by-step workings of the code:
- Step 1: Define the string we want to check in a variable – ‘Hello, World!’ in this case.
- Step 2: Define the substring we want to check for at the beginning of the string in another variable – ‘Hello’ in this case.
- Step 3: Use the indexOf() method on the main string with the substring as an argument. This method will return the index at which the substring starts in the main string.
- Step 4: Check if the returned index is 0 since the first character of a string is at index 0. If the substring is at the beginning of the string, indexOf() will return 0.
- Step 5: If the index is 0, print ‘The string begins with Hello’. If it’s not, print ‘The string does not begin with Hello’.
#3 – By using the charAt() method
The charAt() method in JavaScript returns the character at a specific index in a string. We can use this to check if a string begins with a certain character by comparing the character at the first index (index 0) with the desired character. For example, if we want to check if a string begins with ‘A’, we can use the charAt(0) method and compare its return value with ‘A’.
const str = "Apple";
const charToCheck = "A";
const doesStartWithChar = str.charAt(0) === charToCheck;
console.log(doesStartWithChar);
How it works
The JavaScript charAt() method retrieves the character at a specified index from a string. In our case, we want to check if the string starts with a specific character, so we use the 0th index. If the character at the 0th index matches our desired character, the output is true; otherwise, it is false.
- The ‘str’ variable is assigned the value “Apple”. This is the string we’re checking.
- The ‘charToCheck’ variable is assigned the value “A”. This is the character we’re looking for at the beginning of the string.
- Using the charAt() method on ‘str’, we check the character at the 0th index. The triple equals operator (===) is used to check if this character is the same as ‘charToCheck’. The result of this comparison is assigned to the ‘doesStartWithChar’ variable.
- Finally, the result is printed to the console. If ‘str’ started with ‘A’, the output will be ‘true’; otherwise, it will be ‘false’.
Related:
- How to Add Key-Value Pairs to Objects in JavaScript
- How to Use printf in JavaScript
- How to Use Curly Braces in JavaScript
In conclusion, understanding how to check if a string begins with a specific sequence in JavaScript is an essential skill for every JavaScript developer. It allows more flexibility and control over data manipulation, contributing significantly to the development of efficient and robust applications.
The ‘startsWith’ method is quite simple and straightforward to use, providing accurate results in a jiffy. By leveraging this feature, you can seamlessly implement condition checks based on string beginnings to make your code more dynamic and responsive.